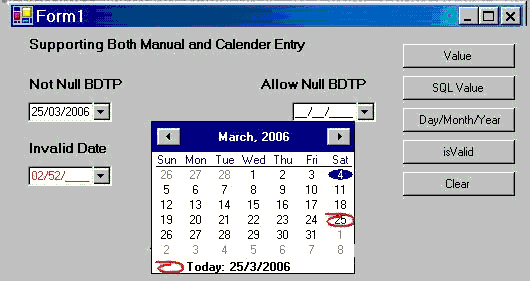
Background
In a database application, date handling is a bit difficult as compared to validating other data types, and getting input by keyboard requires some extra effort. The common solution to this problem is the DateTimePicker
control provided by Microsoft. Unfortunately, DateTimePicker
is not the best suited for fast data entry via keyboard that is always required in database driven applications. I get several complaints from end users that they don’t want to use the mouse for date selection, or that when entering date in a DateTimePicker
via keyboard, it requires extra keys to be pressed from moving the cursor from one day part to next, and so on. This is the motivation behind the development of a new DateTimePicker, which will enable users to enter dates faster and in alternate ways either by using the keyboard or by using the mouse.
Introduction
What I have done is, created a textbox masked control to input date via the keyboard, and also attached a DateTimePicker
control to input date via mouse. It also has the functionality to validate user input if the date is not valid, and then the text color is displayed in red. Also, set its property “IsValid
” to false
. To validate date, what I have done is a trick: just set the value of the DateTimePicker
with the value entered in the TextBox
, and the DateTimePicker
will generate an exception for an invalid date value and the color will change to red.
Using the Control
It is quite easy to use like other controls. Just add to your toolbox, and drop it on the form you want to use. I will give here an overview of the properties that can be used during design time and at run time.
I have tried to expose properties just like in the DateTimePicker
, so if you are going to replace DateTimePicker
from your existing project with this one, you don’t have to change your code.
Properties
Value
Returns the value in the form of date; same as in DateTimePicker
.
Day
Same as DateTimePicker
’s ‘Value.Day
’, but you will get one step in advance, simply ‘Control1.Day
’.
Month
Same as in DateTimePicker
’s ‘Value.Month
’, but you will get one step in advance, simply ‘Control1.Month
’.
Year
Same as in DateTimePicker
’s ‘Value.Year
’, but you will get one step in advance, simply ‘Control1.Year
’.
SQLValue
The specific property required when using the value of DateTimePicker
with SQL Server, because SQL Server requires a date format of “yyyy\MM\dd” to work fine. This property returns the formatted date.
DateFormat
Specify the date format you want to use; currently, there are three supported formats: DMY, MDY, YDM.
IsValid
A read-only property that returns the state of the control. This property can be used for validating the form input before submitting back to the DBMS.
AllowNull
This property is used to replace the checkbox that is displayed with the default DateTimePicker
, that often creates a problem whenever you try to check or uncheck it in code. So for now, when we need to have a null-able date column, this property will be used either to allow a null
or not. It will return null
if the textbox is empty, which means the user doesn’t want to enter the date.
Events
Methods
Clear
It can be used to clear the date if AllowNull
is set to true
; then it will set the value property to null
and clear the text of the control; otherwise, sets the control text to the current date and value accordingly.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.