Introduction
Design patterns are recurring sequences, built-in .NET patterns are scattered over the framework.
In practice, following standard software patterns will help to achieve more standard code that is manageable, and understandable by a bigger set of software programmers/developers and architects.
For example, the data adapter factory that enables the creation of specific DBMS adapters. This centralized class helps in establishing a strong data layer, that supplies a more generic way to communicate with business layer.
For example:
Imports System.Data.Common
dbPFactory = DbProviderFactories.GetFactory("FactoryName")
adapter = dbPFactory.CreateDataAdapter()
UML design of generic Adapter
class that makes use of 'DbProviderFactories
' factory, and DbProviderFactory
, to create a generic layer.
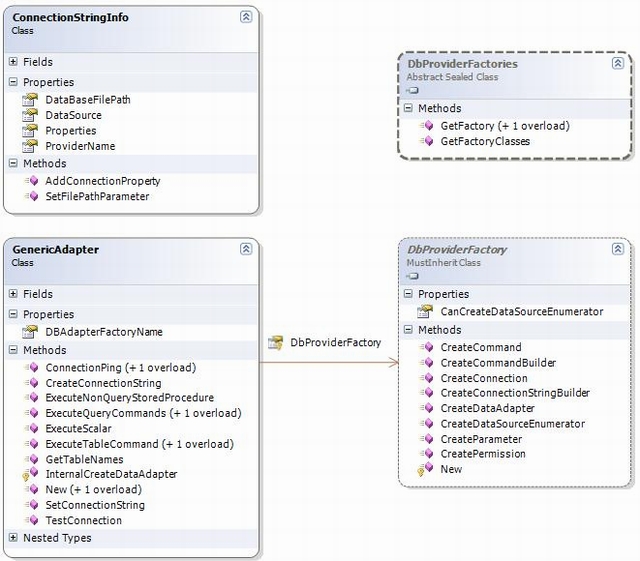
Figure 1.
In simple technical English, a factory pattern is responsible for managing and creating product class instances from a base class that has an increasing or more than one child class. The DbProviderFactories
is a factory of factories, that manages the DBMS adapters in .NET 2.0. Building a class wrapper over this architecture and supplying the correct parameters will enable the direct generation of specified data adapters to communicate with most of the standard DBMSs in the market.
The following is a code example of creating an adapter to connect to Access database:
public DataTable GetData()
{
GenericAdapter.GenericAdapter genericAdapter = null;
ConnectionStringInfo conInfo = new ConnectionStringInfo();
DataTable table = null;
conInfo.DataBaseFilePath = @"c:\db.mdb";
conInfo.ProviderName = "Microsoft.Jet.OLEDB.4.0";
conInfo.DataSource = "{FilePath}";
genericAdapter = new GenericAdapter.GenericAdapter("System.Data.OleDb");
if (genericAdapter.SetConnectionString(conInfo))
{
table = genericAdapter.ExecuteTableCommand("MyTable");
}
return table;
}
Singleton pattern is implemented in many places in the .NET Framework, single instance window applications. We also deal with singleton classes in remoting.
A singleton pattern can be achieved by forbidding the creation of direct class instance, using the constructors. This is done by making constructors private
, and preventing the user from creating an instance of the class.
This enables only the creation of instances from class scope. To enable single instance in memory, we use static
or shared reference to the class, we make it private
to prevent public
access, and we set it to NULL
.
We add a static
or shared function that checks if the class static
reference is NULL
. If NULL
an instance is created, otherwise the previous instance is returned.
The .NET Framework makes good use of the proxy pattern that is a mediator between the real object and the client object. When applying proxy design pattern, the proxy class provides the same services as the real object. That is, they inherit from the same base class, or apply the same interface. Proxy classes are used in remoting and when communicating with services.
Such patterns define the road map for extending a framework. Using these patterns will allow your applications to benefit a great deal, in extendability and code maintenance.
This was an introduction to .NET design patterns. An example of using DBFactory
to create a generic adapter can be downloaded from this page.