Introduction
It is easy to implement Copy Paste operations using C# code.
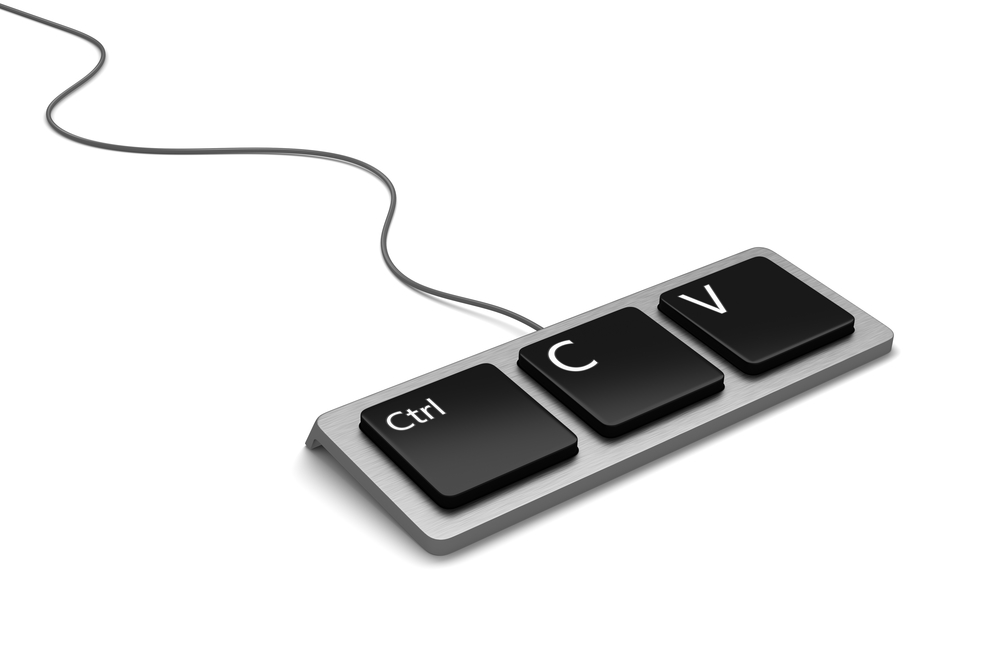
First, you need to make the class that you want to put into clipboard- serializable.
[Serializable]
public class ClipBoardTestCase
{
public ClipBoardTestCase(List<TestCase> testCases, ClipBoardCommand clipBoardCommand)
{
this.TestCases = testCases;
this.ClipBoardCommand = clipBoardCommand;
}
public List<TestCase> TestCases { get; set; }
public ClipBoardCommand ClipBoardCommand { get; set; }
}
ClipBoardCommand
is a simple enum
with three values: Copy
, Cut
, None
. We are going to use it later in the paste operation to determine if the clip should be removed from the clipboard.
Also, make sure that your class is serializable, add the [Serializable]
attribute to it. If you are working with system objects, be sure that all of them can be serialized. I have noticed that some of the core TFS objects are not. If that is the case, just add a [NonSerialized]
attribute to them.
Serialization Tester
Also, you can use the following method to test if the properties and variables of your class can be serialized.
public static bool IsSerializable(object obj)
{
MemoryStream mem = new MemoryStream();
BinaryFormatter bin = new BinaryFormatter();
try
{
bin.Serialize(mem, obj);
return true;
}
catch (Exception ex)
{
MessageBox.Show("Your object cannot be serialized." +
" The reason is: " + ex.ToString());
return false;
}
}
Implement Copy Paste C# Serialization Manager
I have created a reusable generic class for the basic clipboard actions; you can use it directly.
public class ClipBoardManager<T> where T : class
{
public static T GetFromClipboard()
{
T retrievedObj = null;
IDataObject dataObj = Clipboard.GetDataObject();
string format = typeof(T).FullName;
if (dataObj.GetDataPresent(format))
{
retrievedObj = dataObj.GetData(format) as T;
}
return retrievedObj;
}
public static void CopyToClipboard(T objectToCopy)
{
DataFormats.Format format = DataFormats.GetFormat(typeof(T).FullName);
IDataObject dataObj = new DataObject();
dataObj.SetData(format.Name, false, objectToCopy);
Clipboard.SetDataObject(dataObj, false);
}
public static void Clear()
{
Clipboard.Clear();
}
}
The usage is simple. Just initialize your object and pass it to the manager's method.
ClipBoardCommand clipBoardCommand = isCopy ? ClipBoardCommand.Copy : ClipBoardCommand.Cut;
ClipBoardTestCase clipBoardTestCase = new ClipBoardTestCase(testCases, clipBoardCommand);
ClipBoardManager<ClipBoardTestCase>.CopyToClipboard(clipBoardTestCase);
When you are performing cut operations, you need to remove the last clip from the clipboard or clear it all. So you can use the ClipBoardCommand enum
to do it.
Task t = Task.Factory.StartNew(() =>
{
TestSuiteManager.PasteTestCasesToSuite(suiteToPasteIn.Id, clipBoardTestCase);
});
t.ContinueWith(antecedent =>
{
if (clipBoardTestCase.ClipBoardCommand.Equals(ClipBoardCommand.Cut))
{
System.Windows.Forms.Clipboard.Clear();
}
}, TaskScheduler.FromCurrentSynchronizationContext());
If you need to add more logic to your clipboard manager, you can visit the official MSDN documentation here.
So Far in the C# Series
- Implement Copy Paste C# Code
- MSBuild TCP IP Logger C# Code
- Windows Registry Read Write C# Code
- Change .config File at Runtime C# Code
- Generic Properties Validator C# Code
- Reduced AutoMapper- Auto-Map Objects 180% Faster
- 7 New Cool Features in C# 6.0
- Types Of Code Coverage- Examples In C#
- MSTest Rerun Failed Tests Through MSTest.exe Wrapper Application
- Hints For Arranging Usings in Visual Studio Efficiently
- 19 Must-Know Visual Studio Keyboard Shortcuts – Part 1
- 19 Must-Know Visual Studio Keyboard Shortcuts – Part 2
- Specify Assembly References Based On Build Configuration in Visual Studio
- Top 15 Underutilized Features of .NET
- Top 15 Underutilized Features of .NET Part 2
- Neat Tricks for Effortlessly Format Currency in C#
- Assert DateTime the Right Way MSTest NUnit C# Code
- Which Works Faster- Null Coalescing Operator or GetValueOrDefault or Conditional Operator
- Specification-based Test Design Techniques for Enhancing Unit Tests
- Get Property Names Using Lambda Expressions in C#
- Top 9 Windows Event Log Tips Using C#
If you enjoy my publications, feel free to SUBSCRIBE.
Also, hit these share buttons. Thank you!
Source Code
The post- Implement Copy Paste C# VB .NET Application appeared first on Automate The Planet.
All images are purchased from DepositPhotos.com and cannot be downloaded and used for free.
License Agreement