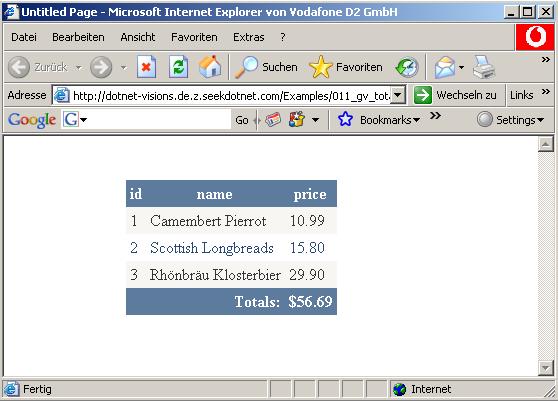
Introduction
This article explains how to display a totals line in a GridView
footer. First, bind an XML file to the GridView
. Here is the code for this task:
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
If Page.IsPostBack = False Then
Dim oDs As New DataSet
oDs.ReadXml(Request.PhysicalApplicationPath + "XMLFile.xml")
GridView1.DataSource = oDs
GridView1.DataBind()
End If
End Sub
And here is the content of the XML file. We want to add the price of each product to a variable called dTotal
and then display this value in the GridView
footer.
="1.0"="utf-8"
<products>
<product>
<id>1</id>
<name>Camembert Pierrot</name>
<price>10,99</price>
</product>
<product>
<id>2</id>
<name>Scottish Longbreads</name>
<price>15,80</price>
</product>
<product>
<id>3</id>
<name>Rhönbräu Klosterbier</name>
<price>29,90</price>
</product>
</products>
The Code
Insert an event handler for the RowCreated
event into your code-behind file. Then, check the RowType
of each new row. In the case of DataControlRowType.DataRow
, add the value of "price" to the variable dTotal
.
In the case of DataControlRowType.Footer
, assign the value of dTotal
to the third cell of the GridView
footer.
Dim dTotal As Decimal = 0
Protected Sub GridView1_RowDataBound(ByVal sender As Object, _
ByVal e As System.Web.UI.WebControls.GridViewRowEventArgs) _
Handles GridView1.RowDataBound
If e.Row.RowType = DataControlRowType.DataRow Then
dTotal += Convert.ToDecimal(DataBinder.Eval(e.Row.DataItem, "price"))
End If
If e.Row.RowType = DataControlRowType.Footer Then
e.Row.Cells(1).Text = "Totals:"
e.Row.Cells(2).Text = dTotal.ToString("c")
e.Row.Cells(1).HorizontalAlign = HorizontalAlign.Right
e.Row.Cells(2).HorizontalAlign = HorizontalAlign.Right
e.Row.Font.Bold = True
End If
End Sub
To display the footer, you have to set the attribute ShowFooter="True"
.