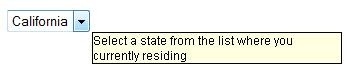
Introduction
IE for Windows does not support the Tooltip
property or even the Title
attribute on the <select>
control. This is because they create
a separate window for the <select>
tag that is above the rest of the info on the browser window. (Think of it as another layer.) The tooltip engine is actually
on the main window layer, so the tooltip would appear behind the <select>
element if it was to appear. This layered behavior also breaks popups that position over
<select>
tags because they are always on the main window layer!
Background
All other elements support tooptip, but dropdown does not. I tried to add this support using simple JavaScript.
Using the code
In order to support tooltip for a dropdown, you need to include the dropdown.js file in your HTML or ASP file.
<head>
<script type="text/javascript" src="dropdown.js"></script>
</head>
Then just declare an iFrame
object named ptoolTip
under the body
tag of the document. We need an iFrame
object
to make sure that elements under the tooltip doesn't bleed through.
<BODY><iframe id="ptoolTip" ondrop="return false;"
style="Z-INDEX: 101;LEFT: -210px; POSITION: absolute; TOP: 40px;"
frameBorder="0" scrolling="no">
</iframe></BODY>
Then just assign the onmouseover
and onmouseout
events to your SELECT
tag:
<BODY>
<iframe id="ptoolTip" ondrop="return false;"
style="Z-INDEX: 101;LEFT: -210px; POSITION: absolute; TOP: 40px;"
frameBorder="0" scrolling="no">
</iframe>
<form>
<select name="State"
onmouseover="return showTip(this,'Select a state from the list where you currently residing')"
onmouseout="return hideText()"></select>
<form>
</BODY>
Now if you move the mouse over the dropdown, the browser will show "Select state..." as a tooltip for the dropdown. If you leave the field or after a certain time,
it will hide the tooltip. The showTip
function first calculates the left and top position to place the iFrame
, that is the bottom right corner of the dropdown.
If the parent of the SELECT
object is a DIV
or SPAN
, then you need to calculate the offset left and top based on the parent
hierarchy. Basically, you need to add the parent's offset left and offset top to get the exact absolute positions. We then create a DIV
element and assign the tooltip to that.
All the styles are part of the DIV
object, but it can be put inside a CSS file. It assumes a 10Px font and calculates the default width; this may not be required as we reassign
the iFrame
's width and height with the DIV
's width and height, so that the iFrame
's dimensions
are enough to display the tooltip. Finally, it sets a timer so that it will automatically closes the tooltip. This can al be parameterized so that it is configurable based on the requirement.
Points of interest
IE 7 stores the selected item's display value in the title
attribute and it may show our custom tip and selected tip also; in order to override that,
we may have to cancel the event. Instead of calling showTip
directly, you may need to assign show("")
for the onmouseover
event.
function show(tip){
ele = event.srcElement;
if(tip != null && tip.length > 0) {
hideText();
ele.title="";
showTip(ele,tip);
event.cancelBubble= true;
return false;
}
}
<BODY>
<iframe id="ptoolTip" ondrop="return false;"
style="Z-INDEX: 101;LEFT: -210px; POSITION: absolute; TOP: 40px;"
frameBorder="0" scrolling="no">
</iframe>
<form>
<select name="State"
onmouseover="return show('Select a state from the list " +
"where you currently residing')"
onmouseout="return hideText()"></select>
<form>
</BODY>