Introduction
We face scenarios where the text in the drop-down list exceeds the size of the drop-down list. Many developers try to wrap the text in such scenarios, this may be helpful, however I felt that it wasn’t a great UI (User Interface). Usually it happens that if we increase the size of the drop-down list the page gets a horizontal scroll-bar.
Truncated text creates problem for the user, as (s)he may not be able to see the entire content in the drop-down and it would create a problem for (s)he when they are selecting any values which differ only on last couple of bits/characters.
The later half of the document describes adding font color and back-ground color to the drop-down list items. Usually we face a problem in ASP.Net when we need to add color to the back-ground of an item in the drop-down list, because of which many developers prefer HTML Controls as adding back-ground color is comparatively simpler with HTML Controls. We have tried to add font color and back-ground color to drop-down list using Java Script.
Using the code
Adjusting the width of the drop-down list dynamically
We would firstly add the drop-down list to the table/data-grid as usual and would then add the logic of adjusting the width.
We are using the “oncontextmenu”, this event gets fired when the user right-clicks on the control. Once the right-click event is captured, we invoke a Javascript function to increase the width of the drop-down list. If the width is initially less, then we increase it to the maximum – the one required to see all the items. If the width is currently maximum, then or right-click we need to again resize the control so that the page would look as usual.
<TABLE id="Table1" style="LEFT: 144px; POSITION: absolute; TOP: 48px;"
cellSpacing="1" cellPadding="1" width="208" border="1">
<TR>
<TD style="WIDTH: 138px"><asp:Label id="lblColour1" runat="server" BorderStyle="None">Colour Name:</asp:Label></TD>
<TD><asp:DropDownList Width="40" id="cmbColour1" runat="server" oncontextmenu="fnJumpSize (this);"></asp:DropDownList></TD>
</TR>
<TR>
<TD style="WIDTH: 138px"><asp:Label id="lblColour2"
runat="server" BorderStyle="None">Colour Name:</asp:Label></TD>
<TD><asp:DropDownList Width="40" id="cmbColour2"
runat="server" oncontextmenu="fnJumpSize(this);"></asp:DropDownList></TD>
</TR>
<TR>
<TD style="WIDTH: 138px"><asp:Label id="lblColour3"
runat="server" BorderStyle="None">Colour Name:</asp:Label></TD>
<TD><asp:DropDownList Width="40" id="cmbColour3"
runat="server" oncontextmenu="fnJumpSize(this);"></asp:DropDownList></TD>
</TR>
</TABLE>
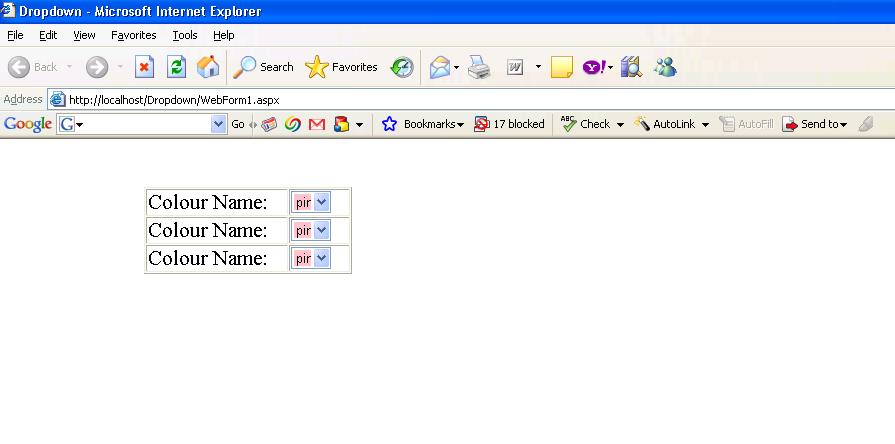
Now we would have a look at the Javascript event which is used to adjust the width of the drop-down list on right-clicking on the drop-down.
