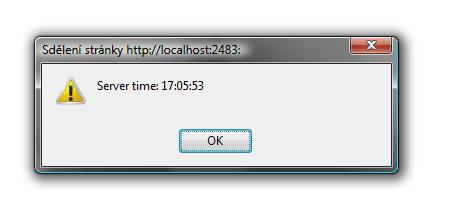
Introduction
This article is intended for anyone who is looking for an easy-to-use AJAX web control.
Background
The actual control consist of two parts: a server-side WebControl which handles AJAX requests from the client, and on the other side, a client-side JavaScript object which handles the responses from the server.
Using the code
This control was intended to be as easy to use as it can be, and so it is.
Server-side code
protected void Page_Load(object sender, EventArgs e)
{
AJAX aj = new AJAX("myAJAX"
, "AJAXCallbackHandler",
this.AJAXCommandEventHandler,
this.AJAXErrorOccuredEventHandler);
this.Controls.Add(aj);
}
private void AJAXCommandEventHandler(object sender, AJAXCommandEventArgs e)
{
e.Response = "Response from the server";
}
private void AJAXErrorOccuredEventHandler(object sender, AJAXErrorEventArgs e)
{
Response.Close();
Response.Write("Error occured in AJAX control - message: " + e.Message);
Response.End();
}
When the page loads, the server control will generate a JavaScript into the head section of the page. This script contains the object that you can use to send requests to the server.
Client-side code
<script type="text/javascript">
function SendToServer(message)
{
myAJAX.SendCommand("message");
myAJAX.SendCommand("message", 10000);
}
function AJAXCallbackHandler(resp)
{
alert("Request was: " + resp.Request +
" and response from the server is " + resp.Response);
}
</script>
Points of interest
Note - if you send many callbacks in a short period of time, the inner JavaScript object will send the first one, and store the others in a buffer. When the response returns, or a timeout occurs, it sends the next callback from the buffer, and so on, until all callbacks are sent. This functionality makes possible that responses from the server will come in the same order as requests were sent.
But, even with this functionality, I recommend you to group those callbacks into a bigger one rather than send many in a sequence. On the other side, if your callbacks are large, then the first option is better.
I've also done some fixes, including sending bad characters ('<', '>', '=', '?', '&', ....) in a callback. Now, you can send anything you like, it should work just fine. If it doesn't, please let me know!
And that's just it. Now, you can easily use AJAX callbacks in your application. The control was tested and worked fine under IE7, IE8, Firefox v3.0, and Opera v9.51 browsers. Please let me know if you experience any difficulties while using the control. And, I will also like to hear some improvements suggestions.