CodeProject
OData is a standardized protocol for creating and consuming data APIs. OData builds on core protocols like HTTP and commonly accepted methodologies like REST. The result is a uniform way to expose full-featured data APIs.
OData Service enables rich querying using URI query string parameters, For e.g.
http://ODataServiceTest/HumanResourceDataService.svc/Employees?$filter=JobTitle eq ‘Design Engineer’
More querying options are available here.
For this tutorial i am using Adventure Works Database, Download is available at codeplex,
http://msftdbprodsamples.codeplex.com/releases/view/55330
For below tutorial I used SQL Server 20008R2 with Visual Studio 2010 (.NET 4.0).
Below is the step-by-step tutorial to create a OData Service Class project.
1. Download Adventure Works database and setup it in local SQL server.
2. Create a new class library project “AdventureWorks.HumanResource.DataAccess”
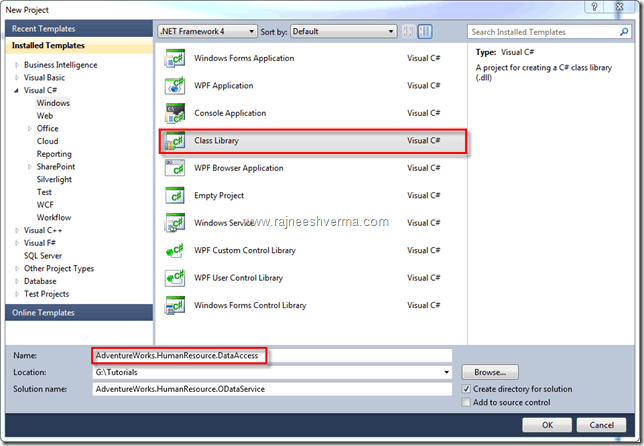
3. Delete Class1.cs file
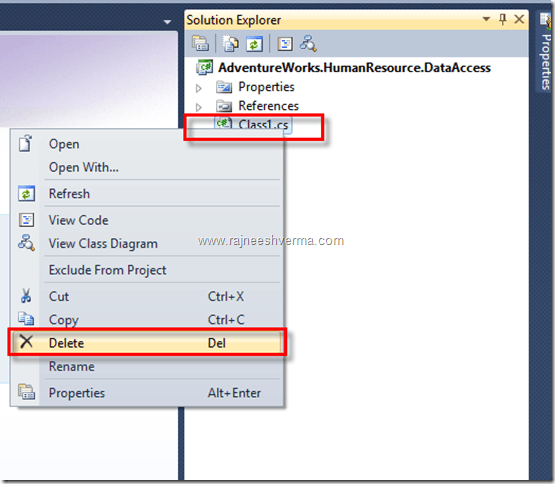
4. Right click on project in solution explorer and click Add –> New Item, Select Data from left tree and ADO.NET Entity Data Model from right pane. Give the name as “HumanResource.edmx” and click Add.
