Introduction
This article is the second in the series for learning Dart language, the new kid on the block. Dart Team was upgrading language almost at the same pace of .NET Core. With Flutter making its main language for app development, I believe it would be in mainstream soon.
In this article, I would be talking about List
type, which is an amalgamation of normal Array
and List
of C++, similar to System.Collection.Generic.List
data structure of C#.
Background
I am going to discuss the following properties and methods of List
class, this class is defined in package Dart:Core
package, since DART
is opensource, you can check its code in list.dart file. I am going to discuss the following properties provided by List
class. Definition and declaration are taken from the DART website. If you need to understand how to create project, please refer to the first article of series here.
- Task#1 Creation
List(int length)
- Create a list of the given length
List.filled(int length,E fill,{bool growable:false})
- Create a fixed length list of the given length
and initialise the value of each position with fill
argument List.generate(int length, E generator(int index),{bool gowable:true}
) - generate a list of values based on generator
function based on length
argument List.unmodifable(Iterable elements)
- Create an unmodifiable list containing all elements. List.from(Iterable elements,{bool growable:false})
- Create a list containing all the elements. static List.copyRange<T>(List<T> target, int at, List<T> source, [int start, int end])
: copy source
list to target
, starting from at
, you can also specify start
(inclusive) and end
(exclusive) of source list to copy.
- Task#2 Properties
first
- Return first element from list last
- Return last element from list length
- Return length of list reversed
- Reverse the list isEmpty
- Check if the list is empty isNotEmpty
- Check if the list is non-empty runtimeType
- Object type, similar to type in C#
- Task#3 Add/Delete Data
add(E value), addAll(Iterable<E> iterable)
- Add Value/iterable to list asMap()
- Returns an unmodifiable Map of list, key would be index
, and list item as value
fillRange(int start, int end, [ E fillValue ])
/ Iterable<E> getRange(int start, int end)
- Sets the objects in the range start
inclusive to end
exclusive to the given fillValue
/ Returns an Iterable that iterates over the objects in the range start
inclusive to end
exclusive. insert(int index, E element)/ insertAll(int index, Iterable<E> iterable)
- Inserts the object at position index
in this list / Inserts all objects of iterable
at position index
in this list & Inserts all objects of iterable
at position index
in this list. setAll(int index, Iterable<E> iterable) /setRange(int start, int end, Iterable<E> iterable, [ int skipCount = 0 ])
- Copies the objects of iterable
, skipping skipCount
objects first, into the range start
, inclusive, to end
, exclusive, of the list. take(int count)/takeWhile(bool test(E value)
) - Returns a lazy iterable of the count
first elements of this iterable. / Returns a lazy iterable of the leading elements satisfying test
. fold<T>(T initialValue, T combine(T previousValue, E element))
- Reduces a collection to a single value by iteratively combining each element of the collection with an existing value join([String separator = "" ])
- Converts each element to a String and concatenates the string
s remove(Object value)/removeAt(int index)/removeLast()
- Removes the first occurrence of value from this list / Removes the object at position index from this list / Pops and returns the last object in this list. removeRange(int start, int end)
- Removes the objects in the range start inclusive to end exclusive.
- Task#4 Find and where is data
indexOf(E element, [ int start = 0 ])
- Returns the first index of element
in this list. elementAt(int index)
- Returns the indexth element. lastIndexOf(E element, [ int start ])
- Returns the last index of element in this list. any(bool f(E element))
- Checks whether any element of this iterable satisfies test. sublist(int start, [ int end ])
- Returns a new list containing the objects from start inclusive to end exclusive. where(bool test(E element))
- Returns a new lazy Iterable with all elements that satisfy the predicate test. singleWhere(bool test(E element))
- Returns the single element that satisfies test. firstWhere(bool test(E element), { E orElse() })
- Returns the first element that satisfies the given predicate test. lastWhere(bool test(E element), { E orElse() })
- Returns the last element that satisfies the given predicate test. retainWhere(bool test(E element))
- Removes all objects from this list that fail to satisfy test removeWhere(bool test(E element))
- Removes all objects from this list that satisfy test.
Using the Code
I have divided the tutorial in four tasks, I will discuss each task separately.
In this section, I am going to discuss various creation methods provided by DART for creation or initial filling up data in List
, I am covering all 6 methods here. You can check creation.dart file in the attached zip for the same.
void creation()
{
List<int> listOfInt = new List<int>();
List<int> listOfIntWithLength = new List<int>(5);
List<int> listofIntFilledFixed = new List<int>.filled(5, 1);
try{
listofIntFilledFixed.add(5);
}
catch(ex)
{
print(ex);
}
List<int> listofIntFilledGrowable = new List<int>.filled(5, 1,growable:true);
listofIntFilledGrowable.add(5);
List<int> listofIntGenerate = new List<int>.generate(5,(int index)=> index+1);
List<int> listOfIntFrom = new List<int>.from(listofIntGenerate);
List<int> listOfIntunmodifiable = new List<int>.unmodifiable(listofIntGenerate);
try{
listOfIntunmodifiable.add(5);
}
catch(ex)
{
print(ex);
}
List<int> listOfIntWithcopyRange = new List<int>(3);
List.copyRange(listOfIntWithcopyRange, 0, listOfIntunmodifiable,0,3);
}
I have provided relevant comments in code itself to demonstrate various code snippets. Here, we reached the end of Task#1.
