Introduction
Its a chat application using the TCP/IP sockets. To get this application running you need to add the control for the communication through the etharnet.
Background
This was the simple application created for the seminar at college level. Hope will be helpful for the beginners in the communication programming
Using the code
There are couple of section that need to be coded before launching the application. We need to create two forms One will act as a server and other will be acting as a client. Where we can manupulate as a client server chat application
Now let see the coding of the Server Side Form
There are couple of events to be handled during the programming.
Private Sub Form_Load()
On Error GoTo err
tcpServer.LocalPort = 1001
tcpServer.Listen
Exit Sub
err:
MsgBox Error
End Sub
Private Sub Command1_Click()
On Error GoTo err
If txtSendData.Text <> "" Then
tcpServer.SendData txtSendData.Text
main.AddItem (tcpServer.LocalHostName + " (You) : " + txtSendData.Text)
Beep
txtSendData.Text = ""
End If
Exit Sub
err:
MsgBox("No Connection", vbInformation)
End Sub
Private Sub Command2_Click()
Dim a
a = StrReverse(txtSendData.Text)
On Error GoTo err
If txtSendData.Text <> "" Then
tcpServer.SendData(a)
main.AddItem(tcpServer.LocalHostName + " (You) : " + Trim(a))
Beep
txtSendData.Text = ""
End If
Exit Sub
err:
MsgBox("No Connection", vbInformation)
End Sub
Private Sub tcpServer_ConnectionRequest (ByVal requestID As Long)
On Error GoTo err
If tcpServer.State <> sckClosed Then _
tcpServer.Close
tcpServer.Accept requestID
Exit Sub
err:
MsgBox Error
End Sub
Private Sub tcpServer_DataArrival (ByVal bytesTotal As Long)
On Error GoTo err
Dim strData As String
tcpServer.GetData strData
txtOutput.Text = strData
main.AddItem (tcpServer.RemoteHostIP + " (Friend) : " + txtOutput.Text)
Beep
Exit Sub
err:
MsgBox Error
End Sub
Now let see the coding of the Client Side Form
Private Sub Form_Load()
On Error GoTo err
cmdDisconnect.Enabled = False
tcpClient.RemotePort = 1001
Exit Sub
err:
MsgBox Error
End Sub
Private Sub cmdConnect_Click()
On Error GoTo err
tcpClient.RemoteHost = Trim(ip.Text)
cmdDisconnect.Caption = "Disconnect To " & ip.Text
tcpClient.Connect
cmdDisconnect.Enabled = True
cmdConnect.Enabled = False
Exit Sub
err:
MsgBox Error
End Sub
Private Sub cmdDisconnect_Click()
On Error GoTo err
tcpClient.Close
cmdDisconnect.Enabled = False
main.Clear
txtSendData.Text = ""
cmdConnect.Enabled = True
Exit Sub
err:
MsgBox Error
End Sub
Private Sub Command1_Click()
On Error GoTo err
If txtSendData.Text <> "" Then
tcpClient.SendData txtSendData.Text
main.AddItem (tcpClient.LocalIP + " (You) : " + txtSendData.Text)
Beep
txtSendData.Text = ""
End If
Exit Sub
err:
MsgBox( "Connection not established", vbInformation)
End Sub
Private Sub Command2_Click()
Dim a
a = StrReverse(txtSendData.Text)
On Error GoTo err
If txtSendData.Text <> "" Then
tcpClient.SendData(a)
main.AddItem (tcpClient.LocalIP + " (You) : " + Trim(a))
Beep
txtSendData.Text = ""
End If
Exit Sub
err:
MsgBox ("Connection not established", vbInformation)
End Sub
Private Sub tcpClient_DataArrival (ByVal bytesTotal As Long)
On Error GoTo err
Dim strData As String
tcpClient.GetData strData
txtOutput.Text = strData
main.AddItem (tcpClient.RemoteHost + " (Friend) : " + txtOutput.Text)
Beep
Exit Sub
err:
MsgBox Error
End Sub
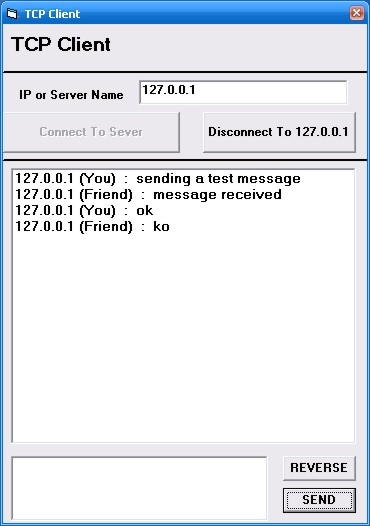
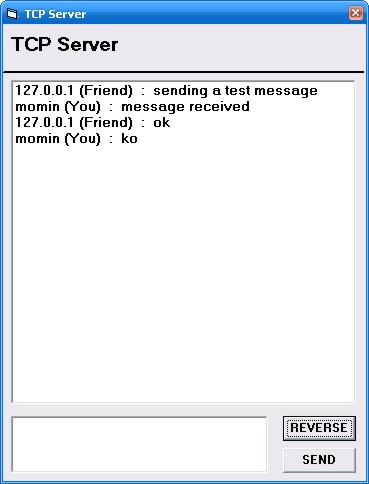