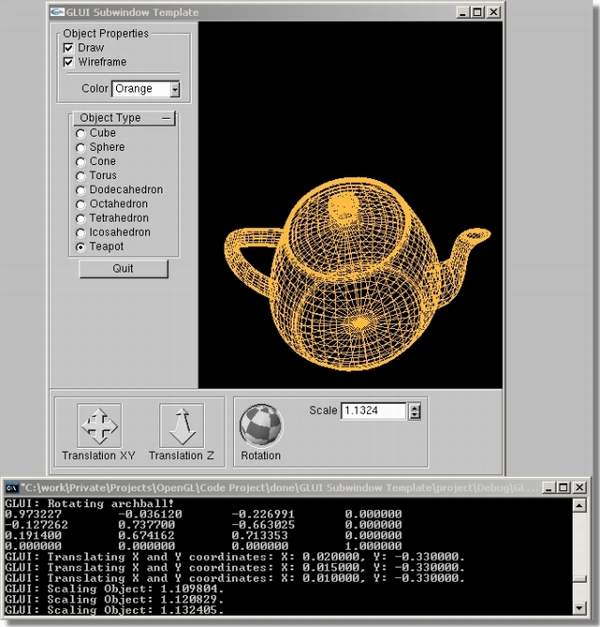
Contents
This article shows you how to create GUI controls for your OpenGL application and organize them into GLUI
subwindows. In this article, we will take the source code from the previous article GLUI Window Template, and modify it so that our GLUI
controls will be laid out inside two subwindows rather than in a single window.
This article can be used in the following ways:
- Learn how to use
GLUI
subwindows - Understand the Viewport concept in OpenGL
- Use the program as a template for your OpenGL applications that require GUI controls
If you don't know what is OpenGL, GLUT
, GLUI
, check the GLUT Window Template article first.
Having a separate window to contain our GLUI
controls could be sometimes annoying for the user, as she or he will have to lose the focus on the OpenGL context window every time she or he wants to do an action. A nice way to avoid this is to place the GLUI
controls directly into the GLUT
OpenGL window by embedding them into a GLUI
subwindow.
To avoid having to write the same code every time you want to create an OpenGL graphical application with controls inside GLUI
subwindows, this program code can be used as a template to get you directly started.
The OpenGL GLUI
subwindow template has the following properties:
- Window Title: "
GLUI
Subwindow Template" - Two
GLUI
subwindows containing GLUI
controls
- The first subwindow would contain some of the controls and will be placed on the left of the window. It will be called the vertical
GLUI
subwindow as controls will be laid out vertically inside it - The second subwindow would contain the remaining controls and will be placed on the bottom of the window. It will be called the horizontal subwindow as controls will be laid out horizontally inside it
GLUI
window where our graphics will be drawn and our two GLUI
subwindows will be placed - Handling of events for our
GLUT
window and our GLUI
controls - Showing when and where events occur and their meaning through the command prompt
For information on how to compile and run the program, please check the Usage section in the GLUI Window Template article.
The source code is intended to be used as a template for your OpenGL applications. To use it in your new application, you can simply rename the CPP file and add it to your Visual Studio project.
Please note that GLUI
is a C++ library, and thus doesn't work with C. So if you're obtaining linkage errors while trying to build a GLUI
program, just check that all file extensions are CPP and not C.
Since we have already gone through the details of creating a GLUI
window with controls and event handling in the previous GLUI Window Template article, what we care for in this article is how to create subwindows and lay out the controls inside them.
We will be replacing the window in the previous article with two subwindows placed on the left and bottom of the main graphics window.
