In this small post, I am going to discuss about the new method introduced in the .NET Framework ForEach
which allows to perform the action on each set of element.
Syntax:
public void ForEach(Action<t> action)
Action<t>
is delegate or function to perform on each element of the List
.
To get into more details about how it works, check the following code.
Below is the Employee
class which has property related to employee
of the company.
public class Employee
{
public string Name { get; set; }
public int Salary { get; set; }
public string Address { get; set; }
public int Id { get; set; }
}
and now in the following line of code, I am initializing list of employee
s.
class Program
{
static void Main(string[] args)
{
List<employee> emplst = new List<employee> {
new Employee() { Name="pranay", Address = "Ahmedabad", Id=1, Salary=1234},
new Employee() { Name="Hemang", Address = "Ahmedabad", Id=2, Salary=2348},
new Employee() { Name="kurnal", Address = "Himmatnagar", Id=3, Salary=8999},
new Employee() { Name="Hanika", Address = "Ahmedabad", Id=4, Salary=7888}
};
Now I want to increase salary
of each employee by 10%, so with the help of new ForEach
construct, I can easily achieve it by using the following line of code.
emplst.ForEach(new Program().incSalary);
}
private void incSalary(Employee emp)
{
emp.Salary += (emp.Salary * 10)/100;
}
}
As you can see in the above code, I have written new Program().incSalary
as action, in the incsalary
method as you see, I increase the salary of each employee by 10%.
This thing can easily also be done by making use of the foreach
loop available but if you see the ForEach
in reflector, it does the same thing.
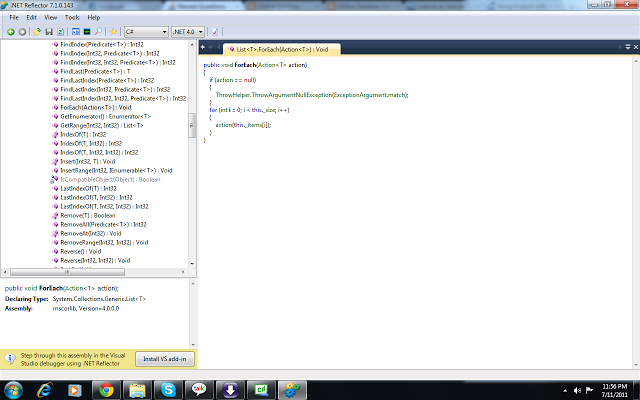
ForEach
method makes code more simple and easy to understand.
CodeProject