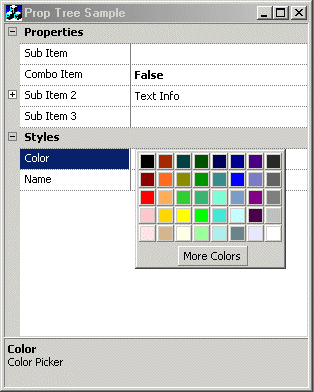
Introduction
The CPropTree
class implements a tree control that is similar to the property
view seen in Visual Studio .Net. The source project compiles to a DLL so you can easily incorporate
it into your project. You can check my web page for more information
on the control.
The control has two window areas:
- Tree Control
- Descriptive area
The tree control section functions just a like a normal tree control with an addition of a
splitter bar separating the tree item properties. Tree item properties are inherited from
the CPropTreeItem
class. These items can be edit controls, static text, dropdown
combo list, or any type of control you wish to create. Items on the root level of the tree
control do not have editable properties. They are used as section headers.
Tree items can contain checkboxes. By default properties that are editable (non-readonly), are displayed in
bold lettering. Read only or non-editable items are displayed in a normal font.
The descriptive area displays the selected item's text and any informational text. This section can
be displayed or hidden by calling CPropTree::ShowInfoText()
.
Implementation
To use the control, create an instance of the class in your dialog or view class header. In
the OnInitDialog()
or OnCreate()
initialize the control:
DWORD dwStyle;
CRect rc;
dwStyle = WS_CHILD|WS_VISIBLE|PTS_NOTIFY;
GetClientRect(rc);
m_Tree.Create(dwStyle, rc, this, IDC_PROPERTYTREE);
To add items to the control, dynamically create objects inherited by the CPropTreeItem
class and call the CPropTree::InsertItem()
method.
CPropTreeItem* pItem1;
pItem1 = m_Tree.InsertItem(new CPropTreeItem());
pItem1->SetLabelText(_T("Properties"));
pItem1->SetInfoText(_T("This is a root level item"));
CPropTreeItem* pItem2;
pItem2 = m_Tree.InsertItem(new CPropTreeItemEdit(), pItem1);
pItem2->SetLabelText(_T("EditItem"));
pItem2->SetInfoText(_T("This is a child item of Properties, with an edit control"));
pItem2->SetItemValue((LPARAM)_T("This is some default text for the edit control"));
Advanced Features
Notification Messages
The control uses WM_NOTIFY
notification messages to signal events. Add a ON_NOTIFY
message handler to the parent window to process notifications. The control supports
most of the common NM_ codes with addition of the following CPropTree
specific WM_NOTIFY
codes.
PTN_INSERTITEM
when an item is insertedPTN_DELETEITEM
when an item is about to be deletedPTN_DELETEALLITEMS
when a call is made to delete all itemsPTN_ITEMCHANGED
when an item's property has been modifiedPTN_SELCHANGE
when the current selection changedPTN_ITEMEXPANDING
when an item is about to expand or closePTN_COLUMNCLICK
when the mouse clicks on the splitter barPTN_PROPCLICK
when the mouse clicks on an item's property areaPTN_CHECKCLICK
when the mouse clicks on an item's checkbox
PTN_
notification messages returns the
NMPROPTREE
structure. You can use this structure along with the
CPropTreeItem::SetCtrlID()
method to determine the tree item that sent an event.
[...]
ON_NOTIFY(PTN_ITEMCHANGED, IDC_PROPERTYTREE, OnItemChanged)
END_MESSAGE_MAP()
void CMyDialog::OnItemChanged(NMHDR* pNotifyStruct, LRESULT* plResult)
{
LPNMPROPTREE pNMPropTree = (LPNMPROPTREE)pNotifyStruct;
if (pNMPropTree->pItem)
{
}
*plResult = 0;
}
Custom Property Items
The library already has some default derived CPropTreeItem
classes ready
to use. Such as an edit control CPropTreeItemEdit
, color
picker CPropTreeItemColor
, dropdown combobox CPropTreeItemCombo
and
static text CPropTreeItemStatic
. To create your own custom property items:
- Create your own class inherited by
CPropTreeItem
. This class usually will have
multiple inheritance depending on what type of item you want to create.
class CMyCustomEditPropItem : public CEdit, public CPropTreeItem
{
public:
CMyCustomEditPropItem();
virtual ~CMyCustomEditPropItem();
[...]
};
class CMyCustomComboBoxPropItem : public CComboBox, public CPropTreeItem
{
public:
CMyCustomComboBoxPropItem();
virtual ~CMyCustomComboBoxPropItem();
[...]
};
- Override the
CPropTreeItem
virtual methods to implement your property item.
- Dynamically create an instance of your class and insert it into a
CPropTree
control.
The methods most often overriden from CPropTreeItem
are:
CPropTreeItem::OnActivate()
. Called when the property area is clicked by the mouse
or the enter key has been pressed on the selected item. The OnActivate()
method is where you
show the property item's window if it has one (such as an edit control or a popup menu).CPropTreeItem::OnCommit()
. Called when data has been commited. In this method you would extract
the changed data and hide the property's item's window. OnCommit()
gets called when CommitChanges()
is called. A derived CPropTreeItem
class would call CommitChanges()
during a loss of the input
focus or if the "Enter" key is press as in a edit control.
CPropTreeItem::DrawAttribute()
. CPropTree
calls DrawAttribute()
when
the property item needs to be displayed. Drawing is done directly on the display context of the PropTree control.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.