Introduction
In the article Configurable Aspects for MEF, I introduced a configurable AOP model
for MEF based on the Dynamic Decorator.
The same model can be applied to other IoC containers like Unity
or Windsor, etc.
In this article, I describe the AOP model for the Unity IoC container. Using this model, you configure aspects for objects in the application configuration
file and use a customized Unity container to bring the objects in life with the aspects attached to them.
Background
The Dynamic Decorator is a tool for extending functionality of objects by attaching behaviors to them instead of by modifying their classes or creating new classes.
It is attractive because it saves you from design or redesign of your classes. Please read the articles Dynamic
Decorator Pattern and Add Aspects to Object Using Dynamic Decorator to understand more
about the Dynamic Decorator, what problems it tries to solve, how it solves the problems, and its limits.
There are several other articles that discuss how the Dynamic Decorator is used for application development and compare it with other similar technologies.
Please refer to the References section of this article for them.
Once you go over some of the articles, you will see that the Dynamic Decorator is simple to use and powerful. In this article, I describe a configurable model
of the Dynamic Decorator, which makes its use even easier and still as powerful. This model is then integrated with the Unity container so that an object coming out
from the Unity container has aspects attached already.
Using this model, you configure aspects for your objects in application configuration files. Then, a customized Unity container brings your objects in life
and wires them with the aspects based on the configurations. By the time you use the objects, the functionality defined by aspects has already attached to the objects.
Example
Say, you have a simple component Employee
that implements two interfaces IEmployee
and INotifyPropertyChanged
as follows.
public interface IEmployee
{
System.Int32? EmployeeID { get; set; }
System.String FirstName { get; set; }
System.String LastName { get; set; }
System.DateTime DateOfBirth { get; set; }
System.Int32? DepartmentID { get; set; }
System.String DetailsByLevel(int iLevel);
}
public interface INotifyPropertyChanged
{
event PropertyChangedEventHandler PropertyChanged;
}
public class Employee : IEmployee, INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void NotifyPropertyChanged(String info)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(info));
}
}
#region Properties
public System.Int32? EmployeeID { get; set; }
public System.String FirstName { get; set; }
public System.String LastName { get; set; }
public System.DateTime DateOfBirth { get; set; }
public System.Int32? DepartmentID { get; set; }
#endregion
public Employee(
System.Int32? employeeid
, System.String firstname
, System.String lastname
, System.DateTime bDay
, System.Int32? departmentID
)
{
this.EmployeeID = employeeid;
this.FirstName = firstname;
this.LastName = lastname;
this.DateOfBirth = bDay;
this.DepartmentID = departmentID;
}
public Employee() { }
public System.String DetailsByLevel(int iLevel)
{
System.String s = "";
switch (iLevel)
{
case 1:
s = "Name:" + FirstName + " " + LastName + ".";
break;
case 2:
s = "Name: " + FirstName + " " + LastName +
", Birth Day: " + DateOfBirth.ToShortDateString() + ".";
break;
case 3:
s = "Name: " + FirstName + " " + LastName +
", Birth Day: " + DateOfBirth.ToShortDateString() +
", Department:" + DepartmentID.ToString() + ".";
break;
default:
break;
}
return s;
}
}
Define Aspects
Aspects are cross-cutting concerns. For Dynamic Decorator, an aspect is a method that takes an AspectContext
type object as its first parameter and
an object[]
type object as its second parameter and returns void
.
You may design your aspects in a more general way to use them in various situations. You may also design your aspects for some particular situations.
