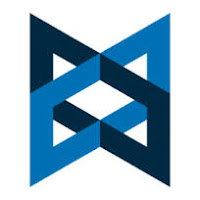 |
Backbone.js Models
|
When we talk about MVC pattern, Model becomes very important part of this. It is the Model which contains application data. The authors of backbone.js have quite a clear definition of what they believe the model represents in
Backbone.js.
Models are the heart of any JavaScript application, containing the interactive data as well as a large part of the logic surrounding it: conversions, validations, computed properties, and access control.
What we have till now-
In my last post
Backbone.js, We have created a HTML page named
main.html in which we had included the required script files. Let's create a new js file
main.js and includes this file in our
main.html page.
<HTML>
<Head>
<title>Backbone js Tutorial</title>
</Head>
<Body>
<script src="scripts/underscore-min.js"></script>
<script src="scripts/jquery-1.11.3.min.js"></script>
<script src="scripts/backbone-min.js"></script>
<script src="scripts/main.js"></script>
</Body>
</HTML>
Defining the Model-
You can define the model by extending the
Backbone.Model class.
var Person = Backbone.Model.extend({
defaults:{
FName:"Manish",
LName:"Dubey",
Age : 28
}
});
In the above code , we are defining a model
"TodoItem" with some default values like
"FName" ,
"LName" and
"Age".
Instantiating the Model-
You can simply instantiate the model using
new keyword.
var person = new Person();
Getters-
In backbone, you can get the attribute values using the
get method.
person.get('Fname');
person.get(Age'); // will return Age value
Setter-
You can also update the attribute values using
set method
person.set("FName", "Deepak");
person.set("Age", 30);
You can also update the values in one go-
person .set({FName:’Deepak’,Age:30});
Deleting a Model-
You can delete the model using
destroy function.
person.destroy();
Json Output-
Todoitem.toJSON()
Output on Browser Console-
Now launch the
main.html page in browser and run the above commands, you will get the below outputs.
CodeProject
