Authentication and Authorization are perhaps the most prominent things in web-based application development nowadays. As developers, we always need to ensure at each instance whether we are showing up the authorized content to the user.
Traditionally in ASP.NET, we achieve these concepts by isolating critical modules from the rest of the application, i.e., by segregating ASPX pages in a folder under the control of a custom web.config file that redirects not-authenticated and unauthorized users to a custom login page.
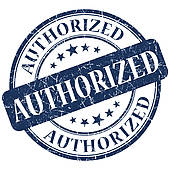
Now ASP.NET MVC alleviates the pain in attaining the role based security just by a simple yet powerful attribute known as Authorize. Let’s dive deep into it but if you are new to ASP.NET MVC, it’s recommended to review a comprehensive list of ASP.NET MVC Interview Questions for experienced and beginners available here.
Authorize Attribute in ASP.NET MVC
In default, all the Controllers and Action methods are accessible by both Anonymous and Authenticated users.
All the public
methods inside the Controllers can be easily accessed if one knows the method name and the
route pattern. Oops, that’s not a security breach, Wait!
Note: If you want to understand or learn all about Controllers and Action Methods in ASP.NET MVC, please follow here.
So how to sway and protect the Controllers and Methods of ASP.NET MVC? There comes our attribute called Authorize
into the play. Just by preceding this piece of word before any controllers or its action methods,
protect them from unauthorized access:
[Authorize] public ActionResult About()
{
ViewBag.Message = "Web Development Help!";
return View();
}
In the above snippet, we have decorated the action method About
with [Authorize]
attribute. So if any anonymous user, try routing to the above method, then he will be navigated to Login page.
Let Us Dirty Our Hands
- Open up Visual Studio 2012 and create an ASP.NET MVC application by choosing the Project template as Internet.

- We have
HomeController
and three Action Methods in it. If we launch of application now, one can access all the three methods without any restriction.



- For instance, let’s consider that Anonymous users should be prevented from accessing
About
method. If he tries to access, then MVC should navigate him to Login page. As discussed before, we can just decorate the About
method with [Authorize]
attribute. Note: While navigating user to Login page for authentication, note that the Re-Direct Url is been handled automatically for us.

- Wala! That works with much ease. So what if we need to protect all the three methods from anonymous user. Yes, preceding it before necessary Controller does that with charm.

This means that Authorize
attribute is inheritable, we can add it to a base controller class and thereby it ensure that any methods of any derived controllers are subject to authentication.
We can make certain Action
methods alone to anonymously access by preceding it with the attribute [AllowAnonymous]
.
[AllowAnonymous] public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
So here in the above snippet, the Contact
method is decorated with AllowAnonymous
attribute so that it can be accessed without any Login authentication.
Handling Authorization
ASP.NET MVC newbies often get confused with the Authorize
attribute’s name because it triggers the Authentication process but the name proclaim as Authorize
. It’s actually not a mislead, let us dive into Authorize
parameters to clarify this.
Additionally, there are two parameters that supports Authorize
attribute in restricting the execution of the action method only to certain user names and/or users with a given role.
[Authorize(Roles="admin",
Users="Imghani, Ren")] public ActionResult About()
{
ViewBag.Message = "Web Development Help!";
return View();
}
So while logging, it checks whether the user is Imghani or Ren and holds the Admin role or not. If not, then it redirects the user to the Login Url.
HTTP 401 or 403 (Custom Attribute)
Authorize
attribute doesn’t provide a clear cut HTTP status in return if the process gets failed. Here the reason could be either of two ways, mainly, i.e.:
- Authentication Failure
- Authorization Failure
For both the instances, Authorize
just returns the HTTP code as 401 which is generic but it is a tedious task for a developer to debug the exact reason behind that. To overcome this, MVC provides us the facility to override the Authorize
attribute.
public class Error401or403 : AuthorizeAttribute
{
public Error401or403()
{ }
Public override void OnAuthorization(AuthorizationContext filterContext)
{
base.OnAuthorization(filterContext);
CheckIfUserIsAuthenticated(filterContext);
}
}
Hence, by overriding the method OnAuthorization
and if we handle some extra logic like getting back the response from Authorize
, we gain complete control.
If the response is 401, instead of navigating the user again to the Login page (it works as default), we can create a custom beautiful UnAuthorized
error page and can navigate the user accordingly.
[Error401or403(Roles="admin", Users="Imghani")] public ActionResult Index()
{
….
}
The custom attribute doesn’t change the basic functionality. Rather it helps us to gain some control over the process. It returns a ViewResult
object, so that we can easily navigate it to our custom error page.
Got something to say!
Keep learning always, hope you have enjoyed reading it. We do have lot of awesome articles on ASP.NET MVC as well as free Online Tests and MCSD Online Practice Exams, please check out this website here.
Thanks, happy coding!
Top 10 Interview Questions and Answers Series
CodeProject
The post Insight of ASP.NET MVC’s Authorize attribute appeared first on Web Development Tutorial.