In this section, we’ll delve further and enhance the app where we stopped last time. Now, let us go ahead and improve the UI a bit, so that it feels good and look good. In order to get started, I will make use of some bootstrap templates which you can refer here @ Bootstrap. Now, from here, I will pick Cyborg theme as shown below in the screenshot.
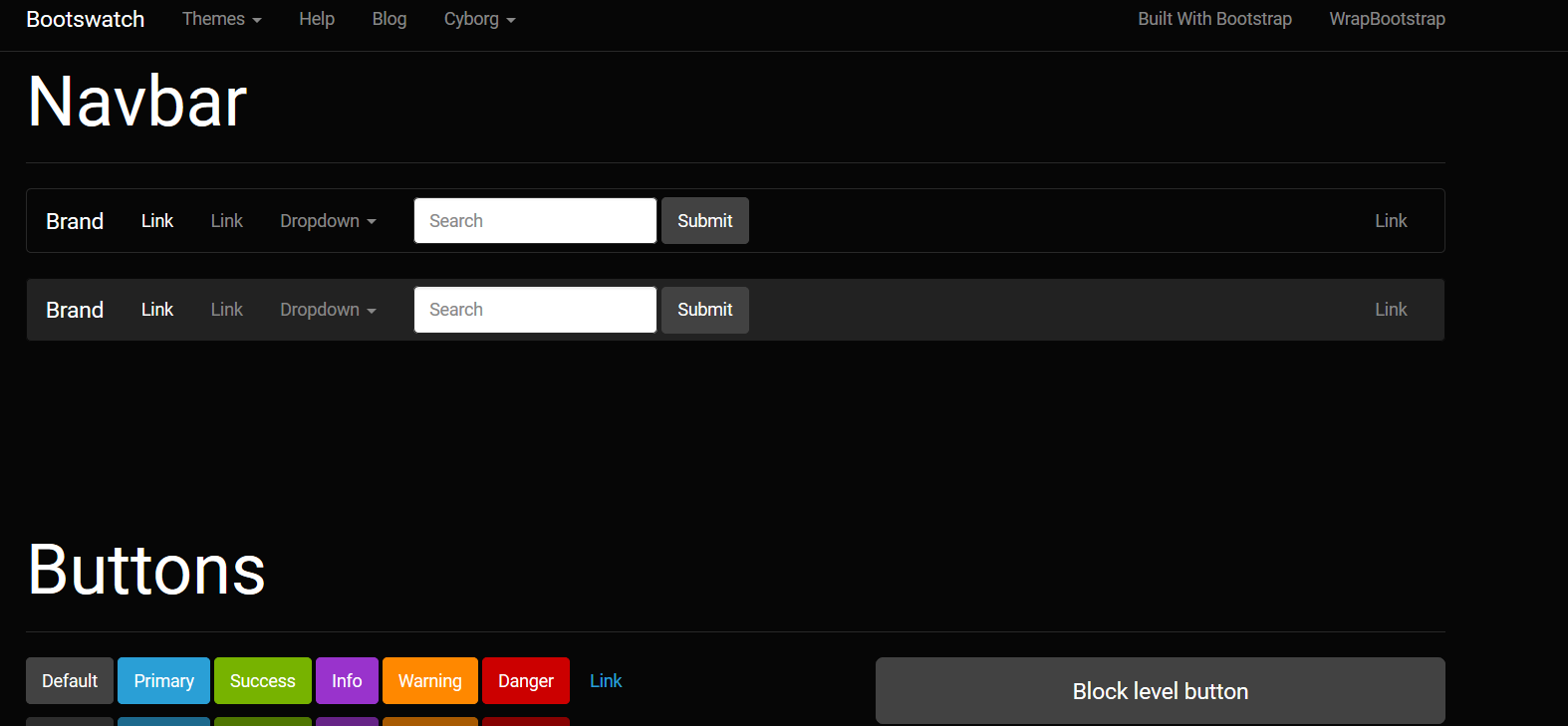
From here, I will just go ahead and download the CSS file and save in my browser components as shown below in the screenshot. I could have placed it in my app styles folder as well, but there my fonts and other dependent items are not there, hence it’s good to have consistency and place it in one place. Here, I downloaded two themes, cyborg and superhero.

