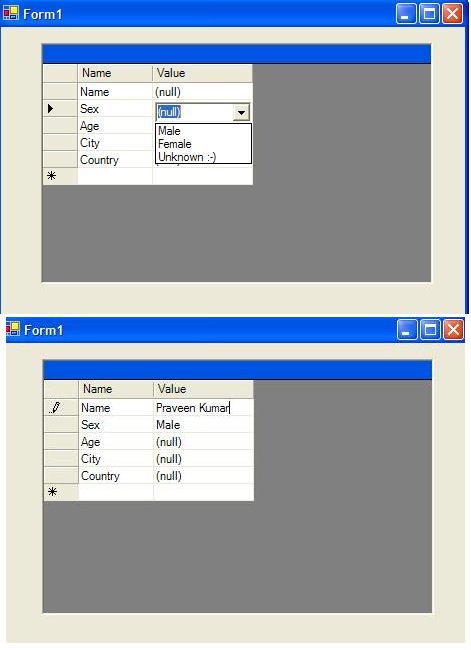
Introduction
There are times when you need to provide a user interface which is capable of taking input both in freeform (name for example) and in restricted form (combobox, e.g., Sex).
This control solves this in an easy way.
Background
I ran into one such requirement. I searched over the internet and all the articles or controls where talking about making the whole column a combobox. So I decided to tweak one
such code to take care of my need.
Using the Code
First of all, you need to add the GridColumnStyle
of the DatagridConditionalComboboxColumn
type in the table style of the DataGrid
. The constructor
of DatagridConditionalComboboxColumn
takes a ComboValueChanged
delegate as an input. This delegate is called whenever the user does
SelectedIndex
changes for the combobox.
Then you need to listen to the StatedEditing
event which is raised by the DatagridConditionalComboboxColumn
whenever a user enters the cell for editing.
Here you can check for the conditions, and based on it, you can set the ShowCombobox
property to either true
or false
.
If you set it to true
, you can the add items to the combobox.
Once you do all this... you are done. Look at the attached source code of the example.
DataGridTableStyle tableStyle = new DataGridTableStyle();
DataGridTextBoxColumn nameColumn = new DataGridTextBoxColumn();
nameColumn.MappingName = "Name";
nameColumn.HeaderText = "Name";
nameColumn.ReadOnly = true;
tableStyle.GridColumnStyles.Add(nameColumn);
ComboValueChanged comboValueChangedDelegate =
new ComboValueChanged(comboValueChanged);
DatagridConditionalComboboxColumn valueColumn =
new DatagridConditionalComboboxColumn(comboValueChangedDelegate);
valueColumn.StartedEditing +=new EventHandler(valueColumn_StartedEditing);
valueColumn.MappingName = "Value";
valueColumn.HeaderText = "Value";
tableStyle.GridColumnStyles.Add(valueColumn);
this.dataGrid1.DataSource = populateDatatable();
this.dataGrid1.TableStyles.Clear();
this.dataGrid1.TableStyles.Add(tableStyle);
Points of Interest
None..