For example, you can define your entering/exiting log aspects in a general way and put them in the class SysConcerns
as follows.
public class SysConcerns
{
static SysConcerns()
{
ConcernsContainer.runtimeAspects.Add(
"DynamicDecoratorAOP.SysConcerns.EnterLog",
new Decoration(SysConcerns.EnterLog, null));
ConcernsContainer.runtimeAspects.Add(
"DynamicDecoratorAOP.SysConcerns.ExitLog",
new Decoration(SysConcerns.ExitLog, null));
}
public static void EnterLog(AspectContext ctx, object[] parameters)
{
StackTrace st = new StackTrace(new StackFrame(4, true));
Console.Write(st.ToString());
IMethodCallMessage method = ctx.CallCtx;
string str = "Entering " + ctx.Target.GetType().ToString() +
"." + method.MethodName + "(";
int i = 0;
foreach (object o in method.Args)
{
if (i > 0)
str = str + ", ";
str = str + o.ToString();
}
str = str + ")";
Console.WriteLine(str);
Console.Out.Flush();
}
public static void ExitLog(AspectContext ctx, object[] parameters)
{
IMethodCallMessage method = ctx.CallCtx;
string str = "Exiting " + ctx.Target.GetType().ToString() +
"." + method.MethodName + "(";
int i = 0;
foreach (object o in method.Args)
{
if (i > 0)
str = str + ", ";
str = str + o.ToString();
}
str = str + ")";
Console.WriteLine(str);
Console.Out.Flush();
}
}
As you can see, these methods access Target
and CallCtx
in a general way and can be shared by various types of objects to write entering/exiting logs.
On the other hand, some aspects may need to access more specific information. For example, you want to attach a change notification functionality only
to an Employee
object when its property is set. The following code defines some specific aspects.
class LocalConcerns
{
static LocalConcerns()
{
ConcernsContainer.runtimeAspects.Add(
"ConsoleUtil.LocalConcerns.NotifyChange",
new Decoration(LocalConcerns.NotifyChange, null));
ConcernsContainer.runtimeAspects.Add(
"ConsoleUtil.LocalConcerns.SecurityCheck",
new Decoration(LocalConcerns.SecurityCheck, null));
}
public static void NotifyChange(AspectContext ctx, object[] parameters)
{
((Employee)ctx.Target).NotifyPropertyChanged(ctx.CallCtx.MethodName);
}
public static void SecurityCheck(AspectContext ctx, object[] parameters)
{
Exception exInner = null;
try
{
if (parameters != null && parameters[0] is WindowsPrincipal &&
((WindowsPrincipal)parameters[0]).IsInRole(
"BUILTIN\\" + "Administrators"))
{
return;
}
}
catch ( Exception ex)
{
exInner = ex;
}
throw new Exception("No right to call!", exInner);
}
}
In the above code, the NotifyChange
method can only be used by a target of Employee
while the SecurityCheck
requires
a WindowsPrincipal
object as a parameter.
Note: You may already have noticed that there is a static constructor in each of the classes SysConcerns
and LocalConcerns
.
Inside the static classes, each of aspect methods defined in the classes is used to create an instance of Decoration
which is then added
to a dictionary ConcernsContainer.runtimeAspects
. The definition of ConcernsContainer
is as follows.
public class ConcernsContainer
{
static public Dictionary<string, Decoration>
runtimeAspects = new Dictionary<string, Decoration>();
}
The purpose of this Dictionary
and the static constructors is to keep an inventory for all Decoration
objects based on the aspect methods defined
in the application and make them accessible by the corresponding method names. It makes possible to configure aspects in the application configuration file
by specifying the corresponding method names.
Configure Aspects
In the configuration file, you specify how aspects are associated with objects. Here are some examples to demonstrate how the aspects are configured.
