Sorting ASP.NET Dropdown list is a very common requirement for any of the web application development. To implement these features, sometimes developers used to iterate through each and every item and create a sorted list of element then reassign the same source to dropdownlist
or sort the element at source itself. But this thing can be done easily using LINQ. In this post, I am going describe how you can sort a ASP.NET DropDownList
based on either of DataTextField
or DataValueField
using LINQ and list of KeyValuePair
elements as DataSource
.
To start with the application, let’s consider you have the following List
of employee
data as datasource
of the DropDownList
.
List<KeyValuePair<int, string>> employees = new List<KeyValuePair<int, string>>
{ new KeyValuePair<int,string>(1,"Abhijit"),
new KeyValuePair<int,string>(2,"Rahul"),
new KeyValuePair<int,string>(3,"Kunal"),
new KeyValuePair<int,string>(4,"Atul"),
new KeyValuePair<int,string>(5,"Abhishek"),
};
In the employee
list collection, you have added KeyValuePair
for each element, where Key
is employee ID and Value
is the employee name. The most interesting part of using KeyValuePair
is that you can bind either of Key or Value with the DropDownList as per your requirement.
Now let’s bind the DropDownList
with the DataSource
.
private void BindDropDownList()
{
dropDownListEmployee.DataSource = this.employees;
dropDownListEmployee.DataTextField = "Value";
dropDownListEmployee.DataValueField = "Key";
dropDownListEmployee.DataBind();
}
If you call the BindDropDownList()
, you will get output like below:
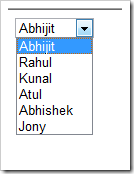
If you look at the code for BindDropDownList()
method, we have set the Value
(Name
) with the DataTextField
and Key
(ID
) with DataValueField
, where Value
and Key
are the Item
of KeyValuePair
.
Now, if you want to sort the List Item based on the Name
of employee
, you have used the below code:
private void BindDropDownList()
{
dropDownListEmployee.DataSource = this.employees.OrderBy(item => item.Value);
dropDownListEmployee.DataTextField = "Value";
dropDownListEmployee.DataValueField = "Key";
dropDownListEmployee.DataBind();
}
In the above code, we are applying sort based on Value
of KeyValuePair
(Name
). Below is the sample output for the same.
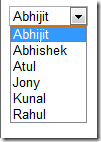
This is really interesting, where you have just made a single line of change and you got the sorted elements in dropdown list. Here is the most important part. In the above code, we have sorted the element based on the DataTextField
(Name
) now if you want to sort the list based on DataValueField
(ID
), you just need to use sort based on the Key
field. Below is the sample code snippet for the same.
private void BindDropDownList()
{
dropDownListEmployee.DataSource = this.employees.OrderBy(item => item.Key);
dropDownListEmployee.DataTextField = "Value";
dropDownListEmployee.DataValueField = "Key";<
dropDownListEmployee.DataBind();
}
After running the application, you will find the dropdown list items as below:
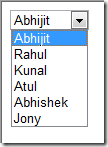
Similarly, you can also do the Descending
order using the below code snippet:
private void BindDropDownList()
{
dropDownListEmployee.DataSource = this.employees.OrderByDescending(item => item.Key);
dropDownListEmployee.DataTextField = "Value";
dropDownListEmployee.DataValueField = "Key";
dropDownListEmployee.DataBind();
}
Below is complete code coverage for the above demonstration:
using System;
using System.Collections.Generic;
using System.Linq
public partial class _Default : System.Web.UI.Page
{
List<KeyValuePair<int, string>> employees = new List<KeyValuePair<int, string>>
{
new KeyValuePair<int,string>(1,"Abhijit"),
new KeyValuePair<int,string>(2,"Rahul"),
new KeyValuePair<int,string>(3,"Kunal"),
new KeyValuePair<int,string>(4,"Atul"),
new KeyValuePair<int,string>(5,"Abhishek"),
};
protected void Page_Load(object sender, EventArgs e)
{
this.BindDropDownList();
this.employees.Add(new KeyValuePair<int, string>(6, "Jony"));
this.BindDropDownList();
}
private void BindDropDownList()
{
dropDownListEmployee.DataSource = this.employees.OrderBy(item => item.Key);
dropDownListEmployee.DataTextField = "Value";
dropDownListEmployee.DataValueField = "Key";
dropDownListEmployee.DataBind();
}
}
ASPX Page code snippet:
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:DropDownList ID="dropDownListEmployee" runat="server">
</asp:DropDownList>
</div>
</form>
<p></body>
</html>
Summary
In this blog post, I have explained how to bind a dropdown with List Of KeyValuePair
elements and sort a DropDownList
items based in the DataTextField
or DataValueField
using LINQ.
Hope this will help you !
Thanks !
AJ
Filed under: .NET 4.0, ASP.NET, ASP.NET 4.0, General, Tips and Tricks