Then, I commented the initial reference of bootstrap and referenced new one as shown below:
<!--
<!--
<!--
<!--
<!--
<!--
<!--
<!--
<link rel="stylesheet" href="../bower_components/bootstrap/dist/css/bootstrap-superhero.css" />
<link rel="stylesheet" href="styles/main.css">
With the above change in place, it produced the below theme:

Now, it feels better. I have also improved a bit in the movies template with the below snippet:
<div>
<header>
<div class="header-content">
<div class="header-content-inner">
<h1>Getting Started with Angular JS</h1>
<hr>
<p>Learn Live with examples how to get started with Angular JS with complete demo</p>
<img src/>
</div>
</div>
</header>
</div>
<br/>
One more change I made for menu highlighting with the minor change is shown below:
<ul class="nav navbar-nav">
<li ui-sref-active="active"><a href="#/">Home</a></li>
<li ui-sref-active="active"><a ng-href="#/movies">Movies</a></li>
<li ui-sref-active="active"><a ng-href="#/">Contact</a></li>
</ul>
With the above change in place, it will look like shown below:


Now, let us go ahead and create Movies
controller to put some data in. For doing that, I have used my yo generator to do the same.



Therefore, this is the benefit of using yo generator. Out of the box, it gave me test file as well. Now, let us go ahead and put some data in there to display on the page. Of course currently, it will be static data. Later, we will see how to couple the same with data fetched from the server. By the way, the below snippet is the default one created by the generator.
'use strict';
angular.module('yeomanApp')
.controller('MoviesCtrl', function () {
this.awesomeThings = [
'HTML5 Boilerplate',
'AngularJS',
'Karma'
];
});
Looks ok to me. But, I don’t need this stuff, hence I will modify the same and make some necessary changes as shown below:
'use strict';
angular.module('yeomanApp')
.controller('MoviesCtrl', function ($scope) {
$scope.show ={
name: 'Jurassic World',
date: '12/06/2015',
time: '07:30 pm',
location:{
address:'IMAX',
city:'Bangalore',
province:'KA'
},
imageUrl:'images/movie-background.jpg',
movies:[
{
name:'Titanic',
directorName:'James Cameron',
duration:'2 hr',
about:'This movie was about great Titanic Ship'
},
{
name:'Tomorrow Never Dies',
directorName:'Roger Spottiswoode',
duration:'1 hr',
about:'Crazy James Bond Movie'
},
{
name:'Die Another Day',
directorName:'Lee Tamahori',
duration:'3 hr',
about:'Crazy James Bond Movie'
}
]
};
});
Now, I need to do corresponding view changes:
<div ng-controller="MoviesCtrl">
<header>
<div class="header-content">
<div class="header-content-inner">
<h1>Getting Started with Angular JS</h1>
<hr>
<p>Learn Live with examples how to get started with Angular JS with complete demo</p>
<img ng-src="{{show.imageUrl}}"
alt="{{show.name}}" style="width:1000px;height: 300px;"/>
</div>
</div>
</header>
<div class="row">
<div class="span11">
<h2> {{show.name | uppercase}}</h2>
</div>
</div>
<hr/>
<div class="row">
<div class="span3">
<div><strong>Date:- {{show.date | date:'short'}}</strong></div>
<div><strong>Time:- {{show.time}}</strong></div>
</div>
<div class="span4">
<address>
<strong>Address:</strong><br/>
{{show.location.address}}<br/>
{{show.location.city}},{{event.location.province}}
</address>
</div>
</div>
<hr/>
<div class="row">
<h3>Movies</h3>
<div>
<div ng-repeat="movie in show.movies">
<div class="row show">
<div class="well span9">
<h4 class="show-title">{{movie.name}}</h4>
<h6 style="margin-top: -10ox;">{{movie.directorName}}</h6>
<span>Duration: {{movie.duration}} </span>
<p>About: {{movie.about}}</p>
</div>
</div>
</div>
</div>
</div>
</div>
<br/>
With the above change in place, it will produce the below result. Although the page doesn’t look super impressive, that’s ok. UI is least bothered for now.


I have also added two more views for Login page and Register as shown below in the screenshot.


We will see the functionality of this in the coming section. Till then, stay tuned and happy coding!
Thanks for reading!