<configuration>
<configSections>
<section name="DynamicDecoratorAspect"
type="DynamicDecoratorAOP.Configuration.DynamicDecoratorSection,
DynamicDecoratorAOP.Configuration" />
</configSections>
<DynamicDecoratorAspect>
<objectTemplates>
<add name="1"
type="ThirdPartyHR.Employee"
interface="ThirdPartyHR.IEmployee"
methods="DetailsByLevel,get_EmployeeID"
predecoration="SharedLib.SysConcerns.EnterLog,SharedLib.SysConcerns"
postdecoration=""/>
<add name="2"
type="ThirdPartyHR.Employee"
interface="ThirdPartyHR.IEmployee"
methods="set_EmployeeID"
predecoration="ConsoleUtil.LocalConcerns.SecurityCheck"
postdecoration="ConsoleUtil.LocalConcerns.NotifyChange"/>
</objectTemplates>
</DynamicDecoratorAspect>
</configuration>
First of all, you need to add a section <DynamicDecoratorAspect>
in your configuration file. Then, inside <objectTemplates>
of <DynamicDecoratorAspect>
, you add individual elements. For each element inside <objectTemplates>
, the following attributes need to be specified:
type
- target typeinterface
- interface returnedmethods
- names of target methods which will be attached to the aspects specified by predecoration
and postdecoration
predecoration
- preprocessing aspectpostdecoration
- postprocessing aspect
Notes:
- The names in the value of the
methods
attribute are comma separated. For example, "DetailsByLevel,get_EmployeeID"
. - The value of the
predecoration
attribute has two parts and is separated by a comma. The first part specifies the aspect name while the second part
specifies the assembly name in which the aspect is defined, for example, "SharedLib.SysConcerns.EnterLog,SharedLib.SysConcerns"
.
If the second part is not specified, it is assumed that the aspect is defined in the entry assembly, for example, "ConsoleUtil.LocalConcerns.SecurityCheck"
. - The value of the
postdecoration
attribute has two parts and is separated by a comma. The first part specifies the aspect name while the second
part specifies the assembly name in which the aspect is defined. If the second part is not specified, it is assumed that the aspect is defined in the entry assembly.
Use AOPUnityContainer
The AOPUnityContainer
class is responsible for reading in aspect configurations, constructing target objects, and wiring aspects to target objects.
The following code demonstrates how it is used.
class Program
{
static void Main(string[] args)
{
using (IUnityContainer container = new UnityContainer())
{
container.RegisterType<IEmployee, Employee>(new InjectionConstructor());
AOPUnityContainer aopcontainer = new AOPUnityContainer(container);
IEmployee emp = aopcontainer.Resolve<Employee, IEmployee>();
emp.EmployeeID = 1;
emp.FirstName = "John";
emp.LastName = "Smith";
emp.DateOfBirth = new DateTime(1990, 4, 1);
emp.DepartmentID = 1;
emp.DetailsByLevel(2);
Employee target = null;
IEmployee emp1 = aopcontainer.Resolve<Employee,
IEmployee>("set_EmployeeID");
try
{
Thread.GetDomain().SetPrincipalPolicy(PrincipalPolicy.WindowsPrincipal);
Decoration dec = ConcernsContainer.runtimeAspects[
"ConsoleUtil.LocalConcerns.SecurityCheck"];
dec.Parameters = new object[] { Thread.CurrentPrincipal };
target = aopcontainer.GetTarget<Employee>();
target.PropertyChanged +=
new PropertyChangedEventHandler(PropertyChanged_Listener);
emp1.EmployeeID = 2;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
Console.ReadLine();
}
static void PropertyChanged_Listener(object sender, PropertyChangedEventArgs e)
{
Console.WriteLine(e.PropertyName.ToString() + " has changed.");
}
}
You create an object container
of UnityContainer
and use it as usual to register types, etc. Then, instead of using it to resolve to a business
object, you create an aopcontainer
of AOPUnityContainer
by passing the container
object into its constructor. At this point, you can use
Resolve<T, V>()
by specifying the target type T
and the returned interface type V
. For example, in the above code, you specify
the Employee
as the target type and IEmployee
as the returned interface type. It returns a proxy of IEmployee
type. That's it. Now, when using emp
, it starts writing the entering log.
Resolve<T, V>()
finds the first matched element in the configuration file by looking for the type
attribute as T
and interface
attribute as V
, and then, uses the element settings to create a proxy. For Resolve<Employee, IEmployee>()
,
it tries to find the type
attribute as "ThirdPartyHR.Employee"
and interface
attribute as "ThirdPartyHR.IEmployee"
in the configuration file. The first element in the configuration is matched. Therefore, the value "DetailsByLevel,get_EmployeeID"
of the methods
attribute, the value "SharedLib.SysConcerns.EnterLog,SharedLib.SysConcerns"
of the predecoration
attribute, and the value ""
of the postdecoration
attribute are used to create a proxy. When using emp
, only the methods DetailsByLevel
and get_EmployeeID
will write the entering log.
