Day 90! Wow it’s been a long time, at the rate I’m making these posts, I really shouldn’t call it day 90 and more like post 90. I don’t really feel like changing conventions, so I’m going to stick with the day notion.
What’s the plan for today? Well at this point, we have the score multiplier power-up already, now we’re going to use it.
The procedure isn’t going to be too different from what we already have done for the magnet power-up. Today, we’re going to:
- Create the code that will pick up our 2X game object
- Add it in our game
At this point, there should be nothing new, but for the sake of completeness, we’re going through this again.
Step 1: Picking up our Score multiplier Power-up
Step 1.1: Modify PlaneCollider to detect our new power-up
At this point, we need to modify our PlaneCollider script to do something when we run into our power-up.
Here’s what the script looks like:
using UnityEngine;
public class PlaneCollider : MonoBehaviour
{
public GameObject PlaneObject;
public GameObject Explosion;
public AudioClip MagnetSFX;
public GameObject MagnetParticleEffect;
private SoundManager _soundManager;
private GameObject _currentParticleEffect;
void Start()
{
_soundManager = GetComponent<SoundManager>();
}
void OnTriggerEnter(Collider other)
{
print(other + " name " + other.name);
switch (other.tag) {
case "Coin":
CoinCollision(other);
break;
case "Magnet":
MagnetCollision(other);
break;
case "Score":
ScoreColllision(other);
break;
default:
CheckUnTaggedCollision(other);
break;
}
}
public void StopParticleEffect()
{
if (_currentParticleEffect != null)
{
ParticleSystem particleSystem = _currentParticleEffect.GetComponent<ParticleSystem>();
particleSystem.Stop();
Destroy(_currentParticleEffect);
_currentParticleEffect = null;
}
}
private void CoinCollision(Collider other) {
Coin coin = other.GetComponent<Coin>();
coin.Collect();
GameManager.Instance.CollectCoin();
}
private void MagnetCollision(Collider other)
{
Debug.Log("magnet collision hit");
PlayerManager.Instance.AddPowerUp(PlayerManager.PowerUpType.Magnet);
Magnet magnet = other.GetComponent<Magnet>();
_soundManager.PlayBackgroundClip(MagnetSFX);
StopParticleEffect();
_currentParticleEffect = Instantiate(MagnetParticleEffect, PlaneObject.transform);
ParticleSystem particleSystem = _currentParticleEffect.GetComponent<ParticleSystem>();
particleSystem.Play();
magnet.Collect();
}
private void ScoreColllision(Collider other)
{
Debug.Log("score collision hit");
PlayerManager.Instance.AddPowerUp(PlayerManager.PowerUpType.Score);
ScoreMultiplier score = other.GetComponent<ScoreMultiplier>();
score.Collect();
}
private void CheckUnTaggedCollision(Collider other) {
if (other.name.Contains("Cube")) {
EnemyCollision();
}
}
private void EnemyCollision()
{
Instantiate(Explosion, PlaneObject.transform.position, Quaternion.identity);
Destroy(PlaneObject);
PlayerManager.Instance.GameOver();
GameUIManager.Instance.GameOver(gameObject);
CameraManager.Instance.GameOver();
}
}
Walking through the code
At this point, there shouldn’t be anythign too surprising about what’s going on here.
- In our
OnTriggerEnter
if we run into something that has the tag Score
then we know we collided against our Score Multiplier. At this point, we’ll call ScoreCollision()
- In
ScoreCollision
we will add the Score power-up (to be added) to our list of power-ups, we’ll grab the ScoreMultiplier script that we will write next and call it’s Collect
function to deal with some of the logic to pick up the game object
Setting up the script
To get this script working, we need to make some changes to our 2X game object.
