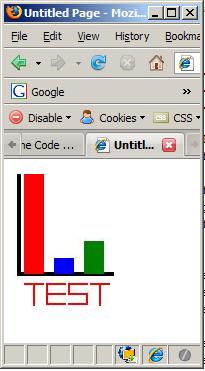
Introduction
This will show how to create graphics on the fly which can be used for reports, verification, etc.
Using the code
The code is very simple, and it uses:
System.Drawing
System.Drawing.Imaging
Here is how it looks like:
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Drawing;
using System.Drawing.Imaging;
public partial class _Default : System.Web.UI.Page
{
protected void Submit_Click(object sender, EventArgs e)
{
Bitmap oBitmap = new Bitmap(100, 150);
Graphics oGraphic = Graphics.FromImage(oBitmap);
SolidBrush rBrush = new SolidBrush(Color.Red);
SolidBrush bBrush = new SolidBrush(Color.Blue);
SolidBrush gBrush = new SolidBrush(Color.Green);
SolidBrush wBrush = new SolidBrush(Color.White);
Pen bPen = new Pen(Color.Black, 4);
Pen rPen = new Pen(Color.Red, 2);
oGraphic.FillRectangle(wBrush, 0, 0, 100, 150);
oGraphic.DrawLine(bPen, new Point(3, 100), new Point(100, 100));
oGraphic.DrawLine(bPen, new Point(5, 0), new Point(5, 100));
Render_T(10, 110, rPen, oGraphic);
Render_E(32, 110, rPen, oGraphic);
Render_S(54, 110, rPen, oGraphic);
Render_T(76, 110, rPen, oGraphic);
float fHighestValue;
float fHeight1;
float fHeight2;
float fHeight3;
float fValue1;
float fValue2;
float fValue3;
fValue1 = Convert.ToSingle(Request["Value1"]);
fValue2 = Convert.ToSingle(Request["Value2"]);
fValue3 = Convert.ToSingle(Request["Value3"]);
if (fValue1 > fValue2)
{fHighestValue = fValue1;}
else
{fHighestValue = fValue2;}
if (fValue3 > fHighestValue)
{fHighestValue = fValue3;}
if (fHighestValue == 0) fHighestValue = 1;
fHeight1 = (fValue1 / fHighestValue) * 100;
fHeight2 = (fValue2 / fHighestValue) * 100;
fHeight3 = (fValue3 / fHighestValue) * 100;
oGraphic.FillRectangle(rBrush, 10, 100 - fHeight1, 20, fHeight1);
oGraphic.FillRectangle(bBrush, 40, 100 - fHeight2, 20, fHeight2);
oGraphic.FillRectangle(gBrush, 70, 100 - fHeight3, 20, fHeight3);
Response.ContentType = "image/jpeg";
oBitmap.Save(Response.OutputStream, ImageFormat.Jpeg);
}
private void Render_T(int XAxis, int YAxis, Pen oPen, Graphics oGraphic)
{
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 0 + YAxis),
new Point(20 + XAxis, 0 + YAxis));
oGraphic.DrawLine(oPen, new Point(10 + XAxis, 0 + YAxis),
new Point(10 + XAxis, 22 + YAxis));
}
private void Render_E(int XAxis, int YAxis, Pen oPen, Graphics oGraphic)
{
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 0 + YAxis),
new Point(20 + XAxis, 0 + YAxis));
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 10 + YAxis),
new Point(20 + XAxis, 10 + YAxis));
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 20 + YAxis),
new Point(20 + XAxis, 20 + YAxis));
oGraphic.DrawLine(oPen, new Point(1 + XAxis, 0 + YAxis),
new Point(1 + XAxis, 20 + YAxis));
}
private void Render_S(int XAxis, int YAxis, Pen oPen, Graphics oGraphic)
{
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 0 + YAxis),
new Point(20 + XAxis, 0 + YAxis));
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 10 + YAxis),
new Point(20 + XAxis, 10 + YAxis));
oGraphic.DrawLine(oPen, new Point(0 + XAxis, 20 + YAxis),
new Point(20 + XAxis, 20 + YAxis));
oGraphic.DrawLine(oPen, new Point(1 + XAxis, 0 + YAxis),
new Point(1 + XAxis, 10 + YAxis));
oGraphic.DrawLine(oPen, new Point(19 + XAxis, 10 + YAxis),
new Point(19 + XAxis, 20 + YAxis));
}
}