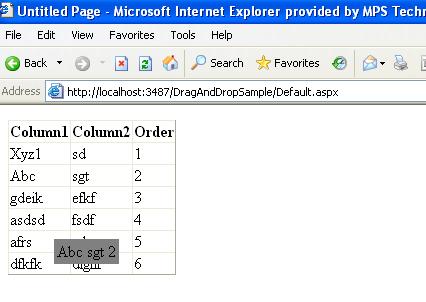

Introduction
In DragAndDropGridView
, the user can rearrange a grid item via dragging and dropping. The user can drag a gridview item and then drop it on the item where he/she wishes to swap with. This control is very helpful for large grid items where we require to order items within the grid.
Using the code
Add the control to the toolbar or add a reference to Utility.dll in your project. Then, bind with the data source which you need to order. Now, add the DragAndDropEvent
handler. DragAndDropEventArgs
gives you the start index (source row index) and the EndIndex
(destination row index). With these indices, update the data source and rebind the grid.
How this control works
This control is inherits from the GridView
so that we can benefit from the GridView
features.
HandleRow event
Handle the mouse down and mouse up events of each row in the gridview:
protected override void OnRowDataBound(GridViewRowEventArgs e)
{
base.OnRowDataBound(e);
e.Row.Attributes.Add("onmousedown", "startDragging" +
this.ClientID + "(" + e.Row.RowIndex + ",this); ");
e.Row.Attributes.Add("onmouseup", "stopDragging" +
this.ClientID + "(" + e.Row.RowIndex + "); ");
}
Whenever the user clicks on a grid item, a div
is added in the body and the innerHTML
of the div
copies the selected item. Now, set the document mouse move event for the moving item.
document.onmousemove = function ()
{
if(__item" + this.ClientID + @" != null){
__item" + this.ClientID + @".style.left=
window.event.clientX + document.body.scrollLeft +
document.documentElement.scrollLeft ;
__item" + this.ClientID + @".style.top =
window.event.clientY + document.body.scrollTop +
document.documentElement.scrollTop ;
}
}
When the user ends up on another grid item, we call stopDragging()
. This method calls the post back event.
Register the post back event script
The __doPostBack
method is not added into the page until we register the post back event on the client-side by calling the Page.ClientScript.GetPostBackEventReference(this, "")
method, which will create the __doPostback
method on the client side.
Raise the post back event
Handle the RaisePostBackEvent
of the control for raising the drag and drop events:
protected override void RaisePostBackEvent(string eventArgument)
{
base.RaisePostBackEvent(eventArgument);
if (Page.Request["__EVENTARGUMENT"] != null &&
Page.Request["__EVENTARGUMENT"] != "" &&
Page.Request["__EVENTARGUMENT"].StartsWith("GridDragging"))
{
char[] sep = { ',' };
string[] col = eventArgument.Split(sep);
DragAndDrop(this, new DragAndDropEventArgs(Convert.ToInt32(col[1]),
Convert.ToInt32(col[2])));
}
}
DragAndDropEventArgs
This EventArgs
contains the start and the end index for the dragging event:
public class DragAndDropEventArgs : EventArgs
{
private int _startIndex;
public int StartIndex
{
get { return _startIndex; }
}
private int _endIndex;
public int EndIndex
{
get { return _endIndex; }
}
public DragAndDropEventArgs(int startIndex, int endindex)
{
_startIndex = startIndex;
_endIndex = endindex;
}
}