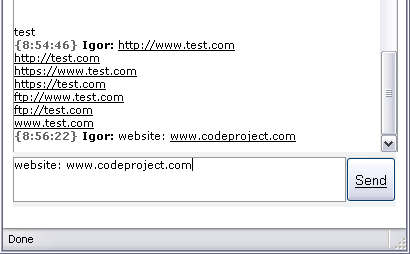
Introduction
This article describes how to replace a URL in JavaScript/AJAX chat applications so users can click on links instead of making them copy the URL from the chat into their browser address bar.
For example, when someone sends http://www.mywebpage.com in chat, you want to be able to click on that link. So this JavaScript function uses regex to replace http://www.mywebpage.com with <a href="http://www.mywebpage.com" class="my_link" target="_blank">http://www.mywebpage.com</a>.
Using the code
You just have to copy my JavaScript function called "chat_string_create_urls
" to your *.js file or in your script
tags in your HTML page and call it before displaying the messages in chat.
function chat_string_create_urls(input)
{
return input
.replace(/(ftp|http|https|file):\/\/[\S]+(\b|$)/gim,
'<a href="$&" class="my_link" target="_blank">$&</a>')
.replace(/([^\/])(www[\S]+(\b|$))/gim,
'$1<a href="http://$2" class="my_link" target="_blank">$2</a>');
}
If your messages come with '<br>
' instead of the '\n
' string then you need to use this function:
function chat_string_create_urls(input)
{
return input
.replace(/<br>/gim, '\n')
.replace(/(ftp|http|https|file):\/\/[\S]+(\b|$)/gim,
'<a href="$&" class="my_link" target="_blank">$&</a>')
.replace(/([^\/])(www[\S]+(\b|$))/gim,
'$1<a href="http://$2" class="my_link" target="_blank">$2</a>')
.replace(/\n/gim, '<br>');
}
The first replace
function replaces all the URLs that have specified a protocol, the second replace
function replaces all the URLs that begins with "www" and assumes that the protocol is HTTP.
It recognizes FTP, HTTP, HTTPS, and file protocols in links; if you don't want to limit the protocols to just those mentioned, you can set the first replace
like this:
.replace(/(\w+):\/\/[\S]+(\b|$)/gim,
'<a href="$&" class="my_link" target="_blank">$&</a>')
and now it will match every protocol.
I tested this in Firefox 2.0.0.3, Opera 9.02, and IE7.0, works OK in all of them. If you want to test it yourself, put these text in your chat app:
- http://www.test.com
- http://test.com
- https://www.test.com
- https://test.com
- ftp://www.test.com
- ftp://test.com
- www.test.com