Introduction
This tip discusses C# WPF application with Windows shutdown timer, fast shutdown, normal shutdown, hibernate, sleep, lock, log off, restart options.
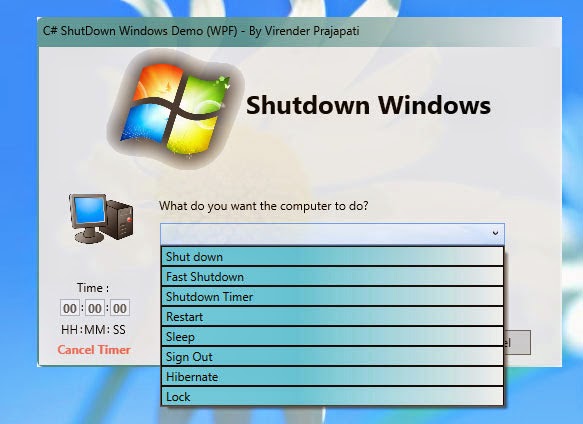
Using the Code
Add these namespaces:
using System.Runtime.InteropServices;
using System.Threading.Tasks;
Creating a Countdown timer.
Intialization:
public static int timeLeft;
public static int hour;
public static int min;
public static int sec;
System.Windows.Forms.Timer timer = new System.Windows.Forms.Timer();
timer.Interval = 1000; timer.Tick += new EventHandler(timer_Tick);
Get Values of time variable and start timer:
timeLeft = hour * 3600 + min * 60 + sec; timer.Start();
Countdown Timer with Each timer tick and shutdown when time is over:
private void timer_Tick(object sender, EventArgs e)
{
if(timeLeft>0) {
timeLeft = timeLeft - 1; hour = timeLeft / 3600; min = (timeLeft - (hour*3600)) / 60; sec = timeLeft - (hour * 3600) - (min * 60); hh.Text = hour.ToString(); mm.Text = min.ToString(); ss.Text = sec.ToString(); }
else
{
timer.Stop(); Process.Start("shutdown", "/s /t 0"); }
}
To cancel timer use:
timer.Stop( );
To pause timer use:
timer.Pause( );
Other Windows Shutdown Options
1. Normal Shut down
Process.Start("shutdown", "/s /t 0");
2. Fast Shut down Forcibly
Process.Start("shutdown", "/s /f /t 0");
3. Restart
Process.Start("shutdown", "/r /t 0");
4. Signout and Lock
Need to import already defined "user32
" library and add these extern methods:
[DllImport("user32")]
public static extern void LockWorkStation(); [DllImport("user32")]
public static extern bool ExitWindowsEx(uint Flag, uint Reason);
SignOut
ExitWindowsEx(0, 0);
Lock
LockWorkStation();
5. Hibernate and Sleep
Need to import already defined "Powerprof
" library and add this extern method:
[DllImport("Powrprof.dll", CharSet = CharSet.Auto, ExactSpelling = true)]
public static extern bool SetSuspendState(bool hiberate, bool forceCritical, bool disableWakeEvent);
Hibernate
SetSuspendState(true, true, true);
Sleep
SetSuspendState(false, true, true);