I started playing around with Azure DocumentDB
, and I am pretty excited about it. The simplicity is just crazy. Most of the applications out there need CRUD operations. So I thought I will try to compile them in one post for quick reference.
- To begin with, you need to create a
DocumentDB
database account. The below link walks through that:
- Create
DocumentDB
collections, which will hold our documents.
- Add documents into the collections. The following link explains how to add, view documents using the document explorer, we will see how to do them using the sdk.
- Get the
DocumentDB
client library package to start coding against Azure DocumentDB
.
Install-Package Microsoft.Azure.DocumentDB
- Create a
DocumentClient
, from which we will execute all our CRUD operations.
string uri= ConfigurationManager.AppSettings["URI"];
string primaryKey= ConfigurationManager.AppSettings["Primarykey"];
Uri endpointUri = new Uri(uri);
var client = new DocumentClient(endpointUri,primaryKey);
You can find the DocumentDB
URI and primary key from the Azure portal.
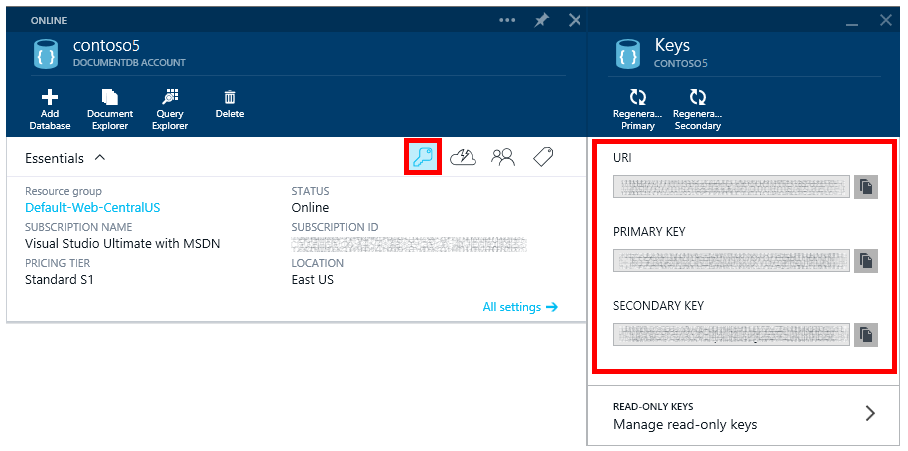
Once we have a DocumentClient
, we are ready to work with collections. To work with collections, we need to get the collection link, the easiest way to find the selflink for the collection is to use a tool called DocumentDB Studio.

Copy the selflink as part of your configuration.
As application developers, we work with entities and objects and their json serialized formats, Azure DocumentDB is designed from the ground up to natively support JSON and JavaScript directly inside the database engine, which makes application development much more agile. You can download the simple console application here which covers all the basic CRUD operations on Azure DocumentDB
. Also for quick reference, below is the code.
public class Employee
{
[JsonProperty(PropertyName = "id")]
public String Id { get; set; }
[JsonProperty(PropertyName = "name")]
public String Name { get; set; }
[JsonProperty(PropertyName = "age")]
public int Age { get; set; }
}
static void Main(string[] args)
{
string uri = ConfigurationManager.AppSettings["URI"];
string primaryKey = ConfigurationManager.AppSettings["Primarykey"];
Uri endpointUri = new Uri(uri);
var client = new DocumentClient(endpointUri, primaryKey);
CreateDocument(client);
ReadDocument(client);
UpdateDocument(client);
DeleteDocument(client);
}
private static void DeleteDocument(DocumentClient client)
{
var employeeToDelete =
client.CreateDocumentQuery<Employee>(ConfigurationManager.AppSettings["CollectionLink"])
.Where(e => e.Name == "John Doe")
.AsEnumerable()
.First();
Document doc = GetDocument(client, employeeToDelete.Id);
Task deleteEmployee = client.DeleteDocumentAsync(doc.SelfLink);
Task.WaitAll(deleteEmployee);
}
private static void UpdateDocument(DocumentClient client)
{
var employeeToUpdate =
client.CreateDocumentQuery<Employee>(ConfigurationManager.AppSettings["CollectionLink"])
.Where(e => e.Name == "John Doe")
.AsEnumerable()
.First();
Document doc = GetDocument(client, employeeToUpdate.Id);
Employee employeUpdated = employeeToUpdate;
employeUpdated.Age = employeeToUpdate.Age + 1;
Task updateEmployee = client.ReplaceDocumentAsync(doc.SelfLink,
employeUpdated);
Task.WaitAll(updateEmployee);
}
private static Document GetDocument(DocumentClient client, string id)
{
return client.CreateDocumentQuery(ConfigurationManager.AppSettings["CollectionLink"])
.Where(e => e.Id == id)
.AsEnumerable()
.First();
}
private static void ReadDocument(DocumentClient client)
{
var employees = client.CreateDocumentQuery<Employee>
(ConfigurationManager.AppSettings["CollectionLink"]).AsEnumerable();
foreach (var employee in employees)
{
Console.WriteLine(employee.Id);
Console.WriteLine(employee.Name);
Console.WriteLine(employee.Age);
Console.WriteLine("----------------------------------");
}
var singleEmployee =
client.CreateDocumentQuery<Employee>
(ConfigurationManager.AppSettings["CollectionLink"])
.Where(e => e.Name == "John Doe")
.AsEnumerable()
.Single();
Console.WriteLine("-------- Read a document---------");
Console.WriteLine(singleEmployee.Id);
Console.WriteLine(singleEmployee.Name);
Console.WriteLine(singleEmployee.Age);
Console.WriteLine("-------------------------------");
}
private static void CreateDocument(DocumentClient client)
{
Employee employee1 = new Employee();
employee1.Name = "John Doe";
employee1.Age = 30;
Task createEmployee = client.CreateDocumentAsync
(ConfigurationManager.AppSettings["CollectionLink"], employee1);
Task.WaitAll(createEmployee);
}
Resources
The post Azure DocumentDB – CRUD Operations appeared first on Tech Musings - Anooj Nair.