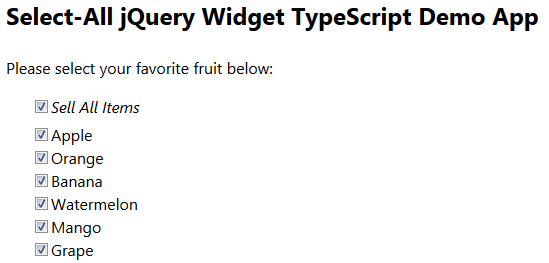
Introduction
This is a simple jQueryUI widget that adds SELECT ALL
function to Checkbox
input on webpage and it is developed using TypeScript in Visual Studio 2015.
Using the Code
You can just pull the source files from github at https://github.com/matthewycso/jquery-select-all.git.
The JavaScript file jquery-select-all.js is the compiled JavaScript from TypeScript for the jquery-select-all
widget of JQueryUI.
To use this widget, in your webpage, add references to jQuery, jQueryUI and file jquery-select-all.js as shown below. Then use jQuery in JavaScript to select the HTML element that will be assigned the select all
function, e.g., checkbox
with id="selectALL"
in the below example, and apply the selectall
method.
The widget has only one option checkboxSelector
which is used to select all checkbox
es that will be changed by the jquery-select-all
widget when it toggles for changing.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.2.4/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.js"></script>
<script src="jquery.select-all.js"></script>
<script src="app.js"></script>
//... In your page javascript section
$(function () {
// Set selectall jquery ui widget
$('#selectALL').selectall({ checkboxSelector: '#chooseFavories :checkbox' });
})
Points of Interest
The selectall Widget
The code shown below is all we needed to create a widget with the select all
feature.
Note that when any of the affected checkbox
changed by user, the select all
widget attached to checkbox
usually needs to be unchecked.
As TypeScript requires every type except any
have all defined properties declared or else it will complain during compilation. The last few lines below are to extend existing JQuery
interface with new selectall
method.
(function ($: JQueryStatic) {
$.widget("sforce.selectall", {
options: {
checkboxSelector: null
},
_create: function () {
let target: JQuery = this.element;
let $checkboxes = this.options.checkboxSelector !== null ?
$(this.options.checkboxSelector) :
$(this.element).closest('div, p, td, body').find(':checkbox');
target
.addClass("select-all")
.on('change.select-all.sforce', function () {
$checkboxes.not(target)
.prop('checked', target.prop('checked'))
.trigger('change') ;
});
$checkboxes.on('change.select-all.sforce', function () {
if (!$(this).is(target) && !$(this).prop('checked'))
target.prop('checked', false);
});
},
_destroy: function () {
this.element
.removeClass("select-all")
.off('select-all.sforce');
}
});
})(jQuery);
interface JQuery {
selectall: (options?: { checkboxSelector: JQuery|string }) => JQuery;
}
History