This time, we will look at how you can use the various loops within Scala.
For Loops
The for
loop in Scala is quite handy, and does some things that other languages do not provide within their for
loop symantics.
Range
The simplest for
of a for
loop is as shown here:
var a = 0
for(a <- 1 to 10) {
println(s"Value of a = $a")
}
Which would give the following results:

You can also use until
instead of to
, which would give you the same results.
Collections
You can also use a for
loop to loop through a collection:
var list = List(1,2,3,4)
var a = 0
for(a <- list) {
println(s"Value of a = $a")
}
Which would give the following results:

Using Filters
Filters may also be applied to for
loops, here is an example:
var list = List(1,2,3,4)
var a = 0
for(a <- list if a % 2 ==0) {
println(s"Value of a = $a")
}
Which when run gives the following results:
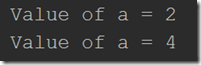
Using Yield
You can also create two new sequences (if you are a .NET programmer which is really who these posts are aimed at, this would be the same as using a LINQ Select
to create a new IEnumerable
for some source IEnumerable
).
var list = List(1,2,3,4)
var a = 0
var evens = for {a <- list if a % 2 ==0
} yield a
for(a <- evens) {
println(s"Value of a = $a")
}
Which when run produces the following results:
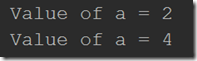
While Loops
Much the same as other languages, Scala has a while
loop that allows us to continue looping while waiting for a certain condition to be met. It is important to note that the while
loop checks the condition at the top of the loop, so if the condition is already met the while
loop may never run.
var a = 0
while(a < 10) {
println(s"Value of a = $a")
a = a + 1
}
Which when run will give the following result:

Do While Loops
Scala also has the popular do
-while
loop, which unlike the while
loop will check the condition at the end of the do
-while
, so is guaranteed to run at least once. Here is a simple example:
var a = 0
do {
println(s"Value of a = $a")
a = a + 1
}while(a < 10)
import scala.util.control._
val loop = new Breaks()
var a = 0
loop.breakable {
for(a <- 0 to 20) {
println(s"Value of a = $a")
if(a > 5)
loop.break()
}
}
println("Outisde the loop")
Which when run will give you the following results:

Breaking Out Of Loops
This is one key area where Scala is very different from .NET. There is no built in break
statement available.
However, if you are using a version of Scala after 2.8, then there is a way to use a break
statement inside your loops.
You need to import a package to allow this to happen.
The idea is that you wrap your loop in a breakable which is available by using the Breaks
class. And then inside your loop, when some condition occurs, you may use Breaks
classes break()
method.
Let's see an example:
import scala.util.control._
val loop = new Breaks()
var a = 0
loop.breakable {
for(a <- 0 to 20) {
println(s"Value of a = $a")
if(a > 5)
loop.break()
}
}
println("Outisde the loop")
Which when run gives the following results:
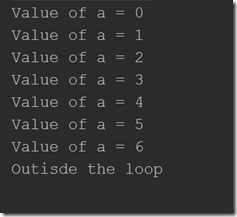