Introduction
What Is a Regular Expression?
A regular expression is a sequence of characters that forms a search pattern. The search pattern can be used for text search and text replace operations. When you search for data in a text, you can use this search pattern to describe what you are searching for. In practice, a regular expression can be a single character, or a more complicated pattern.
In JavaScript, everything is object and so does with regular expression. Regular expressions are patterns used to match character combinations in string
s. These patterns are used with the exec()
and test()
methods of RegExp
, and with the match
, replace
, search
, and split
methods of String
. It can be used to perform all types of text search and text replace operations.
Preview
Here is a simple JavaScript regular expression:
var pattern = /example/i
/example/i
is a regular expression. example is a pattern (to be used in a search). i
is a modifier (modifies the search to be case-insensitive).
Steps to Use Regular Expression
- JavaScript regular expression preparation
- Regular expression pattern
- Regular expression modifier
- Using regular expression object
- Using regular expression for
string
JavaScript Regular Expression Preparation
In JavaScript, regular expression object consists of regular expression pattern and modifiers. Syntax is like:
/pattern/modifiers;
Regular expression pattern specifies the search pattern. A pattern is composed of simple characters, such as /abc/
, or a combination of simple and special characters, such as /ab
c/
or /Chapter (\d+).\d/
.
Pattern Table
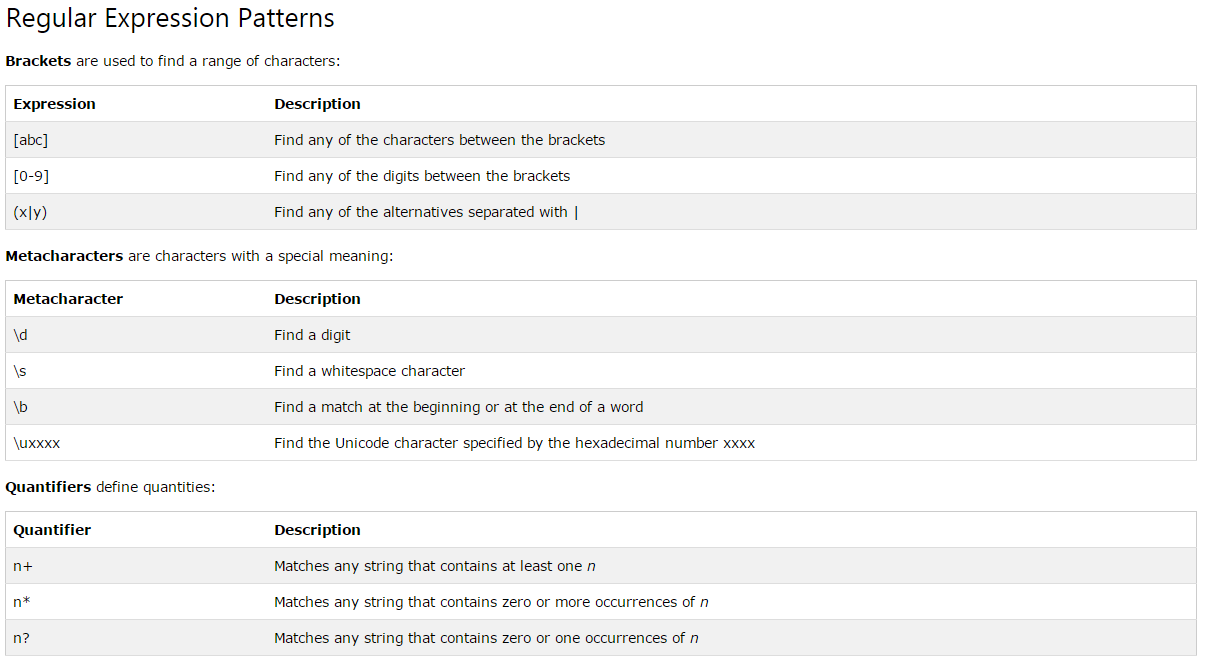
Adapted from w3schools
Modifiers specify if our search should be case-sensitive, global, etc. They are used to perform case-insensitive and global searches:
Modifiers Table

Adapted from w3schools
Now we are ready to apply regular expression in js. There are two basic ways to apply regular expression in JavaScript - Using regular expression object - Using regular expression for string
Using Regular Expression Object
Create a Regular Expression Object
A regular expression object describes a pattern of characters. It is used to perform pattern-matching. There are two ways to construct a regular expression object.
Way 1 - Using a regular expression literal, which consists of a pattern enclosed between slashes, as follows:
var reg = /ab+c/;
Regular expression literals provide compilation of the regular expression when the script is loaded. When the regular expression remains constant, use this for better performance.
Way 2 - calling the constructor function of the RegExp
object, as follows:
var reg = new RegExp("ab+c");
Using the constructor function provides runtime compilation of the regular expression. Use the constructor function when you know the regular expression pattern will be changing, or you don’t know the pattern and are getting it from another source, such as user input.
Regular Expression Object Methods
First, let’s get familiar with some common methods of regular expression object:
compile() Deprecated in version 1.5. Compiles a regular expression
exec() Tests for a match in a string. Returns the first match
test() Tests for a match in a string. Returns true or false
toString() Returns the string value of the regular expression
Examples:
Using test()
The test()
method is a RegExp expression method. It searches a string
for a pattern, and returns true
or false
, depending on the result.
The following example searches a string
for the character “e
”:
var patt = /e/;
patt.test("The best things in life are free!");
Since there is an “e
” in the string
, the output of the code above will be:
true
You don’t have to put the regular expression in a variable first. The two lines above can be shortened to one:
/e/.test("The best things in life are free!");
Using exec()
The exec()
method is a RegExp
expression method. It searches a string
for a specified pattern, and returns the found text. If no match is found, it returns null
.
The following example searches a string
for the character “e
”:
/e/.exec("The best things in life are free!");
Since there is an “e
” in the string
, the output of the code above will be:
.e
Using Regular Expression for String
In JavaScript, regular expressions can also be used with the two string
methods: search()
and replace()
. They are used to handle two fundamental operations - make search
and replace
functions on text.
The search()
method uses an expression to search for a match, and returns the position of the match.
The replace()
method returns a modified string
where the pattern is replaced.
Examples:
Use a regular expression to do a case-insensitive search for “w3schools
” in a string
:
var str = "Visit W3Schools";
var n = str.search(/w3schools/i);
The result in n
will be:
6
The search
method will also accept a string
as search argument. The string
argument will be converted to a regular expression:
Use a string
to do a search for “W3schools
” in a string
:
var str = "Visit W3Schools!";
var n = str.search("W3Schools");
Use a case insensitive regular expression to replace Microsoft with W3Schools
in a string
:
var str = "Visit Microsoft!";
var res = str.replace(/microsoft/i, "W3Schools");
The result in res
will be:
Visit W3Schools!
The replace()
method will also accept a string
as search argument: var str = “Visit Microsoft!”; var res = str.replace(“Microsoft”, “W3Schools”);