Resolve<T, V>(string methods)
finds the first matched element in the configuration file by looking for the type
attribute as T
,
interface
attribute as V
, and methods
attribute as methods
. For example,
the code Resolve<Employee, IEmployee>("set_EmployeeID")
tries to match the type
attribute as "ThirdPartyHR.Employee"
,
the interface
attribute as "ThirdPartyHR.IEmployee"
, and the methods
attribute as "set_EmployeeID"
,
and the second element in the configuration is matched. Therefore, the value "ConsoleUtil.LocalConcerns.SecurityCheck"
of the predecoration
attribute and the value "ConsoleUtil.LocalConcerns.NotifyChange"
of the postdecoration
attribute are used to create a proxy. When using
emp1
, only the method set_EmployeeID
will check the security before its invocation and raise a notification after its invocation.
There are a few more points worth noting. Before the emp1
invokes the aspect LocalConcerns.SecurityCheck
, its paramters
argument
needs to be updated to a WindowsPrincipal
object. It can be achieved by getting the Decoration
object associated with the aspect using
the Dictionary
of the ConcernsContainer
and then setting its Parameters
property. The first three lines of the above code
in the try
block does this.
In order to capture the event raised from the aspect LocalConcerns.NotifyChange
after the method set_EmployeeID
sets the property,
you need to register a listener to the target object of Employee
before you call the method set_EmployeeID
. To achieve this,
you use the GetTarget<T>()
method of AOPUnityContainer
to get the target object, then register the listener to the target object.
The last three lines of code in the try
block does this.
When executing the program, you see the following output.
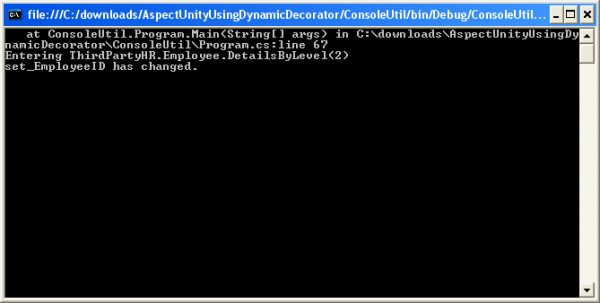
If you comment out the line Thread.GetDomain().SetPrincipalPolicy(PrincipalPolicy.WindowsPrincipal)
, you see the following output.

Summary
Configurable aspects combined with the Unity container make AOP very simple. You define your aspect methods and then add elements in the application configuration
file to associate your business objects with the aspects. Most of the time, that is all you need to do. In some special cases, you can still update the parameters argument
of an aspect and get the target object using code.
References
The following articles will help you understand Dynamic Decorator and its features.
- Dynamic Decorator Pattern
- Add Aspects to Object Using Dynamic Decorator
The following articles discuss how the Dynamic Decorator can help you improve your application development.
- Components, Aspects, and Dynamic Decorator
- Components, Aspects, and Dynamic Decorator for ASP.NET Application
- Components, Aspects, and Dynamic Decorator for ASP.NET MVC Application
- Components, Aspects, and Dynamic Decorator for Silverlight / WCF Service Application
- Components, Aspects, and Dynamic Decorator for MVC/AJAX/REST Application
The following articles compare the Dynamic Decorator with some other similar tools.
- Dynamic Decorator and Castle DynamicProxy Comparison
- Dynamic Decorator, Unity, and Castle DynamicProxy Comparison
The following articles discuss some miscellaneous topics regarding Dynamic Decorator and AOP.
- Performance of Dynamic Decorator
- Generic Dynamic Decorator
- Aspects to Object vs. Aspects to Class