Introduction
In the previous article I explained how to use AngularJs Interceptors to display generic notifications based on Http methods. In this article I will explain the actual implementation of the Notification Service. I am using Typescript with AngularJs.
Background
The following use cases are required:
- Display a message to end user based on the action e.g. Save, Update, Delete, Load and block / unblock the UI
- Display on demand spinner with message
- Display business / system exception using toasts
The followings are examples of the notifications:
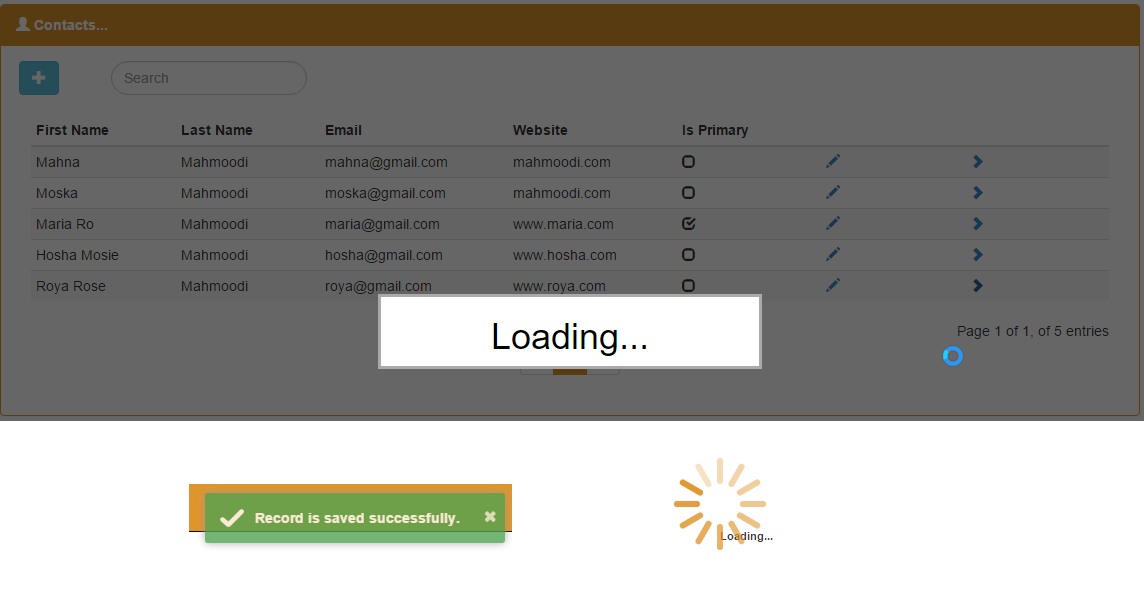
Using the code
I am using the following Angular / jQuery plugins for the Notification Service:
- To show message and block the UI, I use jQuery.BlockUI library.
- For on demand spinner, I use Angular-Loading library
- To show the business exception messages, I use the popular Toastr library
The full Notification Service code is as following:
module CRM.Common {
export interface INotificationService {
blockUi(message?: string): void;
unblockUi(): void;
showError(message): void;
showSucesss(message: string): void;
showProgress(): void;
showInfo(message: string): void;
showSpinner(name): void;
showMainPageSpinner(): void;
hideSpinner(name): void;
hideMainPageSpinner(): void;
}
export class NotificationService implements INotificationService {
private toaster: any;
static $inject = [
"toaster",
"$loading"
];
constructor(
toaster: ngtoaster.IToasterService,
private $loading: any
) {
this.toaster = toaster;
}
blockUi(message?: string): void {
if (message) {
$.blockUI({ message: message });
} else {
$.blockUI({ message: 'Please Wait' });
}
}
unblockUi(): void {
$.unblockUI();
}
showError(message: string): void {
this.toaster.error(message);
}
showSucesss(message: string): void {
this.toaster.success(message);
}
showProgress(): void {
$.blockUI({ message: 'Please Wait ...' });
}
showInfo(message: string): void {
this.toaster.info(message);
}
showSpinner(name:string): void {
this.$loading.start();
}
showMainPageSpinner(): void {
this.$loading.start("main-page-spinner");
}
hideMainPageSpinner(): void {
this.$loading.finish("main-page-spinner");
}
hideSpinner(name): void {
this.$loading.finish(name);
}
}
angular.module("CRM").service("CRM.Common.NotificationService", NotificationService);
}
This is how to use the service inside the controller:
static $inject = [
"CRM.Common.NotificationService"];
constructor(private popupService: CRM.Common.INotificationService) {
}
this.popupService.showError(error.data.message);
Or
this.popupService.showSuccess("Record is saved successfully.");
Would like to know what everyone else is using?
Happy holidays!