In this task, I will demonstrate the use of first
, last
, length
, reverse
, isEmpty
, isNotEmpty
, runtimeType
properties supplied by DART:List
class. The code is written in property.dart,
which is in the attached zip file.
void properties()
{
List<int> listIntProperties = new List.generate(5,(int index)=>index+1,growable: true);
print("First : ${listIntProperties.first}, Last : ${listIntProperties.last}
and number of elements are ${listIntProperties.length}");
listIntProperties.reversed.forEach((int item){ print('item ${item}');});
print("isEmpty : ${listIntProperties.isEmpty}, isNotEmpty : ${listIntProperties.isNotEmpty}
and ObjectTyp ${listIntProperties.runtimeType}");
}
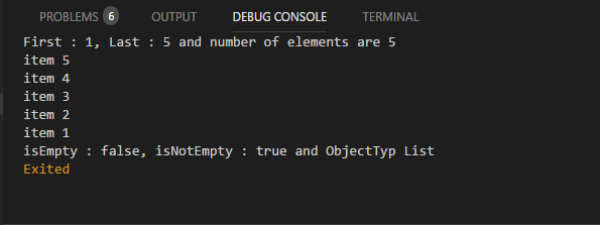
Here we reached end of Task #2.
In this code, I am going to discuss add(E value) , addAll(Iterable<E> iterable), asMap(), fillRange(int start, int end, [ E fillValue ])/ Iterable<E> getRange(int start, int end), insert(int index, E element)/ insertAll(int index, Iterable<E> iterable)
. Look for below code in listadd()
in listadd.dart
.
void listadd()
{
List<int> listIntAdd = new List.generate(5,(int index)=>index+1,growable: true);
List<int> listIntAdd2 = new List.generate(2,(int index)=>index+10,growable: true);
listIntAdd.add(6);
print("After Task 3.1 add (listIntAdd)= " + listIntAdd.join(","));
listIntAdd.addAll(listIntAdd2);
print("After Task 3.1 addAll (listIntAdd) = " + listIntAdd.join(","));
print("Task#3.2 asMap()");
listIntAdd2.asMap().forEach((key,value){ print("key ${key} value is ${value}");});
List<int> listIntfillRange = new List.generate(3,(int index)=>5,growable: true);
listIntfillRange.fillRange(0, 3, 5);
print("After Task 3.3 fillRange (listIntfillRange) = " + listIntfillRange.join(","));
print("After Task 3.3 getRange (listIntAdd) = " + listIntAdd.getRange(3,5).join(","));
listIntfillRange.insert(2,7);
print("After Task 3.4 insert (listIntfillRange) = " + listIntfillRange.join(","));
listIntfillRange.insertAll(2, listIntAdd2);
print("After Task 3.4 insertAll (listIntfillRange) with (listIntAdd2) = " + listIntfillRange.join(","));
}

For second code demonstration, I would be discussing setAll(int index, Iterable<E> iterable) /setRange(int start, int end, Iterable<E> iterable, [ int skipCount = 0 ]) ,take(int count)/takeWhile(bool test(E value)) ,fold<T>(T initialValue, T combine(T previousValue, E element)) ,remove(Object value)/removeAt(int index)/removeLast() ,removeRange(int start, int end),
these are coded in listadd2()
of listadd.dart
.
void listadd2()
{
List<int> listIntAdd = new List<int>.generate(5,(int index)=>index+1,growable: true);
List<int> listIntAdd2 = new List<int>.generate(5,(int index)=>0,growable: true);
print("Task 3.5 Before setAll (listIntAdd2) = " + listIntAdd2.join(","));
listIntAdd2.setAll(0, listIntAdd);
print("Task 3.5 After setAll (listIntAdd2) = " + listIntAdd2.join(","));
print("Task 3.6 take (listIntAdd2) = " + listIntAdd2.take(3).join(","));
print("Task 3.6 takeWhile (smaller than 4) (listIntAdd2) =
" + listIntAdd2.takeWhile((int item) => item <= 3 ).join(","));
print("Task 3.7 fold (listIntAdd2) Initial Val= " + listIntAdd2.join(","));
print("Task 3.7 fold (listIntAdd2) = " + listIntAdd2.fold(0, (prev,element)=>prev+element).toString());
print("Task 3.9 (listIntAdd2) Initial Val= " + listIntAdd2.join(","));
listIntAdd2.remove(5);
print("Task 3.9 remove (listIntAdd2) = " + listIntAdd2.join(","));
listIntAdd2.removeAt(2);
print("Task 3.9 removeAt (listIntAdd2) = " + listIntAdd2.join(","));
listIntAdd2.removeLast();
print("Task 3.9 removeAt (listIntAdd2) = " + listIntAdd2.join(","));
print("Task 3.10 (listIntAdd) Initial Val= " + listIntAdd.join(","));
listIntAdd.removeRange(0, 2);
print("Task 3.10 removeAt (listIntAdd) = " + listIntAdd.join(","));
}

Here, we reached the end of Task #3.
