Introduction
In this Article i'm go to explain how to deploy an An Angular5 app to Firebase and the Firebase library to your application..
What is AngularFire?
- Observable based - Use the power of RxJS, Angular, and Firebase.
- Realtime bindings - Synchronize data in realtime.
- Authentication - Log users in with a variety of providers and monitor authentication state in realtime.
- Offline Data - Store data offline automatically with AngularFirestore.
- ngrx friendly - Integrate with ngrx using AngularFire's action based APIs.
Adding Libraries
So as a first step, let's start with adding libraries to our project by exectuting the following command but before that make sure you have installed latest version of Angular-cli.
$ npm install --save firebase@^4.4.0 angularfire2@^5.0.0-rc.2
Next, in app.module.ts file , add the followings imports statements and add the modules AngularFireModule, AngularFireDatabaseModule, and AngularFireAuthModule to the imports array of the @NgModule decorator:
import { environment } from './../environments/environment';
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { AngularFireModule } from 'angularfire2';
import { AngularFireDatabaseModule } from 'angularfire2/database';
import { AngularFireAuthModule } from 'angularfire2/auth';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { HomeComponent } from './home/home.component';
@NgModule({
declarations: [
AppComponent,
HomeComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
AngularFireModule.initializeApp(environment.firebase),
AngularFireDatabaseModule,
AngularFireAuthModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Using the code
Creating a Firebase Project:
So to create a firebase project , go to https://console.firebase.google.com website and after creating an account and being logged in, click on Go to console , and read the followings steps :
Step 1: Click on "Add Firebase to you web app" icon
Step 2 : Copy the key-value pairs inside the config object and paste it in you project solution in environments/environment.ts like this way
export const environment = {
production: false,
firebase:{
apiKey: "",
authDomain: "example.firebaseapp.com",
databaseURL: "https://example.firebaseio.com",
projectId: "angular5*",
storageBucket: "example.appspot.com",
messagingSenderId: ""
}
};
So that's it , that's all what we need for Firebase settings.
Deploy to Firbase:
It's super simple , just open up your command line and write the following commands:
$npm i -g firebase tools
$firebase login
$firebase init
And then in the firebase.json file , type the following code:
{
"hosting": {
"public": "dist",
"ignore": [
"firebase.json",
"**/.*",
"**/node_modules/**"
],
"rewrites": [
{ "source": "**", "destination": "/index.html" }
]
}
}
$ ng build --prod
$firebase deploy
And you will have lastly the following as mentioned above. So just copy the link and you will get access to your hosted project .
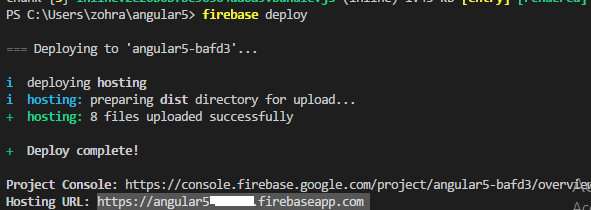
Conclusion
So that's it, if you have any questions don't hesitate to comment out ! Thank you!