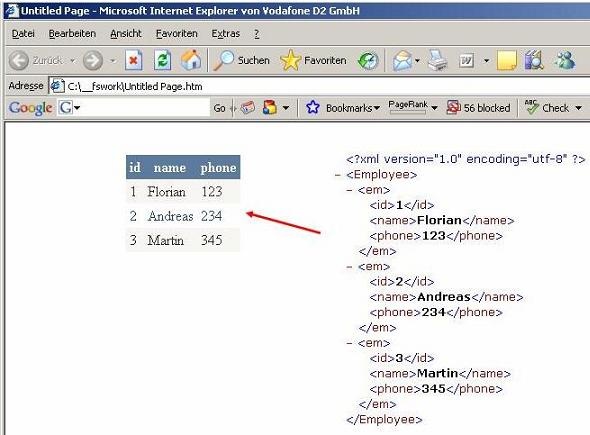
Introduction
Sometimes you want to use an XML file rather than a database to store your information. Maybe you don't have a database at your hosting account or you have mostly static data. This article explains how to bind an XML file to a GridView
in four lines of code.
The XML File
First, create a new XML file and populate it with some data. Here is an example:
="1.0"="utf-8"
<Employee>
<em>
<id>1</id>
<name>Florian</name>
<phone>123</phone>
</em>
<em>
<id>2</id>
<name>Andreas</name>
<phone>234</phone>
</em>
<em>
<id>3</id>
<name>Martin</name>
<phone>345</phone>
</em>
</Employee>
Bind the XML File to a GridView
Drag a GridView
control on to your web form. Next, you need some code to bind the data from the XML file to your GridView
.
Imports System.Data
Partial Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
Dim oDs As New DataSet
oDs.ReadXml(Request.PhysicalApplicationPath + "XMLFile.xml")
GridView1.DataSource = oDs
GridView1.DataBind()
End Sub
End Class
In the Page_Load
event, we create a new DataSet
and use the method ReadXml
to get the data from the XML file. Then we bind the DataSet
to the GridView
.
Points of Interest
In order to avoid "hard coding" the path to the XML file , I use Request.PhysicalApplicationPath
to specify the location of the file.