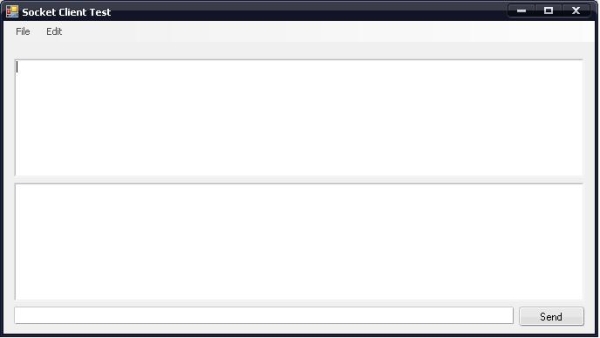
Contents
Getting and storing settings is a needed skill in programming. If you need to store and retrieve configuration sections in a generic and type safe way, then this article will be of help.
The demo application is a Windows socket client that I use for testing the socket protocol.
The common way for storing and retrieving settings is by using AppSettings
or ConnectionString
, and these are used for simple configurations.
When you need to create objects from a configuration file, then creating sections is the way to go. The class that will consume the configuration section will be derived from the ConfigurationSection
class.
Deriving from ConfigurationSection
is a simple and powerful way to use configuration sections in an object oriented way.
The application configuration will store the data and link them to their type.
class OptionsConfigHandler : ConfigurationSection
{
[ConfigurationProperty("HostName", IsRequired = true,
IsKey = false, DefaultValue = "127.0.0.1")]
public string HostName
{
get { return (string)base["HostName"]; }
set { base["HostName"] = value; }
}
[ConfigurationProperty("Port", IsRequired = true,
IsKey = false, DefaultValue = "4433")]
public string Port
{
get { return (string)base["Port"]; }
set { base["Port"] = value; }
}
}
The OptionsConfigHandler
class can be used to retrieve data from a section in the application configuration file. When this class is used to store data to the application setting, the setting will look like:
="1.0"="utf-8"
<configuration>
<configSections>
<section name="OptionSection"
type="SocketTest.OptionsConfigHandler, SocketTest, Version=1.0.0.0,
Culture=neutral, PublicKeyToken=null" allowLocation="true"
allowDefinition="Everywhere"
allowExeDefinition="MachineToApplication"
overrideModeDefault="Allow"
restartOnExternalChanges="true" requirePermission="true" />
</configSections>
<OptionSection HostName="127.0.0.1" Port="4433" />
</configuration>
public class ExeConfig
{
private static Configuration config;
public static Configuration Config
{
get
{
if (config == null)
config = ConfigurationManager.OpenExeConfiguration(
ConfigurationUserLevel.None);
return config;
}
}
public static T GetSection<T>(string sectionName) where T :
ConfigurationSection
{
T options = null;
try
{
options = Config.GetSection(sectionName) as T;
}
catch
{
}
return options;
}
public static void AddSection(string name, ConfigurationSection section)
{
config.Sections.Add(name, section);
Config.Save(ConfigurationSaveMode.Full);
}
public static void Save()
{
Config.Save(ConfigurationSaveMode.Modified);
}
}
This class reads and writes sections to the configuration file, and it is all you need to get started.
To read the OptionsConfigHandler
section from the configuration file, here is the code:
OptionsConfigHandler options = ExeConfig.GetSection<OptionsConfigHandler>(sectionName);
To write the OptionsConfigHandler
section to the configuration file, here is the code:
OptionsConfigHandler options =
ExeConfig.GetSection<OptionsConfigHandler>(sectionName);
if (options == null)
{
options = new OptionsConfigHandler();
ExeConfig.AddSection(sectionName, options);
}
ExeConfig.Save();
Voila!