- Currently we have a game object called 2X that has a child game object called 2X, rename the child 2X to be 2X Model
- In 2X Model we need to make some adjustments to make it the same size as the magnet. Change Rotation to be (0, 180, 0). Change Scale to be (0.2, 0.2, 0.2)
- Add a new tag to 2X Model and call it Score
- Add a Box Collider to 2X Model. Set the Center to be (-1, 7, 8.5) and Size to be (24, 15, 1)
- Finally, with all of these changes, drag and drop 2X back into our Prefab folder to create a prefab of our object. I prematurely made a prefab in the last post, so get rid of that prefab and call our new prefab
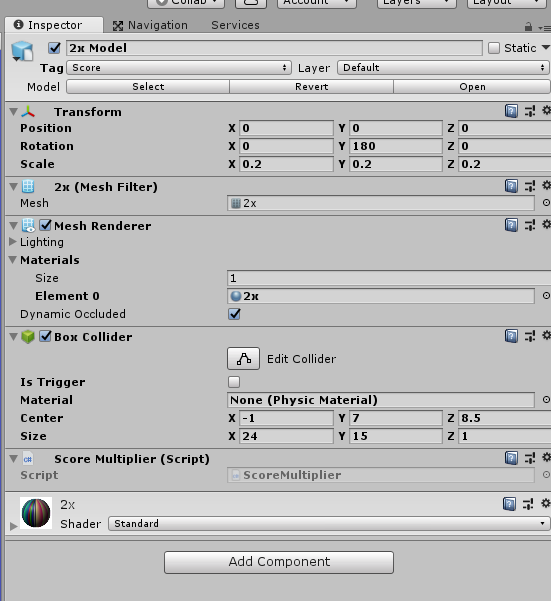
Step 1.2: Create the ScoreMultiplier script
Currently, our compiler will complain about ScoreMultiplier
not being a script and that makes sense, we haven’t made the script yet!
We’re going to create this script.
- In 2X Model, add a new script and call it
ScoreMultiplier
Here’s what ScoreMultiplier
looks like:
using UnityEngine;
using System.Collections;
public class ScoreMultiplier : MonoBehaviour
{
public void Collect()
{
StartCoroutine(RemoveGameObject());
}
private IEnumerator RemoveGameObject()
{
yield return new WaitForSeconds(0.1f);
Destroy(transform.parent.gameObject);
}
}
Walking through the code
If you’re wondering if this looks exactly like the Magnet script, you’d be absolutely correct! We just copy and pasted the Magnet Script and renamed it ScoreMultiplier.
- PlaneCollider calls
Collect()
which will start a coroutine RemoveGameObject()
which will get rid of our game object’s parent in 0.1 seconds. We get rid of the parent, because the script is placed in 2X Model and when we get rid of it, we also want to get rid of its container
Step 1.3: Add Score to the PowerUpType Enum
The final thing that needs to be added to make our game run again is to add a new enum value to our PowerUpType
enum which is located in PlayerManager
.
I’ll omit most of the code, but this is what we want to change in PlayerManager
:
public class PlayerManager : MonoBehaviour
{
…
public enum PowerUpType { Magnet, Score }
…
}
For now, we’re not going to do anythign with this enum, however in the upcoming articles, we’ll be adding those functionalities in.
Now with this, our game will start running.
Step 2: Adding the 2X Power-Up into the Generated Paths
Step 2.1: Adding the Score Multiplier power-up to the ItemLoaderManager script
We have a new power-up now it’s time to add it into our paths. You might recall that for the Magnet Power-Up, we instantiate the power-up into our game from the ItemGenerator script that each one of our path object contains, however inside the ItemGenerator script, we actually pull our list of power-ups to choose from our ItemLoaderManager
All we need to do to add our new score-multiplier power-up into the game is to add it to the ItemLoaderManager
and our existing will already be able to handle the rest. Neat!
- In the game hierarchy, find Manager and look for ItemLoaderManager
- In the Power Ups slot, change the array size to be 2 and for Element 1 add our 2x prefab to be our 2nd power-up.
With this, if we play the game now, our Score Multiplier will randomly show up!

End of Day 90
And that’s it! A lot of the work we must do is some tedious setup code, however luckily for us, we already have most of the existing infrastructure to leverage. As a result, today’s work was very straightforward!
Today we:
- Wrote code to allow us to pick up our new score multiplier power-up
- Have the power-up randomly spawn the power-up in our game
In our next post, we’re going to start implementing the score-multiplier effect, I don’t expect it to be too long or hard, so we might also start integrating the rest of the work needed for our score multiplier into the game. Find out next time!