Introduction
Many times we need to create/scan Barcode and QR code in mobile apps. So we will see how to generate barcode/QR code in Windows Universal app. For this we will use ZXing Barcode Scanning Library. It is available on Nuget for .Net developers.
Description
Take a blank Universal app. It will contain three types of project Windows Store app, Windows phone app and Shared project.
Now install ZXing Barcode Scanning Library .Net from Nuget
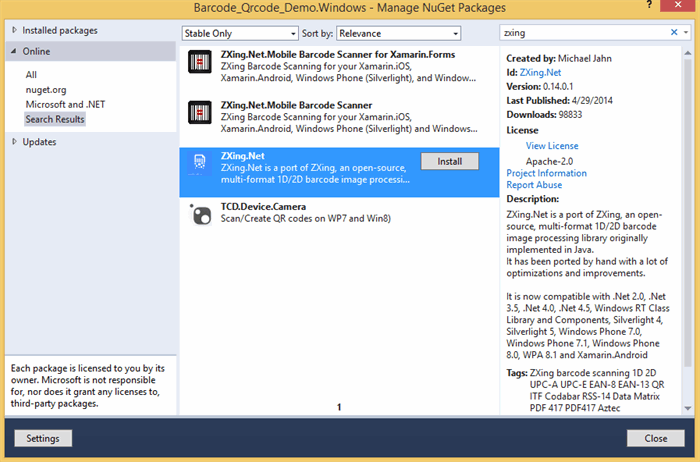
To install ZXing Barcode Scanning Library .Net Port from Package Manager Console, run the following command in the Package Manager Console
PM> Install-Package ZXing
You will need to install this library in both Windows Store and Windows phone apps.
Now add MainPage class in shared project because we will share code.
public sealed partial class MainPage : Page
{
}

Create layout in XAML to show Barcod and QR code images. layout will be separate for both apps.
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<StackPanel Grid.Row="0" VerticalAlignment="Center" Orientation="Horizontal">
<TextBlock Text="Barcode" Foreground="White" FontSize="25" VerticalAlignment="Center" Margin="10 0 10 0"></TextBlock>
<TextBox VerticalAlignment="Center" Width="150" Foreground="Black" FontSize="25" Margin="0 0 10 0" Name="txtBarcode"></TextBox>
<Button Content="Ok" Foreground="White" FontSize="25" Name="btnBarcode" Click="btnBarcode_Click"></Button>
</StackPanel>
<StackPanel Grid.Row="1" Grid.RowSpan="2" Background="White">
<Image Name="imgBarcode" Width="200" Height="200" HorizontalAlignment="Center" VerticalAlignment="Center"></Image>
</StackPanel>
<StackPanel Grid.Row="4" VerticalAlignment="Center" Orientation="Horizontal">
<TextBlock Text="QRcode" Foreground="White" FontSize="25" VerticalAlignment="Center" Margin="10 0 10 0"></TextBlock>
<TextBox VerticalAlignment="Center" Width="150" Foreground="Black" FontSize="25" Margin="0 0 10 0" Name="txtQRcode"></TextBox>
<Button Content="Ok" Foreground="White" FontSize="25" Name="btnQRcode" Click="btnQRcode_Click"></Button>
</StackPanel>
<StackPanel Grid.Row="5" Grid.RowSpan="2" Background="White">
<Image Name="imgQRCode" Width="200" Height="200" HorizontalAlignment="Center" VerticalAlignment="Center"></Image>
</StackPanel>
</Grid>
Create handler of Barcode and QRcode button click and generate images. There are multiple types of encoding options available in this library, you can use any according to your requirement.
private void btnBarcode_Click(object sender, RoutedEventArgs e)
{
IBarcodeWriter writer = new BarcodeWriter
{
Format = BarcodeFormat.CODE_128,
Options = new ZXing.Common.EncodingOptions
{
Height = 100,
Width = 450
}
};
var result = writer.Write(txtBarcode.Text);
var wb = result.ToBitmap() as WriteableBitmap;
imgBarcode.Source = wb;
}
private void btnQRcode_Click(object sender, RoutedEventArgs e)
{
IBarcodeWriter writer = new BarcodeWriter
{
Format = BarcodeFormat.QR_CODE,
Options = new ZXing.Common.EncodingOptions
{
Height = 160,
Width = 160
}
};
var result = writer.Write(txtQRcode.Text);
var wb = result.ToBitmap() as WriteableBitmap;
imgQRCode.Source = wb;
}
Output
