Introduction
If you are new to Angular JS, I suggest you read the Basics of AngularJS.
Now with the belief that you have read my previous article, let's begin.
Using the Code
We will explain that with a demo. Please see the below implementation.
<body ng-app ng-init="placesVisited=['Delhi','Kerala','Tamil Nadu',
'Banglore','Uttar Pradesh','Noida','Haryana','Thrissur'];">
<div>
<input type="text" ng-model="curPlace" class="inputText">
<ul>
<li ng-repeat="place in placesVisited | filter:curPlace">
<a ng-href="https://www.google.co.in/webhp?q={{place}}">{{place}} </a>
</li>
</ul>
</div>
<script src="angular.min.js"></script>
</body>
In this, as we discussed with the previous article, I have declared my ng-app
and ng-init
. In the initialization part, I have given the place names I have visited.
placesVisited=['Delhi','Kerala','Tamil Nadu','Banglore','Uttar Pradesh','Noida','Haryana','Thrissur'];
I have declared my model with the name curPlace
and after we are binding the initialized array elements to the li
tag using ng-repeat
directive. I have set the href as follows to make it meaningful, so that if you click a place name, it will redirect to the Google search.
<a ng-href="https://www.google.co.in/webhp?q={{place}}">{{place}} </a>
Here, the process is whenever you type any character in the model curPlace
, the filter will be triggered and it will bind the appropriate result to the li
. For a basic implementation, you do not need to do anything other than this. Angular JS makes a searching functionality much easier, right?
Include CSS If You Need
<style>
.inputText {
border: 1px solid #ccc;
border-radius: 10px;
background-color: #0ff;
box-shadow: 1px 1px 1px 1px #ccc;
width: 230px;
height: 30px;
}
ul {
list-style: none;
padding: 10px;
background-color: #CAEAF5;
border-radius: 10px;
border: 1px solid #ccc;
width: 210px;
}
li {
border: 1px solid #ccc;
border-right: none;
border-left: none;
padding: 2px;
text-align: center;
}
</style>
Complete HTML
<!DOCTYPE html>
<html>
<head>
<title>Angular animated search box - SibeeshPassion </title>
<style>
.inputText {
border: 1px solid #ccc;
border-radius: 10px;
background-color: #0ff;
box-shadow: 1px 1px 1px 1px #ccc;
width: 230px;
height: 30px;
}
ul {
list-style: none;
padding: 10px;
background-color: #CAEAF5;
border-radius: 10px;
border: 1px solid #ccc;
width: 210px;
}
li {
border: 1px solid #ccc;
border-right: none;
border-left: none;
padding: 2px;
text-align: center;
}
</style>
</head>
<body ng-app ng-init="placesVisited=['Delhi','Kerala','Tamil Nadu',
'Banglore','Uttar Pradesh','Noida','Haryana','Thrissur'];">
<div>
<input type="text" ng-model="curPlace" class="inputText">
<ul>
<li ng-repeat="place in placesVisited | filter:curPlace">
<a ng-href="https://www.google.co.in/webhp?q={{place}}">{{place}} </a>
</li>
</ul>
</div>
<script src="angular.min.js"></script>
</body>
</html>
Output
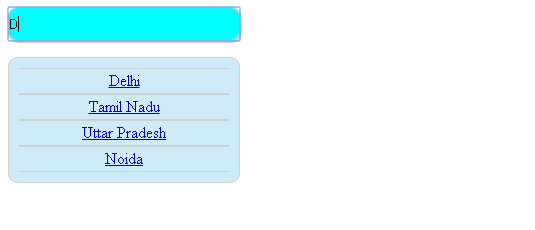
Conclusion
Now that is all for the day. I will come with another set of items in Angular JS soon? I hope you liked this article. Please give your valuable suggestions.