function fnJumpSize(cmbDrop)
{
if(cmbDrop.style.width != "100px")
{
cmbDrop.style.width = "100";
}
else
{
cmbDrop.style.width = "40";
}
}
On right-clicking the drop-down list box
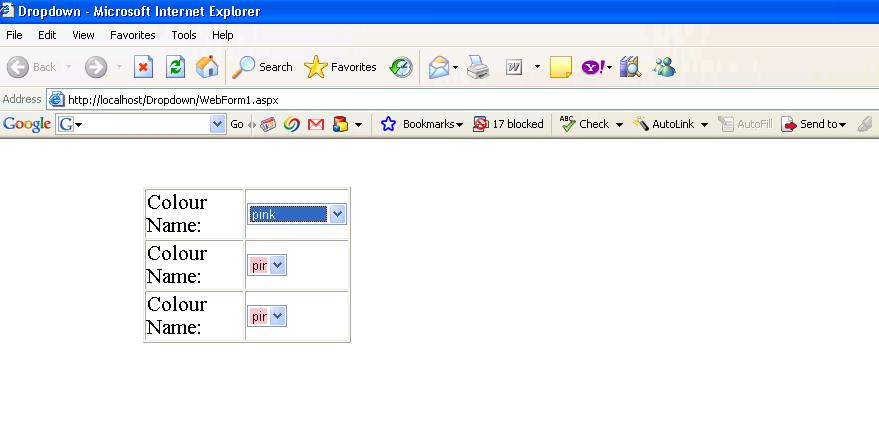
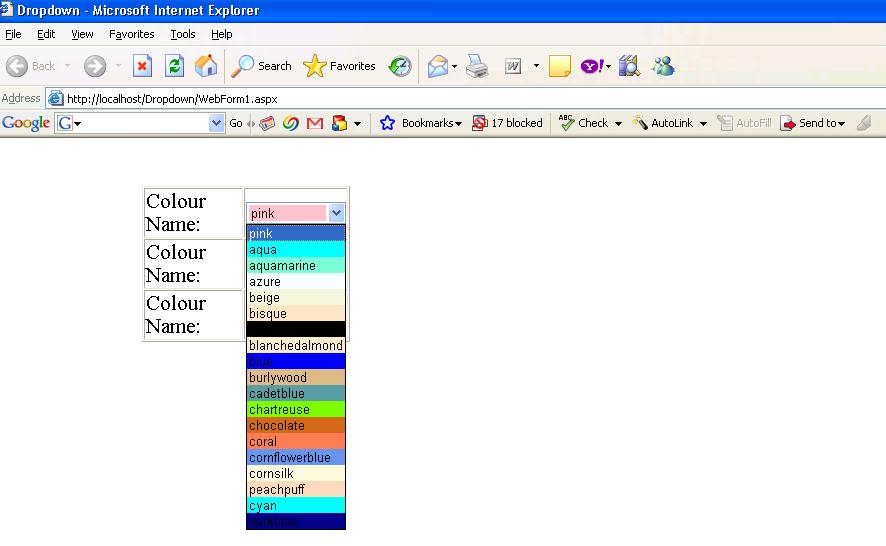
On right-clicking the drop-down list box again
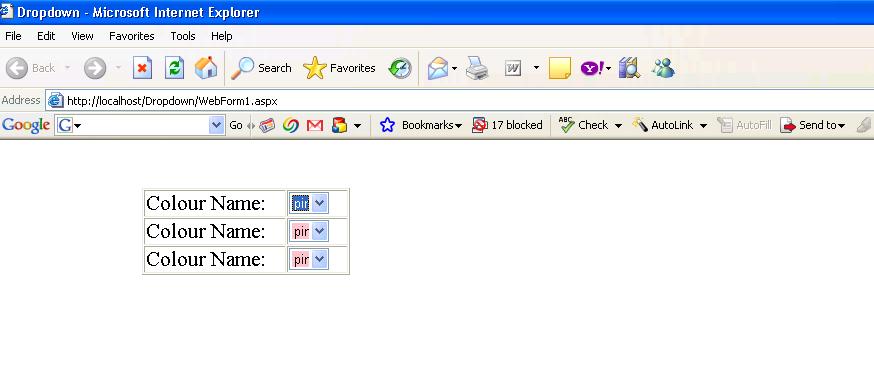
Important: Need to note the Width of the Items
This section is very important for the developers. I would suggest that during creation of the web-page, give the width for the drop-down list in such a manner that it would avoid any horizontal scroll bar and the design would be fit the screen. Later one check for the maximum width of the drop-down list items and enter that value into the javascript function fnJumpSize
.
In case you wish to incorporate this functionality across the application then just replace it with any constant and populate the value of that constant/hidden variable from the front-end.
Adding color to the back-ground and fore-ground using JavaScript
We are calling a Javascript function at page load which adds color to the list items. This function takes the back-ground colors from the string format and adds them to the individual items, in case you have multiple color scheme, I would suggest to populate it in the front-end itself.
function fnColourIt()
{
var strColors = "pink$aqua$aquamarine$azure$beige$bisque$black$blanchedalmond$
blue$burlywood$cadetblue$chartreuse$chocolate$coral$cornflowerblue$
cornsilk$peachpuff$cyan$darkblue";
var arrColors = strColors.split("$");
var startOne = 1;
while(startOne < 4)
{
var drpOne = document.getElementById("cmbColour" + startOne);
var i;
for (i = 0; i < arrColors.length; i++)
{
htmlCode = document.createElement('option');
htmlCode.text = arrColors[i];
htmlCode.value = arrColors[i];
drpOne.options.add(htmlCode);
drpOne.options[i].style.background = drpOne.options[i].value;
}
startOne = parseInt(startOne) + 1;
}
}
The first three lines in the for loop are creating a list item and assigning it text and value respectively. Fourth line runs the command to add the list item to the drop-down list.
The following line adds the back-ground color for the list item:
drpOne.options[i].style.background = drpOne.options[i].value;
The following line adds the fore-ground color for the list item:
drpOne.options[i].style.color = "white";
We can make any other modifications like changing the font-family, size or making any particular text as bold et al in a similar manner.
Conculsion:
1) User can increase the size/width of any drop-down list dynamically
2) Back-ground coloring for the drop-down list in ASP.Net is time consuming and difficult, which is handled in a fairly easy manner in Javascript
3) Simple to implement
4) Scalable, i.e. by making small changes to the JS function, you can scale it across the application