Introduction
I found many beginners who work on ASP.NET WebGrid
are facing problems to align header and put sorting icon in header. This little part is for them. We can easily do it using jQuery and CSS in client side.
Using the Code
In this section, we are going to add some CSS and jQuery in our application.
CSS
First of all, we need three images for both side sort order, ascending order and descending order. Or we can use Icon
class instead of images. In CSS, we have to add three classes for sorting like this:
.ascendingorder {background: url("../images/sort_asc.png") no-repeat scroll right center;}
.descendingorder {background: url("../images/sort_desc.png") no-repeat scroll right center;}
.bothorder {background: url("../images/sort_both.png") no-repeat scroll right center;}
WebgridSample.cshtml
We need to add two hidden fields for identifying the clicked header and its sorting direction.
<div id="WebGrid">
@{
WebGrid grid = new WebGrid(
rowsPerPage: Model.RowsPerPage,
ajaxUpdateContainerId: "WebGrid",
ajaxUpdateCallback: "ChkAddClass"); // It will get called after the server call is completed.
// Your Code
tableStyle: "ASPGrid",
@Html.Hidden("dir", grid.SortDirection)
@Html.Hidden("col", grid.SortColumn)
}
Then, we need to add some jQuery at the bottom of the page. Because it is good practice. It will load the image/icon in every column header when the page is loaded.
<script type="text/javascript">
$(document).ready(function () {
$(".ASPGrid tr th").addClass("bothorder");
})
</script>
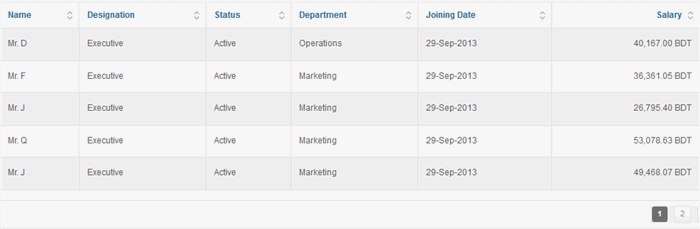
For getting ascending and descending order icon according to which column header is clicked, we need to add some jQuery in ajaxUpdateCallback
function.
<script>
function ChkAddClass() {
$(".ASPGrid tr th").addClass("bothorder");
var dir = $('#dir').val();
var col = $('#col').val();
var clickedheader = $('th a[href*=' + col + ']');
var countTh = document.getElementsByTagName('th').length;
for (var i = 1; i <= countTh; i++) {
var txtTh = $('.ASPGrid tr th:nth-child(' + i + ')').text();
if (txtTh.trim() == clickedheader.text() && dir == 'Ascending')
{
$('.ASPGrid tr th:nth-child(' + i + ')').removeClass("bothorder");
$('.ASPGrid tr th:nth-child(' + i + ')').addClass("ascendingorder");
}
else if (txtTh.trim() == clickedheader.text() && dir == 'Descending')
{
$('.ASPGrid tr th:nth-child(' + i + ')').removeClass("bothorder");
$('.ASPGrid tr th:nth-child(' + i + ')').addClass("descendingorder");
}
}
}
</script>
That's it. We will get this:


Now the last part for header alignment.
CSS
.ASPGrid tr th:nth-child(1)
{
text-align: left;
}
.ASPGrid tr th:nth-child(7)
{
text-align: right;
}