Introduction
In this article, we will see how we can make use of the jQuery library to make AJAX calls in ASP.NET with the help of HTTPHandlers
. We will also see the use of JSON (JavaScript Object Notation) which is a format describing name/value pairs in a structured manner.
Asynchronous JavaScript and XML (AJAX) is a method which we can use to exchange data with the server without reloading the complete page.
jQuery
is a JavaScript library which makes it easier to manipulate and navigate DOM elements in a web page. It also helps in implementing AJAX in rapid web application development. Currently, we have Intellisense support for jQuery in Visual Studio 2008/2010.
HTTPHandler
is a process which returns data when its URL is called. The returned data can be anything from a simple string
to data formats like JSON or XML.
References: jQuery, MSDN
Prerequisite
Download the latest version of jQuery from the jQuery site (http://jquery.com/). In Visual Studio 2010, it is added by default when a new web application is created.
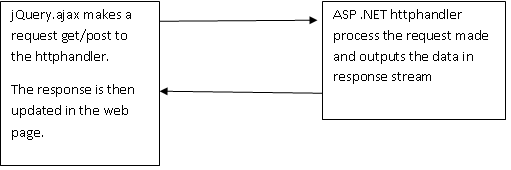
Flow Diagram
Example: Making a Simple AJAX Call
In this example, we will make a simple AJAX call with the help of the jQuery.ajax
API to an HTTPHandler
. The response from the HTTPHandler
will be the JSON response which is then updated in the web page. JavaScript Object notation (JSON) is a format of key/value pairs to store data. It’s easy to consume in JavaScript and compact than XML. So let’s start with creating a handler page.
public void ProcessRequest (HttpContext context) {
string method = context.Request.QueryString["MethodName"].ToString();
context.Response.ContentType = "text/json";
switch (method)
{
case "GetData" :
context.Response.Write(GetData());
break;
}
}
protected string GetData()
{
return (@"{""FirstName"":""Ravi"", ""LastName"":""Baghel"",
""Blog"":""ravibaghel.wordpress.com""}");
}
As you can see, we are simply putting a response of JSON type with sample data. In order to demonstrate passing a parameter to a handler, I have used the querystring
methodname
which will simply switch to the method you want to execute.
Now, we need to make a call to the handler.
<head runat=""server"">
<title>jQuery</title>
<script src="Scripts/jquery-1.5.min.js" type="text/javascript"></script>
<script type="text/javascript" >
jQuery(function() {
$(‘#btnClick’).click(function(){
jQuery.ajax({
type : "GET",
url : "Data.ashx",
data : "MethodName=GetData",
success : function(data){
$(‘#display’).html("<h1> Hi, " + data.FirstName + " " +
data.LastName + " your Blog Address is http://" +
data.Blog + "</h1>");
}
});
});
});
</script>
</head>
<body>
<form id="form1″ runat=""server"">
<input id="btnClick" type="button" value="Ajax Call" />
<div id="display">
</div>
</form>
</body>
We first need to add a reference to the jQuery library which we downloaded as:
<script src="Scripts/jquery-1.5.min.js" type="text/javascript"></script>
If you look at the jQuery.ajax
syntax, you can see different parameters as:
Type
that describes whether the request is GET
or POST
URL
of the HTTPHandler
Data
specifying the querystring
Success
defining a function which manipulates the response from the handler
Download the latest version of the project here.