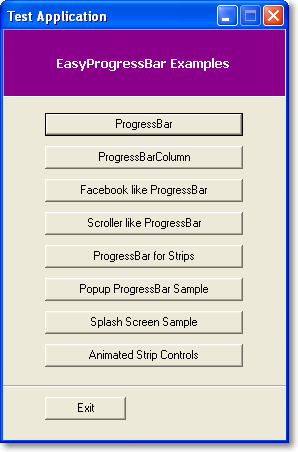
Welcome screen
The library packet includes the following controls and components:
FloatWindowAlphaMaker | Component |
AniEasyProgressTaskManager | Component |
AnimatedEasyProgressControl | Control |
EasyProgressBarSplashForm | Form |
EasyProgressBar | Control |
and includes three derived controls:
ToolStripEasyProgressBarItem | derives from the ToolStripControlHost class |
ToolStripAnimatedEasyProgressBarItem | derives from the ToolStripControlHost class |
EasyProgressBarColumn | derives from the DataGridViewColumn class |
Introduction
This library has a few progressbar examples for strip controls, DataGridView columns, and a Windows Forms application with clone support.
- RGBA changer
- Docking support
- Supports digital number style [7 segment]
- Supports dynamic properties for end-users
- Provides keyboard support to the user for progressbar operations
For more details, please look at my EasyProgressBar for Windows Forms Application article.
Background

EasyProgressBar sample
We need to create specific classes, properties, methods, and a progress event for our controls. To do this, I have implemented custom classes and defined an interface and its
name is IProgressBar
. EasyProgressBar
and ToolStripEasyProgressBarItem
implement this interface.
public interface IProgressBar
{
GradientHover HoverGradient { get; set; }
GradientProgress ProgressGradient { get; set; }
GradientBackground BackgroundGradient { get; set; }
ColorizerProgress ProgressColorizer { get; set; }
GradientDigitBox DigitBoxGradient { get; set; }
int Value { get; set; }
int Minimum { get; set; }
int Maximum { get; set; }
bool IsPaintBorder { get; set; }
bool IsDigitDrawEnabled { get; set; }
bool ShowPercentage { get; set; }
string DisplayFormat { get; set; }
Color BorderColor { get; set; }
EasyProgressBarBorderStyle ControlBorderStyle { get; set; }
event EventHandler ValueChanged;
}
Shortcut Keys
If IsUserInteraction
property is enabled, provides keyboard support to the user for EasyProgressBar
operations.
Keyboard Keys
Keys | Description |
End | Changes to maximum value. |
Home | Changes to minimum value. |
Left | Decrease the current value on the control. |
Right | Increase the current value on the control. |
Return/Enter | It changes our control's docking mode. |
F1 | Not implemented. |
EasyProgressBarColumn

EasyProgressBarColumn sample
To create a progressbar column for DataGridView
controls, you must first create a class that derives from the DataGridViewColumn
class.
You can then override the CellTemplate
property in this class. Here's the class declaration:
public class EasyProgressBarColumn : DataGridViewColumn
{
#region Constructor
public EasyProgressBarColumn()
: base(new EasyProgressBarCell())
{
}
#endregion
#region Property
public override DataGridViewCell CellTemplate
{
get { return base.CellTemplate; }
set
{
if (value != null &&
!value.GetType().IsAssignableFrom(typeof(EasyProgressBarCell)))
{
throw new InvalidCastException(
"The cell template must be an EasyProgressBarCell.");
}
base.CellTemplate = value;
}
}
#endregion
}
To use the percentage format or change the display format for your columns, you need to use the CellStyle Builder dialog.