In the following code, I am going to discuss indexOf(E element, [ int start = 0 ]),elementAt(int index),lastIndexOf(E element, [ int start ]),any(bool f(E element)),sublist(int start, [ int end ]),where(bool test(E element))
. The example is present in listwhere()
function of where.dart
.
listwhere(){
List<int> listIntWhere= new List<int>.generate(8,(int index)=>index+1,growable: true);
print("Task 4.1 indexOf (listIntWhere) InitialVal= " + listIntWhere.join(","));
print("Task 4.1 indexOf (listIntWhere)= " + listIntWhere.indexOf(6).toString());
print("Task 4.2 elementAt (listIntWhere) InitialVal= " + listIntWhere.join(","));
print("Task 4.2 elementAt (listIntWhere)= " + listIntWhere.elementAt(5).toString());
List<int> listDuplicate = new List.generate(6, (int x)=> x<3?x:x-3);
print("Task 4.3 lastIndexOf (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.3 lastIndexOf (listDuplicate)= " + listDuplicate.lastIndexOf(1).toString());
print("Task 4.4 any (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.4 any (listDuplicate)= " + listDuplicate.any((int item)=>item==2).toString());
print("Task 4.5 sublist (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.5 sublist (listDuplicate)= " + listDuplicate.sublist(3,6).join(","));
print("Task 4.6 where (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.6 where (listDuplicate)= " + listDuplicate.where((int item)=> item%2==0).join(","));
}

Last but not the least, in the following code, I am going to discuss singleWhere(bool test(E element)) ,firstWhere(bool test(E element), { E orElse() }),lastWhere(bool test(E element), { E orElse() }) ,retainWhere(bool test(E element)),removeWhere(bool test(E element))
. The example is present in listwhere2()
function of where.dart
.
listwhere2(){
List<int> listIntWhere= new List<int>.generate(8,(int index)=>index+1,growable: true);
print("Task 4.7 singleWhere (listIntWhere) InitialVal= " + listIntWhere.join(","));
print("Task 4.7 after singleWhere (listIntWhere)= " +
listIntWhere.singleWhere((int item)=>item==2).toString());
try{
listIntWhere.singleWhere((int item)=>item==9);
}
catch(ex)
{
print("Task 4.7 after singleWhere (listIntWhere) (from catch) notFound = " + ex.toString());
}
List<int> listDuplicate = new List.generate(8, (int x)=> x<4?x:x-4);
print("Task 4.8 firstWhere (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.8 after firstWhere (listDuplicate)= " +
listDuplicate.firstWhere((int item)=>item==2).toString());
print("Task 4.8 after firstWhere (listDuplicate) notFound (return -1)= " +
listDuplicate.firstWhere((int item)=>item==10,orElse: ()=>-1).toString());
print("Task 4.9 lastWhere (listDuplicate) InitialVal= " + listDuplicate.join(","));
print("Task 4.9 after lastWhere (listDuplicate)= " +
listDuplicate.lastWhere((int item)=>item==2).toString());
print("Task 4.9 after lastWhere (listDuplicate) notFound (return -1)= " +
listDuplicate.lastWhere((int item)=>item==10,orElse: ()=>-1).toString());
print("Task 4.10 retainWhere (listDuplicate) InitialVal= " + listDuplicate.join(","));
listDuplicate.retainWhere((int x)=> x%2==0);
print("Task 4.10 after retainWhere (listDuplicate) = " + listDuplicate.join(","));
print("Task 4.11 removeWhere (listIntWhere) InitialVal= " + listIntWhere.join(","));
listIntWhere.removeWhere((int x)=> x%2!=0);
print("Task 4.11 after removeWhere (listIntWhere) = " + listIntWhere.join(","));
}

Here, we reached the end of Task #4.
Points of Interest
Flutter Tutorial
- Flutter Getting Started: Tutorial #1
- Flutter Getting Started: Tutorial 2 - StateFulWidget
DART Series
- DART2 Prima Plus - Tutorial 1
History
- 08-July-2018: First version