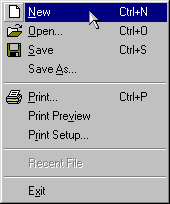
Introduction
This article describes a method of adding bitmaps to your menus. The method
is very easy to use, for example adding all the bitmaps on a tool bar
is accomplished by a single function call. The demo project includes a class
called BitmapMenu
that can be used to add bitmap menus to your
own projects. This class is made up of a small amount of source code, making
it easy to understand and maintain.
There are two good related articles on the code
project about adding bitmaps to menus. They are:
These are both excellent articles with lots of good accompanying code.
Brent Corkum's code in particular produces nice looking bitmaps, and it has
been extensively tested and updated. So of course you are thinking:
"Why do I need to bother with your article?"
The main disadvantage of the Corkum method is the size of the code.
Something as simple as placing bitmaps on menus requires a significant amount
of code which must be maintained by you (or you have to hope that Mr. Corkum
is nice enough to keep updating his classes). For this reason I chose to
use the much more compact Denisov method. Nikolay Denisov's article addresses
much more than putting bitmaps on menus, and the bitmap code is intertwined with
his other code, so it is difficult to use independently. Therefore, I developed
a class based on the Denisov method that is modular. The result is that both my
method and the Corkum method are easy to setup, but there is a significant
difference in the resulting amount of source code. The approximate lines of
source code required by the two methods are:
- Corkum method: 2,350 lines
- This method: 250 lines
Unlike Corkum's work, this code has not been well tested. It has only been tested under Windows 98, and will not work under Windows 95/NT.
To use this code download the demo project. The demo has a second tool bar which
demonstrates two of the class member functions: AddToolBar
and
RemoveToolBar
. To add bitmap menus to your own project follow
these 5 steps:
-
Add the following files to your project:
BitmapMenu.cpp, BitmapMenu.h, winuser2.h
-
In
MainFrm.h
- Add this line to the top of the file:
#include "BitmapMenu.h"
-
In
MainFrm.h
- Inherit your main frame window from
BitmapMenu
rather than from CFrameWnd
or CMDIFrameWnd
.
class CMainFrame : public BitmapMenu<CFrameWnd>
-
In
MainFrm.cpp
- Add three message handlers between
BEGIN_MESSAGE_MAP
and END_MESSAGE_MAP
.
ON_WM_INITMENUPOPUP()
ON_WM_MEASUREITEM()
ON_WM_DRAWITEM()
-
In
MainFrm.cpp
- At the end of the CMainFrame::OnCreate()
function add each tool bar that you want to appear on the menu:
AddToolBar(&m_wndToolBar);
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.