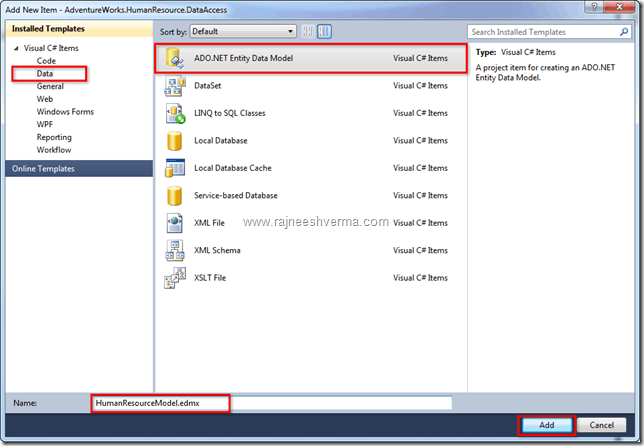
5. In Entity Data Model wizard select Generate from database and click next.
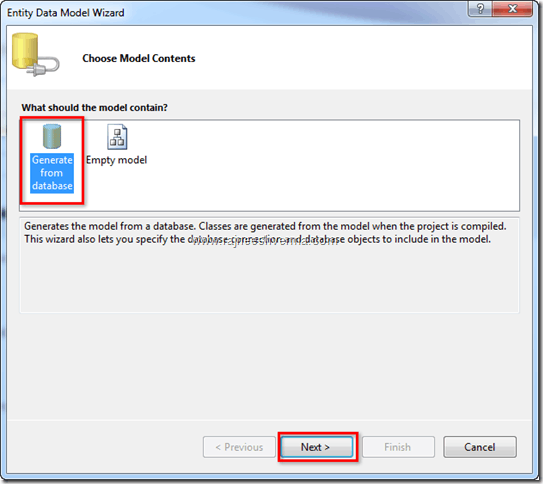
6. Create a new connection to Adventure Works database as below.Test connect and click OK to continue.
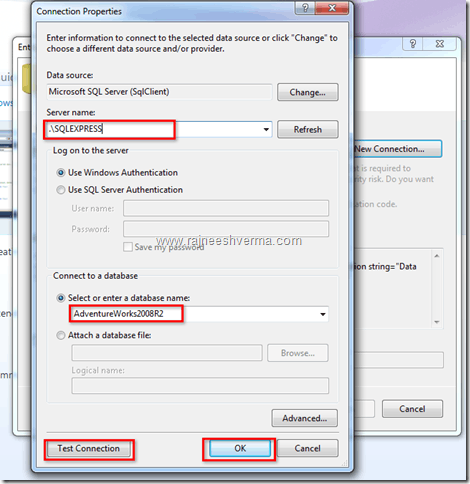
7. You will see connection details as below
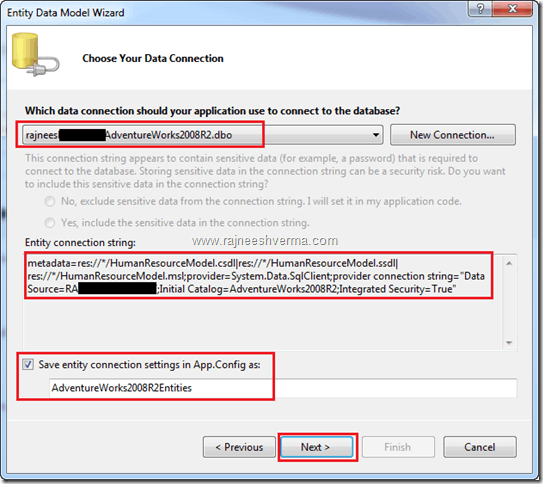
8. Now option to choose database objects for OData, for this sample I am selecting only Tables (HumanResources schema), In later tutorial I will explore views and stored procedures for OData service.
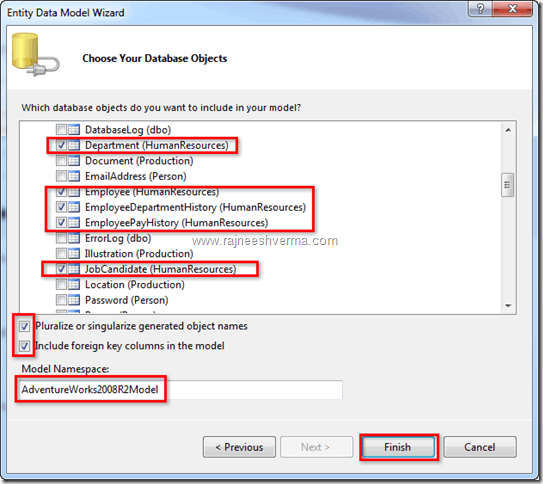
9. After clicking Finish button, VS will create Entity Diagram, for entities make sure you have proper relation between tables.
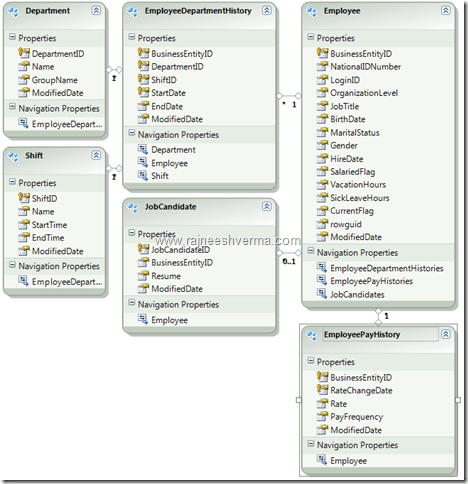
10. Build the project, DataAccess project is ready with OData.
Now create a new WCF Data Service project and refer DataAccess dll in it.
