Introduction
This custom UI Actions for SharePoint extends the lists action menu to allow users to zip document library items and download all of them with or without version.
Features
- Download all document library items
- Versions: if you are care about document versions, you can download them as well
- Ability to download only the selected view items instead of all list items
The new menu added to the SharePoint document library Actions menu:
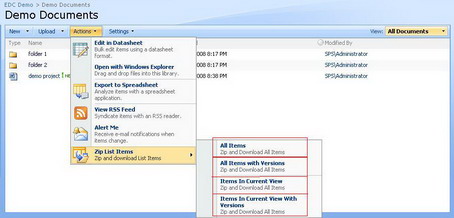
After clicking over the menu item:

the compressed file is downloaded:

The compressed file downloaded with the file versions:

Code description
First, we need to create a new feature that defines a new SharePoint Custom UI Action as below:
="1.0"="utf-8"
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction
Id="DownloadZippedItems.MenuLink"
Location="Microsoft.SharePoint.StandardMenu"
GroupId="ActionsMenu"
ControlAssembly="MZaki.CustomActions.DownloadZippedItems,
Version=1.0.0.0, Culture=neutral, PublicKeyToken=da6289be64eaeba3"
ControlClass="MZaki.CustomActions.DownloadZippedItems">
</CustomAction>
</Elements>
In this custom action, we use a Control Assembly instead of redirecting to another URL. This assembly is responsible for rendering the menu and its sub menus and handling the postback event:
protected override void CreateChildControls()
{
if (!this.ChildControlsCreated)
{
base.CreateChildControls();
SubMenuTemplate mnuZipListItems = new SubMenuTemplate();
mnuZipListItems.Text = "Zip List Items";
mnuZipListItems.ImageUrl = "/_layouts/images/TBSPRSHT.GIF";
mnuZipListItems.Description = "Zip and download List Items";
PostBackMenuItemTemplate mnuListItem =
new PostBackMenuItemTemplate();
mnuListItem.ID = "menu1";
mnuListItem.Text = "All Items";
mnuListItem.Description = "Zip and Download All Items";
mnuListItem.OnPostBack +=
new EventHandler<eventargs>(mnuListItem_OnPostBack);
PostBackMenuItemTemplate mnuListItem2 = new PostBackMenuItemTemplate();
mnuListItem2.Text = "All Items with Versions";
mnuListItem2.Description = "Zip and Download All Items";
mnuListItem2.ID = "menu2";
mnuListItem2.OnPostBack +=
new EventHandler<eventargs>(mnuListItem2_OnPostBack);
MenuSeparatorTemplate separator = new MenuSeparatorTemplate();
PostBackMenuItemTemplate mnuListItemCurrentView =
new PostBackMenuItemTemplate();
mnuListItemCurrentView.Text = "Items In Current View";
mnuListItemCurrentView.Description = "Zip and Download All Items";
mnuListItemCurrentView.ID = "menu3";
mnuListItemCurrentView.OnPostBack +=
new EventHandler<eventargs>(mnuListItemCurrentView_OnPostBack);
PostBackMenuItemTemplate mnuListItemCurrentViewVersions =
new PostBackMenuItemTemplate();
mnuListItemCurrentViewVersions.Text =
"Items In Current View With Versions";
mnuListItemCurrentViewVersions.Description =
"Zip and Download All Items";
mnuListItemCurrentViewVersions.ID = "menu4";
mnuListItemCurrentViewVersions.OnPostBack +=
new EventHandler<eventargs>(mnuListItemCurrentViewVersions_OnPostBack);
mnuZipListItems.Controls.Add(mnuListItem);
mnuZipListItems.Controls.Add(mnuListItem2);
mnuZipListItems.Controls.Add(separator);
mnuZipListItems.Controls.Add(mnuListItemCurrentView);
mnuZipListItems.Controls.Add(mnuListItemCurrentViewVersions);
this.Controls.Add(mnuZipListItems);
}
}
Now, in the postback handler, we simply retrieve the list item files and compress them to a temp directory, and force the browser to download the file.
void PushFileToDownload(string FilePath,string FileName)
{
FileInfo fInfo = new FileInfo(FilePath);
HttpContext.Current.Response.ContentType = "application/x-download";
HttpContext.Current.Response.AppendHeader("Content-Disposition",
"attachment; filename=" + FileName);
HttpContext.Current.Response.AddHeader("Content-Length", fInfo.Length.ToString());
HttpContext.Current.Response.WriteFile(FilePath);
HttpContext.Current.Response.Flush();
HttpContext.Current.Response.End();
}
Here is the project page on CodePlex: http://codeplex.com/mzakicustomactions.
Known issues
- Arabic file names: Arabic file names are not downloaded correctly
- The tool uses the system temporary directory to export the list items and compress them, so you need to watch this folder and manage a cleanup mechanism
Thank you
- The compression library I've used to compress the list items is SharpZipLib.
- I have also used a code sample posted on Peter Bromberg's blog to compress the entire folder. Thank you Peter :) The post and the code sample were great.