Introduction
Here is the code for Add, Update, Delete in Angular.
Using the Code
Starting with the code, first we do need to include Angular file in our project.
Then make a simple table for Employee ID, Name, designation, as I have included the code for the same here, then write the Angular code in script
tag.
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="../jquery/jquery/jquery-1.11.2.js"></script>
<script src="angular.min.js"></script>
</head>
<body ng-app="" ng-controller="empcontroller">
<br />
<br />
<table>
<tbody> <!--
<tr>
<td>Enter ID: </td><td>
<input type="text" ng-model="id"/></td>
</tr>
<tr>
<td>Enter Name: </td><td>
<input type="text" ng-model="name"/></td>
</tr>
<tr>
<td>Enter Designation: </td><td>
<input type="text" ng-model="desg"/></td>
</tr>
<tr>
<td><input type="button"
ng-click="btnclk()" value="Save"/></td>
<td><input type="button"
ng-click="btnupd(id,name,desg)" value="Update"/></td>
</tr>
</tbody>
</table>
<table> <!--
<thead>
<tr>
<td>ID</td>
<td>Name</td>
<td>Designation</td>
</tr>
</thead>
<tbody ng-repeat="emp in emparr">
<tr>
<td ng-bind="emp.arr_id"></td>
<td ng-bind="emp.arr_name"></td>
<td ng-bind="emp.arr_desg"></td>
<td><input type="button"
ng-click="edit(emp,emparr.indexOf(emp))" value="Edit"/></td>
<td><input type="button"
ng-click="del(emparr.indexOf(emp))" value="Delete"/></td>
</tr>
</tbody>
</table>
Now in the first table, we have created the textbox
to enter data and bind the HTML control with ng-model
and get the value of that control.
In the second table, we have used ng-repeat
that will clone HTML elements one for each item in collection (in an array).
We have created a button (Save) that will have a click event to call the function associated with it to save the data in the second table. On clicking the Edit button the data will be set in the textboxes in the first table to be edited, button (Update) that will update the existing data in the table and delete button that will delete the selected data.
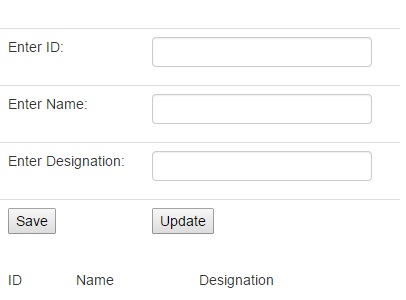
This is the first table to input data.
As I have also designed the code to validate that the user doesn't enter blank data in the textboxes, now enter some data and click Save button.
Now you can see that user is not allowed to enter blank details.
Saving Data
Now enter data and click save button, clicking it will invoke btnclk()
method to save the data. To enter the data, we are using push
method to add an array element in ng-repeate=emparr
. The textbox
es are binded by ng-model
so that their values will be used to save data in the array element.
$scope.btnclk = function () {
if (!$scope.id)
{
alert("Enter ID");
}
else if (!$scope.name)
{
alert("Enter Name");
}
else if (!$scope.desg) {
alert("Enter Designation");
}
else {
$scope.emparr.push({ 'arr_id': $scope.id,
'arr_name': $scope.name, 'arr_desg': $scope.desg });
$scope.id = '';
$scope.name = '';
$scope.desg = '';
}
};

Editing Data
Select any of the data you want to edit by clicking edit button.
Clicking the button will pass the (emp
) model along with the assigned index number of the array element by indexOf
method. By the data in the model, we are going to assign the particular data to the textbox
es of ID, Name, Designation and the index number received in the data will be saved in a variable (key). This variable will be used to recognize to which array element we have to update the data. So in this manner, whenever you click any element of array will update the value of key variable as well by current index number of the array.
var key;
$scope.edit = function (emp, indx) {
key = indx;
$scope.id = emp.arr_id;
$scope.name = emp.arr_name;
$scope.desg = emp.arr_desg;
};

Updating Data
Now edit the data and after editing, click update button.
Clicking the button will pass the ng-model
of ID, Name, designation textboxes values as parameter, and that data will be used to update our array element. Now here, we will use the value of variable (key) saved to identify at which element we have to update the array.
$scope.btnupd = function (id, name, desg) {
$scope.emparr[key].arr_id = id;
$scope.emparr[key].arr_name = name;
$scope.emparr[key].arr_desg = desg;
$scope.id = '';
$scope.name = '';
$scope.desg = '';
};

Deleting Data
Now select the data which you want to delete.
Clicking the delete button will pass the index number of the array by indexOf
method and then we are using the Splice
method to remove array element. The first argument of splice
method defines which array element to remove and the second defines how many to remove.
$scope.del = function (id) {
$scope.emparr.splice(id, 1);
};