1. Go to solution explorer, Add –> New Project
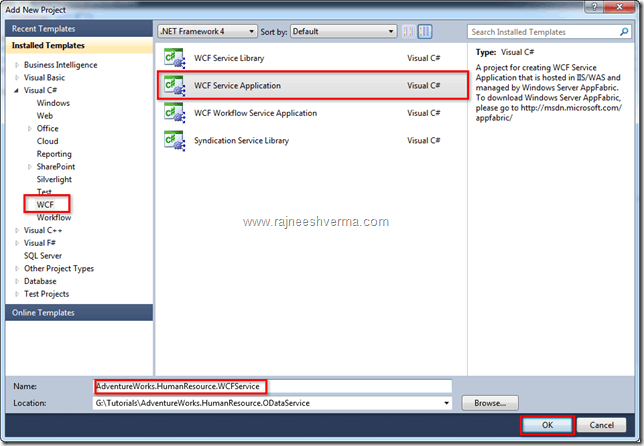
2. Delete Service1.svc & IService.cs files
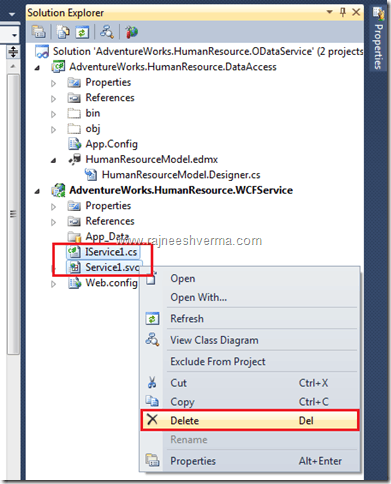
3. Add –> New Item… select WCF Service and named it as “HumanResourceDataService.svc”
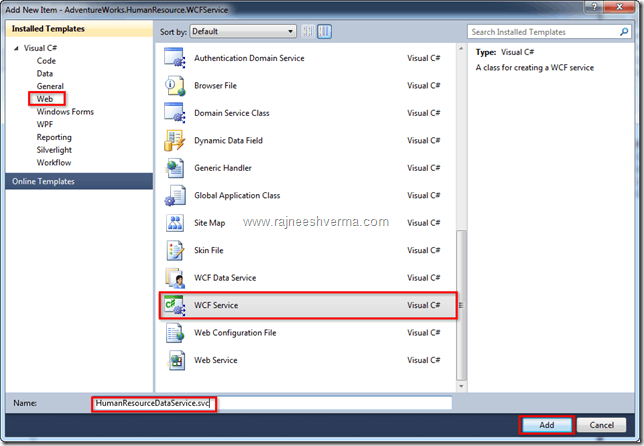
4. Add “AdventureWorks.HumanResource.DataAccess.dll” using Add Reference…
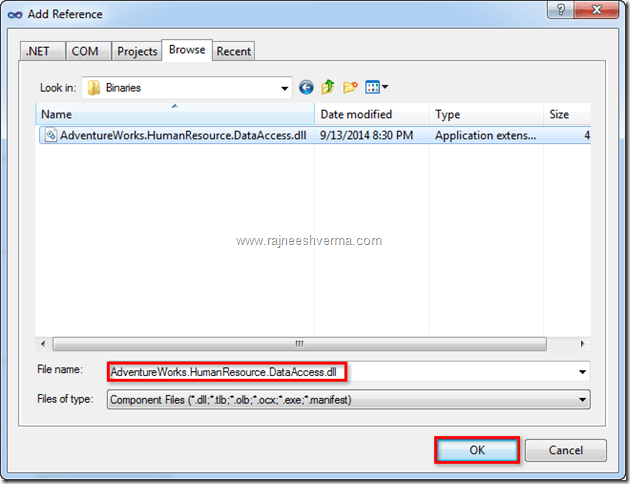
5. Add below dlls reference (you can add these dlls using Manage NuGet packages)
a) Microsoft.Data.Edm.dll
b) Microsoft.Data.OData
c) Microsoft.Data.Services
d) Microsoft.Data.Services.Client
e) System.Spatial.dll
6. HumanResourceDataService.svc.cs code is as below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
using AdventureWorks.HumanResource.DataAccess;
using System.Data.Services;
using System.Data.Services.Common;
namespace AdventureWorks.HumanResource.WCFService
{
public class HumanResourceDataService : DataService<AdventureWorks2008R2Entities>
{
public static void InitializeService(DataServiceConfiguration config)
{
config.SetEntitySetAccessRule("*", EntitySetRights.AllRead);
config.DataServiceBehavior.MaxProtocolVersion = DataServiceProtocolVersion.V3;
}
}
}
7. HumanResourceDataService.svc code is as below:
<%@ ServiceHost Language="C#" Debug="true" Factory="System.Data.Services.DataServiceHostFactory,
System.Data.Services, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"
Service="AdventureWorks.HumanResource.WCFService.HumanResourceDataService" %>
8. Add new endpoint for data service with webHttpBinding
, code snippet will be as below:
<? xml version="1.0" encoding="utf-8"?>
<configuration>
<connectionStrings>
<add name = "AdventureWorks2008R2Entities" connectionString="metadata=res://*/HumanResourceModel.csdl|
res://*/HumanResourceModel.ssdl|res://*/HumanResourceModel.msl;provider=System.Data.SqlClient;provider connection
string="data source=RAJNEESH\SQLEXPRESS;initial catalog=AdventureWorks2008R2;integrated
