Introduction
This writing will focus on the need, implementation and using EntityManager
API in your project. The sole purpose of this API is to protect your passwords and other important data from getting hacked. This API basically focuses on the encryption of hardcoded credentials. It is a well known fact that any .jar or .class file can be reverse engineered and any experienced person can snoop your hard coded passwords. "Hard Coding" means something that you want to embed with your program or any project that cannot be changed directly. For example, if you are using a database server, then you must hardcode the credentials to connect your database with your project and that cannot be changed by the user. Because you have hard coded it. Sometimes, embedded data can be confidential and we would never want to reveal it to the user/snooper. So basically, hard coding is not a good practice. It is one of the top coding mistakes made by programmers that lead their application to be hacked. There are several methods available on the internet to avoid the hardcoding of the important data. But almost all the methods are very complex for new buddies to implement. But don’t get worried, here is ‘EntityManager
’ API to help you.
What is 'EntityManager API' and How Does It Work?
Basically, this is a Java API, developed under Java and supports SE 1.7+. It encrypts your password (data) through SYBO encryption and stores the data into .sybo file. You can easily use our GUI to manage your data.
Using the API
- Download the API from Github. Unzip the zipped file wherever you want.
- Run EntityManager.jar
- Add as much as entity you want:
Example: Entity Name: password
Entity Value: 123@sybero
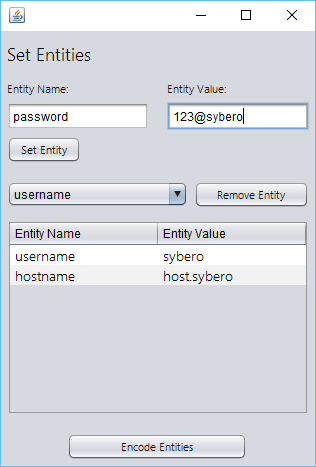
- Now click ‘Encode Entities’
- You will see a new file has been created as ‘entities.sybo’. Copy this file and save it at a safe place as it will act as the source of data.
- Now import the EntityManager.jar in your project and copy ‘entities.sybo’ to your project folder. The location will be as:
In Windows:
C:\Users\Sybero Home\Documents\NetBeansProjects\<YourProjectName>
- Sample program using this API:
File name: Example.java
import entitymanager.Entity;
import entitymanager.EntityException;
public class Example {
public static void main(String[] args) {
try{
Entity entity = new Entity();
System.out.println("user : "+entity.getEntity("username"));
System.out.println("pass : "+entity.getEntity("password"));
System.out.println("host : "+entity.getEntity("hostname"));
}catch(EntityException e){
System.out.println(e.getMessage());
}
}
}
Output:
sybero
123@sybero
host.sybero
- Now develop your project as you want, but remember to add ‘entities.sybo’ as your project source, the file must be in the folder containing your built <YourProjectName>.jar during distribution.
Edit or Add Some More Details
How to edit or add some more details in ‘entities.sybo’ ?
For example, you forget to add some password to the ‘entities.sybo’ file and you want to add these data right now. Simply delete the previous ‘entities.sybo’ file and create a fresh file following the previous process and add copy it to your project folder.
Have a Problem
If you have any issues/problems, add a comment/question or feel free to contact me at rohit.sybero@rediffmail.com or rohit7209@rediffmail.com.