Introduction
NB: This is a tiny article, but more than a tip - I'm sure I'll find more to add to it down the road, but for the moment, it is what it is, and provides a fully working solution. :)
I had a requirement recently to be able to click a button, and send a tweet, without having to compose it.. 'one click tweet' let's say (well, in its actually two clicks, but!!) .. anyway, I didn't have the time to hook into the twitter API and do the entire OAuth thing, and frankly, for the needs of this mini-project, that would have been total overkill. My simple requirement was - have a list of potential statuses on a list in front of me, and be able to click one to send. Fast, simple. This is the quick and dirty solution!
Step 1 - Click !
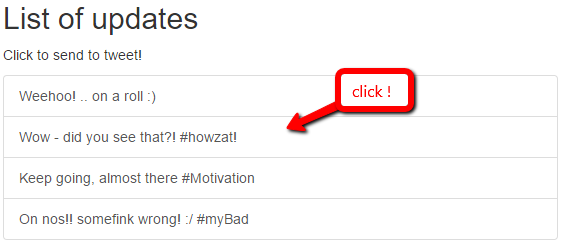
Step 2 - Click !

Using the Code
Everything about this little solution is very standard - first, setup some simple HTML, and style with our friend Bootstrap. Stick in a class name (in this case 'shoutout
') that we will use to hook our JavaScript to.
<div class="list-group">
<a href="#" class="list-group-item list-group-item-success shoutOut">Weehoo! .. on a roll :) </a>
<a href="#" class="list-group-item list-group-item-info shoutOut">Wow - did you see that?! #howzat!</a>
<a href="#" class="list-group-item list-group-item-warning shoutOut">Keep going, almost
there #Motivation</a>
<a href="#" class="list-group-item list-group-item-danger shoutOut">On nos!! somefink
wrong! :/ #myBad</a>
</div>
Next, stick in some Javascript/JQuery (whatever your drink of choice) that grabs the context of what was clicked by hooking into anything that uses the 'shoutOut
' class, and captures its 'innerText
'. Finally, encode the innerText
, and open a new browser window with a target of '_blank
', to the constructed URL string var
'tweetOut
'.
<script>
$('.shoutOut').click(
function(){
var tweetOut = 'https://twitter.com/intent/tweet?text=' + encodeURIComponent(this.innerText)
window.open(tweetOut, '_blank');
return false;
}
)
</script>
Points of Interest
Three things of note:
- You need to have your browser logged into Twitter before using the code for the first time, otherwise it naturally, takes more than two clicks. :)
- Ensure you use the '
encodeURIComponent
' method so that any hashtags, etc. get rendered correctly. - Make sure that the last line in your function returns
false
.
That's it, #JobDone.
If you need it, there's a small project attached with the code.
History
- 29th February, 2016 - V1. Published