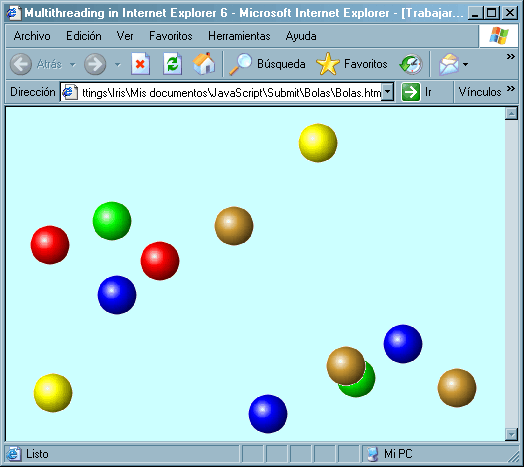
Introduction
This JavaScript code shows how to dynamically create threads in Internet Explorer that run at the same time.
Background
There is no JavaScript command to create and kill threads (and if there is I don't know it). But it is possible: threads can be created and killed with no ad-hoc code. This example shows how.
Using the code
As the user presses the Enter key or clicks the mouse, a new thread is created. This thread throws a ball to the page which bounces against the window borders. If you press the Delete key the last created thread is killed, and therefore the last thrown ball disappears. The balls are six .gif images which only differ in the color. Here comes the code:
<HTML>
<HEAD><TITLE>Multithreading in Internet Explorer 6</TITLE></HEAD>
<BODY onkeydown="KeyDown()"
onclick="GoAhead()" bgcolor="#CCFFFF">
</BODY>
</HTML>
<script language=javascript>
var IdCounter = 0
var Diameter = 40
var IdSubstring04 = "Ball"
function Bola(){
this.id = IdSubstring04
this.top = 0
this.left = 0
this.SignTop = 0
this.SignLeft = 0
}
function KeyDown(){
switch (window.event.keyCode){
case 13 :
document.body.click()
break
case 46 :
RemoveBall()
break
}
}
function GoAhead(){
var Ball = CreateBall()
ThrowBall(Ball.id, Ball.left, Ball.top,
Ball.SignLeft, Ball.SignTop)
}
function RemoveBall(){
var old = document.body.lastChild
if (old.id.substring(0, 4) == IdSubstring04)
document.body.removeChild(old)
}
function RandomNorM(n, m){
var x = Math.random()
if (x < 0.5) {return n} else {return m}
}
function CreateBall(){
var Ball = new Bola()
var x = RandomNorM(0, 1)
var Colors = new Array("red", "green",
"blue", "cian",
"gold", "yellow")
if (x == 0){
Ball.top = -1
Ball.left = Math.floor(Math.random() *
document.body.clientWidth) - Diameter
if (Ball.left < 0) Ball.left = 0
}
else{
Ball.top = Math.floor(Math.random() *
document.body.clientHeight) - Diameter
if (Ball.top < 0) Ball.top = 0
Ball.left = -1
}
Ball.SignLeft = RandomNorM(-1, 1)
Ball.SignTop = RandomNorM(-1, 1)
Ball.id += IdCounter
IdCounter++
var BallSpan = document.createElement("span")
BallSpan.setAttribute("id", Ball.id)
BallSpan.style.position = "absolute"
BallSpan.style.left = Ball.left
BallSpan.style.top = Ball.top
BallSpan.style.width = Diameter
BallSpan.style.height = Diameter
var ImageTag = document.createElement("img")
ImageTag.setAttribute("src","Bolas/" +
Colors[Math.ceil(Math.random() * Colors.length) - 1] +
".gif")
ImageTag.setAttribute("width", Diameter)
ImageTag.setAttribute("height", Diameter)
BallSpan.appendChild(ImageTag)
document.body.appendChild(BallSpan)
return Ball
}
function ThrowBall(BallId, BallLeft, BallTop, SignLeft, SignTop){
var BallElement = document.getElementById(BallId)
if (BallLeft + Diameter >= document.body.clientWidth) SignLeft =-1
if (BallLeft <= 0) SignLeft = 1
if (BallTop + Diameter >= document.body.clientHeight) SignTop =-1
if (BallTop <= 0) SignTop = 1
BallLeft += SignLeft
BallTop += SignTop
BallElement.style.left = BallLeft
BallElement.style.top = BallTop
var tilde = "'"
var comma = ","
window.setTimeout("ThrowBall(" + tilde + BallId + tilde +
comma + BallLeft + comma + BallTop + comma +
SignLeft + comma + SignTop + ")", 1)
}
</script>
The function CreateBall
creates an instance of the object Bola
(Ball in Spanish) defined by the Bola
function. This object encapsulates the ID, the position (fields top
and left
) of the ball, and the x (field SignLeft
) and y (field SignTop
) direction of its movement defined by the number 1 with positive or negative sign. The ID is generated by a counter (IdCounter
), and the position and direction as well as the color of the ball are generated with the help of the function RandomNorM(n, m)
, which returns randomly one of its two parameters. With all this information, the function CreateBall
creates a <span>
tag that looks like this one:
<span><img src="Bolas/red.gif" height=40 width=40></span>
which is the tag that will be dragged by its own code thread. Finally, the function returns the object Bola
.
ThrowBall
is responsible for the movement of the span
tag. It calculates the next position of the tag taking account of the bounces and then, provided with this new position, calls itself indefinitely so that the tag is always repositioned producing the effect of movement. It doesn't stop until the thread is killed or, obviously, the web page is closed. The function that kills the thread is RemoveBall
, which gets the last created ball by its ID and destroys the tag. After removing a thread, the statusbar of IE 6 displays an error message. I haven't found the error, but the script does work.