The image below shows how our controls were laid out into a single GLUI
window in the previous article:

The image below shows how our controls will be laid out into two separate subwindows placed on the left and the bottom of our main GLUT
window:

The GLUI
subwindows are created as follows:
GLUI *glui_h_subwindow, *glui_v_subwindow;
glui_h_subwindow = GLUI_Master.create_glui_subwindow
(main_window, GLUI_SUBWINDOW_BOTTOM);
glui_v_subwindow = GLUI_Master.create_glui_subwindow
(main_window, GLUI_SUBWINDOW_LEFT);
Here is the create_glui_subwindow
function definition, as described in the GLUI manual:
GLUI *GLUI_Master_Object::create_glui_subwindow( int window, int position );
Attribute | Description |
window | ID of existing GLUT graphics window |
position | Position of new subwindow, relative to the GLUT graphics window it is embedded in. This argument can take one of the following values:
GLUI_SUBWINDOW_RIGHT
GLUI_SUBWINDOW_LEFT
GLUI_SUBWINDOW_TOP
GLUI_SUBWINDOW_BOTTOM
You can place any number of subwindows at the same relative position; in this case, multiple subwindows will simply be stacked on top of one another. For example, if two subwindows are created inside the same GLUT window, and both use GLUI_SUBWINDOW_TOP , then the two are placed at the top of the window, although the first subwindow will be above the second.
|
Fortunately, adding controls to GLUI
subwindows is exactly the same as adding controls to a GLUI
window. Thus, for example, to add the Object Properties panel to the GLUI
vertical subwindow instead of adding it to the GLUI
window, we can simply change the following code fragment:
From
|
GLUI_Panel *op_panel = glui_window->add_panel ("Object Properties");
|
To
|
GLUI_Panel *op_panel = glui_v_subwindow->add_panel ("Object Properties");
|
Thus, for all the controls that we need to place into the GLUI
vertical subwindow, we can simply replace glui_window
with glui_v_subwindow
, and for all the controls that we want to place into the GLUI
horizontal subwindow, we need to replace glui_window
with glui_h_subwindow
.
After creating our two GLUI
subwindows, we need to let each of them know where its main graphics window is:
glui_h_subwindow->set_main_gfx_window( main_window );
glui_v_subwindow->set_main_gfx_window( main_window );
When a control in a GLUI
subwindow changes value, a redisplay request will be sent to this main graphics window.
When the main window is resized, we need to change the viewport size, where our OpenGL graphics are drawn. However, this time, we can't simply set the viewport width and height to be equal to the width and height of the main window due to the GLUI
subwindows that we have added into the main window. Thus, we need to take the following into consideration:
- Viewport X Position = Width of
GLUI
subwindow placed on left - Viewport Width =
GLUI
Window Width - Width of GLUI
subwindow placed on left - Width of GLUI
subwindow placed on right - Viewport Y Position = Height of
GLUI
subwindow placed on top - Viewport Height = Height of main
GLUI
window - Height of GLUI
subwindow placed on top - Height of GLUI
subwindow placed on bottom
Thankfully, the GLUI
library supports us with a method to automatically determine the x, y, width, and height of the viewport drawing area. This would be done through the following code snippet that will be placed in the reshape function:
int vx, vy, vw, vh;
GLUI_Master.get_viewport_area( &vx, &vy, &vw, &vh );
glViewport(vx, vy, vw, vh);
The GLUI
library also supports us with a function that can even make things easier. Thus, the above code snippet can be replaced with the following code:
GLUI_Master.auto_set_viewport();
Below is the specification of each of the get_viewport_area
and auto_set_viewport
functions as they appear in the GLUI manual:
Determines the position and dimensions of the drawable area of the current window. This function is needed when GLUI
subwindows are used, since the subwindows will occupy some of the area of a window, which the graphics app should not overwrite. This function should be called within the GLUT
reshape callback function.
void GLUI_Master_Object::get_viewport_area(int *x, int *y, int *w, int *h );
Attribute | Description |
x , y , w , h | When the function returns, these variables will hold the x, y, width, and height of the drawable area of the current window. These values should then be passed into the OpenGL viewport command, glViewport() . |
Automatically sets the viewport for the current window. This single function is equivalent to the following series of commands:
int x, y, w, h;
GLUI_Master.get_viewport_area( &x, &y, &w, &h );
glViewport( x, y, w, h );
The function prototype is as follows, and it does not take any parameters:
void GLUI_Master_Object::auto_set_viewport( void );
The mouse passive motion and display events will not be logged so that we can clearly see other events on the command prompt log.
The purpose of this article is to teach you how to set up a GLUT
window with multiple GLUI
subwindows with controls inside them, along with event handling. At the same time, the template can be used to save lots of copy and paste from old projects or the Internet.
In case you find this template useful or have any suggestions, please let me know.
- 25/09/2007: Original article posted