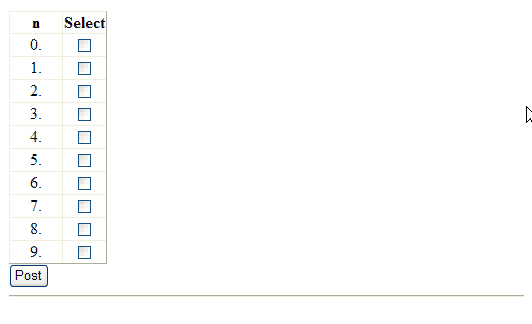
Introduction
I am presenting here a JavaScript code that ensures that at least one checkbox is checked among the checkboxes in a particular column inside a GridView
control before submitting a form.
Using the code
I have used a TemplateField
inside the GridView
and put a CheckBox
in the ItemTemplate
of the TemplateField
.
The HTML code looks like this:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:BoundField HeaderText="n" DataField="sno">
<HeaderStyle HorizontalAlign="Center" VerticalAlign="Middle" Width="50px" />
<ItemStyle HorizontalAlign="Center" VerticalAlign="Middle" />
</asp:BoundField>
<asp:TemplateField HeaderText="Select">
<ItemTemplate>
<asp:CheckBox ID="chkBxSelect" runat="server" />
</ItemTemplate>
<HeaderStyle HorizontalAlign="Center" VerticalAlign="Middle" />
<ItemStyle HorizontalAlign="Center" VerticalAlign="Middle" />
</asp:TemplateField>
</Columns>
</asp:GridView>
<asp:Button ID="btnPost" runat="server" Text="Post"
OnClientClick="javascript:return TestCheckBox();"
OnClick="btnPost_Click" />
Attach a OnClientClick
event to the button [btnPost
]:
<asp:Button ID="btnPost" runat="server" Text="Post"
OnClientClick="javascript:return TestCheckBox();"
OnClick="btnPost_Click" />
Add this JavaScript in the page’s head
section:
<script type="text/javascript">
var TargetBaseControl = null;
window.onload = function()
{
try
{
TargetBaseControl =
document.getElementById('<%= this.GridView1.ClientID %>');
}
catch(err)
{
TargetBaseControl = null;
}
}
function TestCheckBox()
{
if(TargetBaseControl == null) return false;
var TargetChildControl = "chkBxSelect";
var Inputs = TargetBaseControl.getElementsByTagName("input");
for(var n = 0; n < Inputs.length; ++n)
if(Inputs[n].type == 'checkbox' &&
Inputs[n].id.indexOf(TargetChildControl,0) >= 0 &&
Inputs[n].checked)
return true;
alert('Select at least one checkbox!');
return false;
}
</script>
In the above script, the global variable TargetBaseControl
has been initialized in the window.onload
event in order to get the reference of the parent control [GridView
]. The function TestCheckBox
first gets the array of all ‘input’
elements inside the parent control. After that, it iterates all the elements of the array and tests a condition, and if the condition is found to be true, it returns true
indicating that at least one checkbox is checked inside the GridView
; else it displays a message and returns false
. The condition tests the elements of a specific type in a particular column inside the parent element.
Just download the sample application and enjoy coding! I hope you will like this article.
Features of this script
This script is cross-browser compatible and fast as it iterates elements of a specific tag inside a target element [GridView
] rather than iterating in a whole form. It searches the elements of a specific type in a particular column of the target element [GridView
].
Supporting browsers
I have tested this script on the following browsers:
