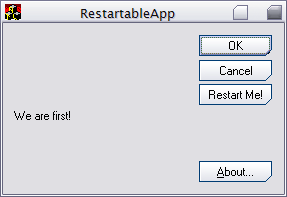 |
Step 1. Application Before Restart |
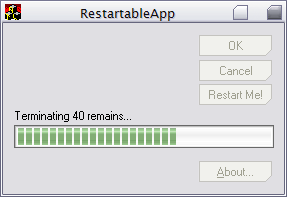 |
Step 2. Application While Restarting |
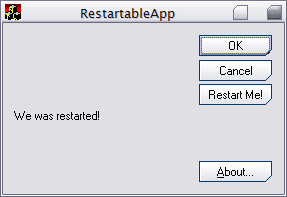 |
Step 3. Application After Restart |
Introduction
In some cases, your application must be restarted to apply some changes. For example, you might want to restart an application after downloading newer versions of files or changing the UI language of the application. For users, it is very convenient not to have to restart an application manually. The best way is to ask the user if the application has to be restarted then or later.
In this article, I describe an easy way to complete this task with a few lines of code.
Using the code
Using the restart code is very simple.
First, include "RestartAPI.h" in your "stdafx.h", and add "RestartAPI.cpp" in your VC project.
The second step is modifying your main
or WinMain
function like shown below. Another way is to add the restart initialization code to your application start point and add the restart finishing code to the exit point, such as PreMessageLoop
/PostMessageLoop
in a WTL application. Here is an example of the modified WinMain
function.
int WINAPI _tWinMain(HINSTANCE hInstance,
HINSTANCE ,
LPTSTR lpstrCmdLine, int nCmdShow)
{
if (RA_CheckForRestartProcessStart())
RA_WaitForPreviousProcessFinish();
RA_DoRestartProcessFinish();
return 0;
}
The third step is to determine the point where you want to initialize the restarting of an application. In the attached sample, this point is Click handler of the button "Restart Me!". For restarting the application, you must call the RA_ActivateRestartProcess
function and terminate your application.
LRESULT CMainDlg::OnBnClickedRestart(WORD ,
WORD , HWND , BOOL& )
{
if (!RA_ActivateRestartProcess())
{
::MessageBox(NULL, _T("Something Wrong"),
_T("Restart App"),
MB_OK|MB_ICONEXCLAMATION);
return 0;
}
CloseDialog(IDC_RESTART);
return 0;
}
Short functions reference
Macro
Command line switch for restarting the application:
#define RA_CMDLINE_RESTART_PROCESS TEXT("--Restart")
You can redefine the command line switch for your purposes. Restarted application is started with this switch:
#define RA_MUTEX_OTHER_RESTARTING TEXT("YOUR_RESTART-MUTEX_NAME")
Mutex unique name
Named mutex is used for waiting termination of the first instance of a process. The mutex name must be unique. For that, you can use GUIDs to define mutex namea like this:
#define RA_MUTEX_OTHER_RESTARTING
TEXT("APPRESTART-E476AE82-AA92-11DA-ACE4-006098EFC07C")
You can redefine a mutex name for your purposes.
Functions
BOOL RA_CheckProcessWasRestarted();
Returns TRUE
if process was restarted.
BOOL RA_CheckForRestartProcessStart();
Checks the process command line for restart switch. Call this function to check that it is a restarted instance.
BOOL RA_WaitForPreviousProcessFinish();
Wait till the previous instance of the process is finished.
BOOL RA_DoRestartProcessFinish();
Call it when a process finishes.
BOOL RA_ActivateRestartProcess();
Call this function when you need to restart an application. Start another copy of the process with the command line RA_CMDLINE_RESTART_PROCESS
. After calling RA_ActivateRestartProcess
, you must close an active instance of your application.
Downloads
Demo project
The demo project includes a sample WTL dialog application that demonstrates how to use the restarting API. From the screenshots, you can see some stages of the application at work.
The first screen shows the application starting normally. In the second screen, the user clicked the "Restart Me!" button and you see the pseudo-termination progress. If you run the Task Manager at that moment, you'll see two instances of RestartableApp.exe. In the last screen, the application is in the normal state after it was restarted.
Source files
Source files include "RestartAPI.h" and "RestartAPI.cpp" which can be used separately in your Win32 application.
History