CellStyle builder dialog
EasyProgressBar for strip controls
To create a progressbar for strip controls, you must first create a class that derives from the ToolStripControlHost
class.
The following code snippet shows how you can create a custom progressbar for all strip controls.
[ToolboxBitmap(typeof(System.Windows.Forms.ProgressBar))]
[ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.All)]
public class ToolStripEasyProgressBarItem : ToolStripControlHost, IProgressBar, ICloneable
{
}

ToolStripEasyProgressBarItem sample
Animated progress control for strip controls
The following code snippet shows how you can create a custom animated progress control for all strip controls.
[ToolboxBitmap(typeof(System.Windows.Forms.ProgressBar))]
[ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.All)]
public class ToolStripAnimatedEasyProgressBarItem : ToolStripControlHost, ICloneable
{
}

ToolStripAnimatedEasyProgressBarItem samples
AniEasyProgressTaskManager
We use this component to apply animation support for a progressbar image. The important properties for the component are listed in the below table.
Property | Description |
FPS | Gets or sets the frame per second value of the specified image. |
AnimatedGif | Gets or sets the image to be animated. |
FrameCount | Gets the total number of frames of the specified image. |
CurrentFrame | Gets the index of the active frame. |
IsReverseEnabled | Determines whether the animated GIF should play backwards when it reaches the end of the frame. |
IsWorking | If the task monitor is running, return true, otherwise false. |
CancelCheckInterval | How often the thread checks to see if a cancellation message has been heeded (a number of seconds). |
CancelWaitTime | How long the thread will wait (in total) before aborting a thread that hasn't responded to the cancellation message. TimeSpan.Zero means polite stops are not enabled. |
Properties from the AniEasyProgressTaskManager component
How can I use this component?
To use this tool for your AnimatedEasyProgressControl
or EasyProgressBarSplashForm
, firstly, you need to add
a new AniEasyProgressTaskManager
component to your design form and connect it with your animated control or splash form. And then,
you can call the Start(Control invokeContext)
and StopTask()
methods of the component.
private void btnGetData_Click(object sender, EventArgs e)
{
aniEasyProgressTaskManager1.Start(animatedEasyProgressControl1);
aniEasyProgressTaskManager1.StopTask();
}
You can also customize your progress bitmap while the task is running, or handle a new image when the index of the active frame changes. To do this,
you can use FrameChanged
event of the animated control or splash form.

Popup sample
Clone support
I've created an attribute to apply clone support to a specific property. This way, we can copy control properties that are important to us.
After that, we need to link this attribute to the appropriate property. The following code snippet shows how you can add this attribute to your appropriate property declaration:
[Description("Gets or Sets, the maximum progress value of the control")]
[DefaultValue(100)]
[Browsable(true)]
[Category("Progress")]
[IsCloneable(true)]
public int Maximum { get; set; }
You can do the same behavior for other properties. To clone a control, we call the below code in our method:
public object Clone()
{
EasyProgressBar progressBar =
CustomControlsLogic.GetMyClone(this) as EasyProgressBar;
progressBar.Font = this.Font;
progressBar.ForeColor = this.ForeColor;
return progressBar;
}
Here's the clone method and the details for control cloning:
public static Object GetMyClone(Object source)
{
Type sourceType = source.GetType();
object newObject = Activator.CreateInstance(sourceType, false);
foreach (System.Reflection.PropertyInfo pi in sourceType.GetProperties())
{
if (pi.CanWrite)
{
IsCloneableAttribute attribute = GetAttributeForSpecifiedProperty(pi);
if (attribute == null)
continue;
if (attribute.IsCloneable)
{
Type ICloneType = pi.PropertyType.GetInterface("ICloneable", true);
if (ICloneType != null)
{
ICloneable IClone = (ICloneable)pi.GetValue(source, null);
if (IClone != null)
{
pi.SetValue(newObject, IClone.Clone(), null);
}
else
{
pi.SetValue(newObject, null, null);
}
}
else
{
pi.SetValue(newObject, pi.GetValue(source, null), null);
}
}
}
}
return newObject;
}
History
- November 18, 2011 - Updated
- Created a new animated progress control for all strip controls and added a new example for this.
- Fixed
NullReferenceException
- November 14, 2011 - Updated
- Added splashscreen example
- Fixed
ObjectDisposedException
- November 11, 2011 - First release