Well, achieving a Twitter page like functionality was never the intention, but this simple example will introduce you to the element binding concept in Silverlight. In Element Binding, we can bind a control property to another control property, that too declaratively inside XAML.
In this post, we will emulate the Twitter feature where the number of characters entered by the user will get displayed while the user enters text in the text box, that too without a single line in the code-behind.
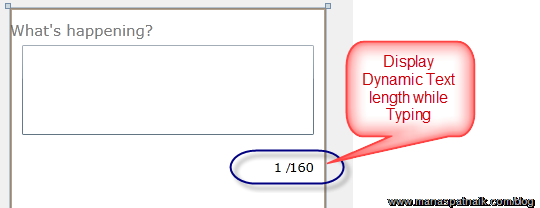
Here I have added two TextBlock
s to hold the max length and to hold the count of characters. We want the TextBlock
Text
property to show the Textbox.Length
property. We will bind the TextBlock
’s Text
to the Length
property of the TextBox
.

The code to bind the element is as follows:
<TextBlock Height="23″ Margin="0,150,44,0″ Name="tbRem" VerticalAlignment="Top"
FontSize="13″ TextAlignment="Right" HorizontalAlignment="Right" Width="46″
Text="{Binding Path=Text.Length,ElementName=txtMsg}" />
In the above code, the third line is the key where we assign the binding to the Text
property; remember the dependency property?

The XAML source code, based on the above fundamentals, achieves the Twitter messaging like functionality:
<UserControl x:Class="TwitterLikeMessege.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008″
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006″
mc:Ignorable="d" d:DesignHeight="300″ d:DesignWidth="400″
xmlns:sdk="http://schemas.microsoft.com/winfx/2006/xaml/presentation/sdk">
<Grid x:Name="LayoutRoot" Background="White">
<sdk:Label Height="30″ HorizontalAlignment="Left" Margin="0,12,0,0″
Name="lblCaption" VerticalAlignment="Top" Width="237″
Content="What’s happening?" FontSize="15″ Foreground="#82000000″ />
<TextBlock Height="23″ Margin="0,150,44,0″ Name="tbRem" VerticalAlignment="Top"
FontSize="13″ TextAlignment="Right" HorizontalAlignment="Right" Width="46″
Text="{Binding Path=Text.Length,ElementName=txtMsg}" />
<TextBlock Height="23″ Margin="0,150,11,0″ Name="tbMaxLen" VerticalAlignment="Top"
Width="26″ TextAlignment="Right" FontSize="13″ HorizontalAlignment="Right"
Text="{Binding Path=MaxLength,ElementName=txtMsg}"/>
<TextBox Height="90″ Margin="12,37,12,0″ Name="txtMsg"
VerticalAlignment="Top" MaxLength="160″ />
<sdk:Label HorizontalAlignment="Right" Margin="0,152,32,0″
Name="lblSlash" Width="25″ Content=" / " Height="28″ VerticalAlignment="Top" />
</Grid>
</UserControl>