Introduction
This tip will help you understand the basic way of creating WCF services.
Background
I used to create web services but then I was once working on a migration application project where I was supposed to convert all web services to WCF services. I was new to it. I still am. But will little hands on experience. Let me share how I learned creating and consuming WCF services
Ok before that, my manager asked me a simple question “What do you know about message binding, contracts, and endpoints?” without which you cannot proceed with it.
I said “I don’t know technical definitions. What I know is you should know:
- Where the service is located
- How we can communicate or consume the service
- And what the parameters the service seeks are.”
Now that suffices completely. You can now map it with technical jargons.
I learnt these things from .NET interview videos.
I thought of a simple webpage with functionality of doing mathematical calculation to learn WCF.
I created new Projects from Visual Studio 2013 of type WCF application.
Using the Code
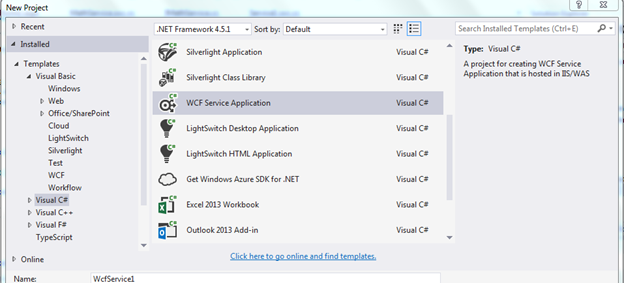
Now I created a class (svc file) which was supposed to be an interface and define the methods to be implemented later called IMathService.svc.
[ServiceContract]
public interface IMathService
{
[OperationContract]
Int32 Sum(Int32 a, Int32 b);
[OperationContract]
Int32 difference(Int32 a, Int32 b);
[OperationContract]
Int32 Product(Int32 a, Int32 b);
}
}
Now I declared a class which can actually implement the interface class for maths operation:
public class MathService : IMathService
{
public Int32 Sum(Int32 a, Int32 b)
{
return a+b;
}
public Int32 difference(Int32 a, Int32 b)
{
return a-b;
}
public Int32 Product(Int32 a, Int32 b)
{
return a * b;
}
}
}
Now my service is ready to use.
Let's see how we can consume this service. To do that, I created a Simple User Interface which holds UI controls to do maths calculation and display result.
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
num1 <asp:TextBox ID="txt1Num1" runat="server"></asp:TextBox>
num2 <asp:TextBox ID="txtNum2" runat="server"></asp:TextBox>
<asp:DropDownList ID="DropDownList1" runat="server">
<asp:ListItem>Add</asp:ListItem>
<asp:ListItem>sub</asp:ListItem>
<asp:ListItem>product</asp:ListItem>
</asp:DropDownList>
result <asp:TextBox ID="txtResult" runat="server"></asp:TextBox>
<asp:Button ID="btnDisplay" runat="server"
Text="Display" OnClick="btnDisplay_Click" />
</div>
</form>
</body>
</html>
Now the most important thing is to add the web reference to consume it.

Now on the button click event, I implemented the corresponding functionality:
using MathApp.ServiceReference1;
namespace MathApp
{
public partial class WebForm1 : System.Web.UI.Page
{
MathServiceClient client = new MathServiceClient();
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnDisplay_Click(object sender, EventArgs e)
{
if(DropDownList1.SelectedItem.Text=="Add")
{
var res = client.Sum(Int32.Parse(txt1Num1.Text), Int32.Parse(txtNum2.Text));
txtResult.Text=res.ToString();
}
else if (DropDownList1.SelectedItem.Text == "sub")
{
var res = client.difference(Int32.Parse(txt1Num1.Text), Int32.Parse(txtNum2.Text));
txtResult.Text = res.ToString();
}
else
{
var res = client.Product(Int32.Parse(txt1Num1.Text), Int32.Parse(txtNum2.Text));
txtResult.Text = res.ToString();
}
}
}
}
That’s it. You are now ready to test the WCF service. Run the solution.



Points of Interest
So that’s how I tried to make it very simple to learn how to create WCF services.
By the way, the technically endpoint, binding and contract details are available in the web.config as below:
<endpoint address="http://localhost:18647/MathService.svc"
binding="basicHttpBinding"
bindingConfiguration="BasicHttpBinding_IMathService"
contract="ServiceReference1.IMathService"
name="BasicHttpBinding_IMathService" />
I will post more on an advanced way of creating WCF service.