security=True;multipleactiveresultsets=True;App=EntityFramework"" providerName="System.Data.EntityClient" />
</connectionStrings>
<system.web>
<compilation debug = "true" targetFramework="4.0" />
</system.web>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior>
<!-- To avoid disclosing metadata information, set the value below to false and remove the metadata endpoint
above before deployment -->
<serviceMetadata httpGetEnabled = "true" />
<!-- To receive exception details in faults for debugging purposes, set the value below to true. Set to false
before deployment to avoid disclosing exception information -->
<serviceDebug includeExceptionDetailInFaults = "false" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service name = "KPMG.eAudIT.QueueService.WASHost.HumanResourceDataService" >
<endpoint address = "" binding="webHttpBinding" bindingConfiguration="webhttpBinding"
contract="System.Data.Services.IRequestHandler">
</endpoint>
</service>
</services>
<serviceHostingEnvironment multipleSiteBindingsEnabled = "true" />
<bindings>
<webHttpBinding>
<binding name = "webhttpBinding" maxBufferSize="2147483647" maxBufferPoolSize="2147483647"
maxReceivedMessageSize="2147483647" receiveTimeout="01:00:00" sendTimeout="01:00:00">
<readerQuotas maxDepth = "2147483647" maxStringContentLength="2147483647" maxArrayLength="2147483647"
maxBytesPerRead="2147483647" maxNameTableCharCount="2147483647" />
<security mode = "TransportCredentialOnly" >
<transport clientCredentialType = "Windows" />
</security>
</binding>
</webHttpBinding>
</bindings>
</system.serviceModel>
<system.webServer>
<modules runAllManagedModulesForAllRequests = "true" />
</system.webServer>
</configuration>
9. WCF service is ready to host, host the service in local iis or run directly from visual studio and see the result as below.
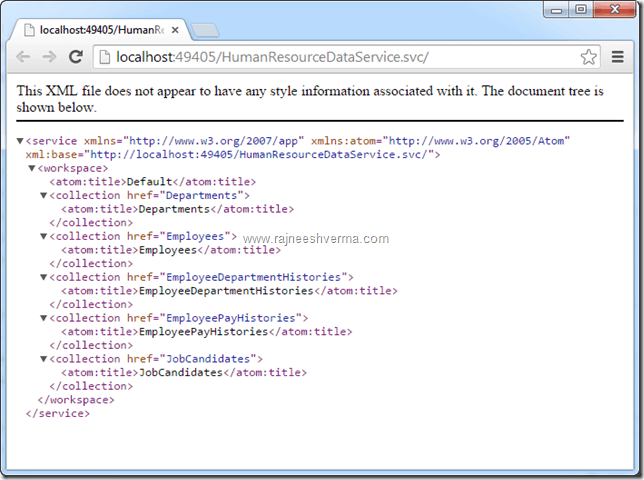
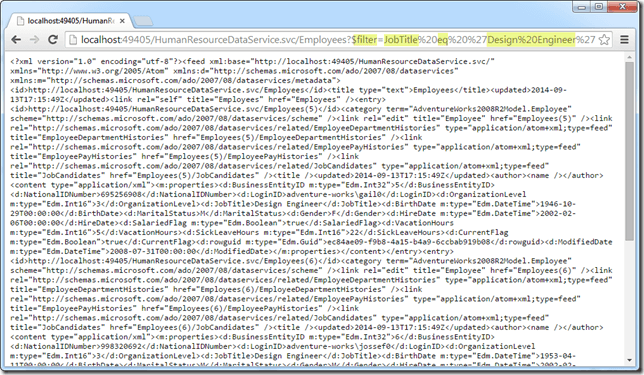
10. Use LINQPad to see the results and query the data.
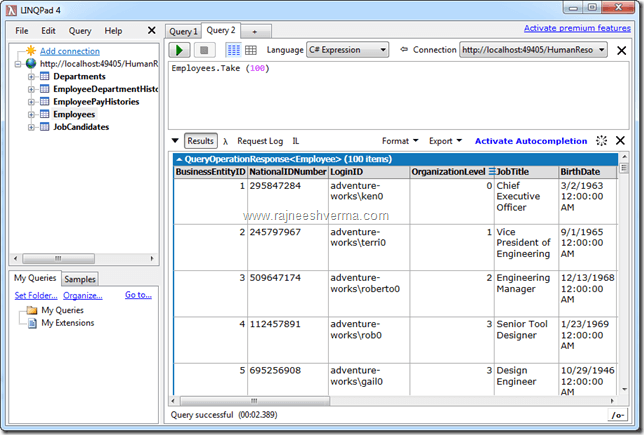
11. Source code download from here