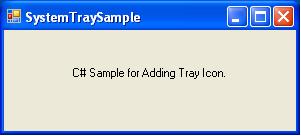
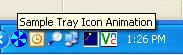
Introduction
This article explains how to animate the tray icon. In .NET, they have simplified the things to add an icon in the system tray.
While writing an application, in many cases we need to add the application to the system tray and most of the common functionality will be provided from the context menu from the system tray. The application will show background processing through an icon animation, like if the application is processing an event, then it will animate the icon till the event processing is complete.
Background
If you are writing an application in C#, it has the NotifyIcon()
component with capabilities to add an application icon in the system tray, or you can use the Shell API Shell_NotifyIcon()
to add the icon; using the API is not very easy though.
About the SystemTray Class
This class wraps almost all the functionalities of the NotifyIcon
component as well as extends the functionality to achieve easy animation of the tray icon. The class provides functions with which it is easy to configure the class.
SystemTray Class Summary
It is very simple to use this class. I will describe how to use the class after explaining all the other details of the class.
Constructors
Syntax
This class provides four constructors to simplify the use of the class in your project.
SystemTray()
SystemTray(string pTrayText)
SystemTray(string pTrayText, Icon pIcon)
SystemTray(string pTrayText,Icon pIcon,bool pAnimate)
Parameter details
pTrayText
: A string you want to display as the tooltip text when the mouse points at the application system tray icon.pIcon
: A System.Drawing.Icon
object which will be displayed in the system tray.pAnimate
: A bool
parameter to support the animation capabilities.
Methods
Syntax
void StartAnimation()
void StopAnimation()
void StartAnimation(int timeOut)
void ShowBaloonTrayTip(int nTimeout)
void ShowBaloonTrayTip(int nTimeOut,string tipTitle,
string tipText,ToolTipIcon tipIcon)
void SetIconRange(object[] IconList)
Properties
Icon Icon
bool Visible
string TrayText
bool Animate
string BaloonTipText
string BalloonTipTitle
ToolTipIcon BalloonTipIcon
Using the SystemTray Class
Here is how to use the SystemTray
class:
object[] objValues = new object[2];
objValues[0] = Convert.ToString("Icon1.ico");
objValues[1] = Convert.ToString("Icon2.ico");
stray= new SystemTray("Sample Tray Icon Animation",null,true);
stray.SetIconRange(objValues);
stray.SetContextMenuOrStrip((object)contextMenuStrip1);
stray.DoubleClick += new System.EventHandler(this.DbClick);
stray.ContextMenuStrip.Items[0].Click +=
new EventHandler(SystemTraySample_Click);
stray.StartAnimation(2000);