Do you also like the binary watches from IO?

OK, I like them and was inspired to make some binary clock for the Windows Mobile taskbar.

The time shown here is 14:23 displayed as binary dots. The top row (red) shows the hours in 24h format. The second row shows the minutes and the bottom row shows the seconds.

What you see here is encoded in binary:

01110 is equal to 0*16 1*8 1*4 1*2 0*1 = 8 + 4 + 2 = 14
10111 is equal to 1*16 0*8 1*4 1*2 1*1 = 16 + 4 + 2 + 1 = 23
00100 is equal to 0*16 0*8 1*4 0*2 0*1 = 4 = 4
So you see, it shows the time is 14:23:04
.
The code itself is not very interesting except for the WM_PAINT
one:
case WM_PAINT:
RECT rt;
hdc = BeginPaint(hWnd, &ps);
GetClientRect(hWnd, &rt); DEBUGMSG(1, (L"Received WM_PAINT: %ix%i\n", rt.right, rt.bottom));
SYSTEMTIME st;
GetLocalTime(&st);
bHour=(BYTE)st.wHour; theTime[0]=bHour;
bMin =(BYTE)st.wMinute; theTime[1]=bMin;
bSec =(BYTE)st.wSecond; theTime[2]=bSec;
for (int iRow = 0; iRow < 3; iRow++)
{
for (int bit = 0; bit < 6; bit++)
{
if (! (iRow == 0 && bit == 5) ) {
iTemp=bit;
bTemp=(((theTime[iRow] >> iTemp) & 1) == 1);
RECT rectC;
rectC.left=xMax - ((iOffsetX + (bit * rWidth)) + (iGapX * bit)); rectC.top=iOffsetY + (iRow * rHeight) + (iGapY * iRow);
rectC.right=rectC.left + rWidth; rectC.bottom=rectC.top + rHeight; if(bTemp){
SelectObject(hdc, thePens[iRow]);
SelectObject(hdc, theBrushes[iRow]);
}else{
SelectObject(hdc, hpenBlack);
SelectObject(hdc, hbrBlack);
}
Ellipse(hdc, rectC.left, rectC.top, rectC.right, rectC.bottom);
}
}
}
if(bRes)
DEBUGMSG(1, (L"Ellipse done\n"));
else
DEBUGMSG(1, (L"Ellipse failed: %i\n", GetLastError()));
EndPaint(hWnd, &ps);
return 0;
case WM_CLOSE:
A Binary Clock in C#
Here is something more to play with, a binary clock written for Compact Framework.
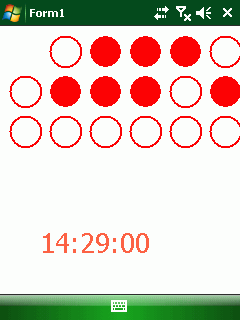
The code is as given below:
private void f1_paint(object sender,PaintEventArgs e) {
Graphics g = e.Graphics;
DateTime dt = DateTime.Now;
string s=string.Format("{0:HH:mm:ss}", dt);
g.DrawString(s,new Font("Verdana",20,FontStyle.Regular),
new SolidBrush(Color.Tomato),40,200);
byte bHour = (byte)dt.Hour;
byte bMin = (byte)dt.Minute;
byte bSec = (byte)dt.Second;
int iOffsetX = 10;
int iOffsetY = 10;
int rWidth = 30;
int rHeight = 30;
int xMax = 240-rWidth+iOffsetX;
byte[] bTimes = new byte[3];
bTimes[0] = bHour;
bTimes[1] = bMin;
bTimes[2] = bSec;
boolStruct[] boolTimes = new boolStruct[3];
boolTimes[0] = byte2bool(bHour);
boolTimes[1] = byte2bool(bMin);
boolTimes[2] = byte2bool(bSec);
for (int iRow = 0; iRow < 3; iRow++)
{
for (int bit = 0; bit < 6; bit++)
{
if (boolTimes[iRow].boolList[bit])
{
if (!((iRow == 0) && (bit == 5)))
g.FillEllipse(bRed, new Rectangle
(xMax - (iOffsetX + bit * 40), iOffsetY +
(iRow * 40), rWidth, rHeight));
}
else
{
if (!((iRow == 0) && (bit == 5)))
g.DrawEllipse(pRed, new Rectangle
(xMax - (iOffsetX + bit * 40), iOffsetY +
(iRow * 40), rWidth, rHeight));
}
}
}
}
private class boolStruct
{
private bool[] _boolList = new bool[6];
public bool[] boolList
{
get { return _boolList; }
set { _boolList = value; }
}
}
private boolStruct byte2bool(byte b){
boolStruct bArr = new boolStruct();
for (int i = 0; i < 6; i++)
{
bool Temp_Bool = (((b >> i) & 1) == 1);
bArr.boolList[i] = Temp_Bool;
}
return bArr;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.