Introduction
This is a simple web application for users who are new to ASP.NET. This will show how we can retrieve an image from a database and display it in a GridView
.
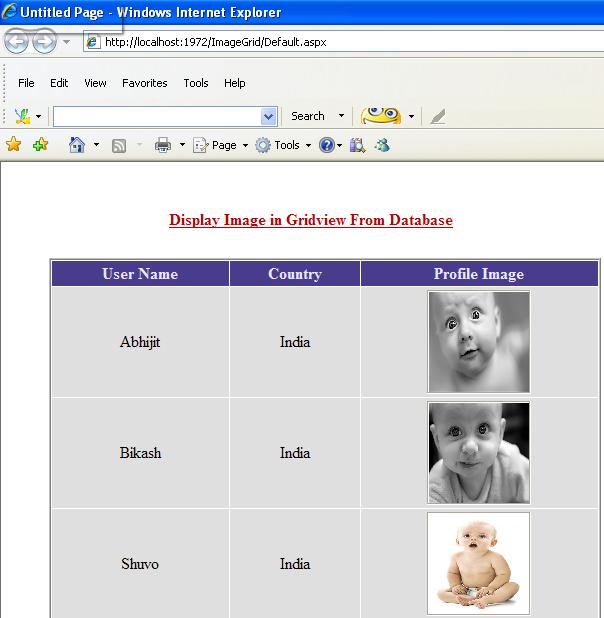
Background
Sometimes we need to upload images to a web application and store it in a database, which store images in binary format. Since that can cause a loss of image quality, we can instead store the image path in the database, and retrieve that image path from it, and display the image from that location in the web page.
In order to do this, first we need to use ADO.NET to connect to the database. The database I use here is SQL Server.

In the database shown, the Profile_Picture field contains the image path. In my case, I have stored all my images in the application directory. You may change this to any other directory, like ~\myfolder\myimage.jpg.
Now our application reads images from its current directory, so if you use a different folder from the current directory, you have to set the application's current directory by calling Directory.SetCurrentDirectory
, of the System.IO
namespace.
We also need to set some properties in the GridView
.

Perform the following actions:
- Uncheck the Auto-generate field
- Use two BoundFields for UserName and Country
- Set the Header Text and DataField (field name in database)
- Use ImageField for the image and set
DataImageUrlField = ImageFieldName
- Click OK
Using the code
The code required for the explanation above is very simple.
public partial class _Default : System.Web.UI.Page
{
SqlConnection conn = new SqlConnection();
protected void Page_Load(object sender, EventArgs e)
{
conn.ConnectionString
= "Data Source=MyServer; Integrated Security=True; database=test";
Load_GridData();
}
void Load_GridData()
{
conn.Open();
SqlDataAdapter Sqa = new SqlDataAdapter("select * from picture", conn);
DataSet ds = new DataSet();
Sqa.Fill(ds);
GridView1.DataSource = ds;
GridView1.DataBind();
conn.Close();
}
}
Points of Interest
Using images in your web application is always interesting, and after all, they are from a database!
History
First published | 7 Oct 2007 |
Edited | 10 Dec 2010 |